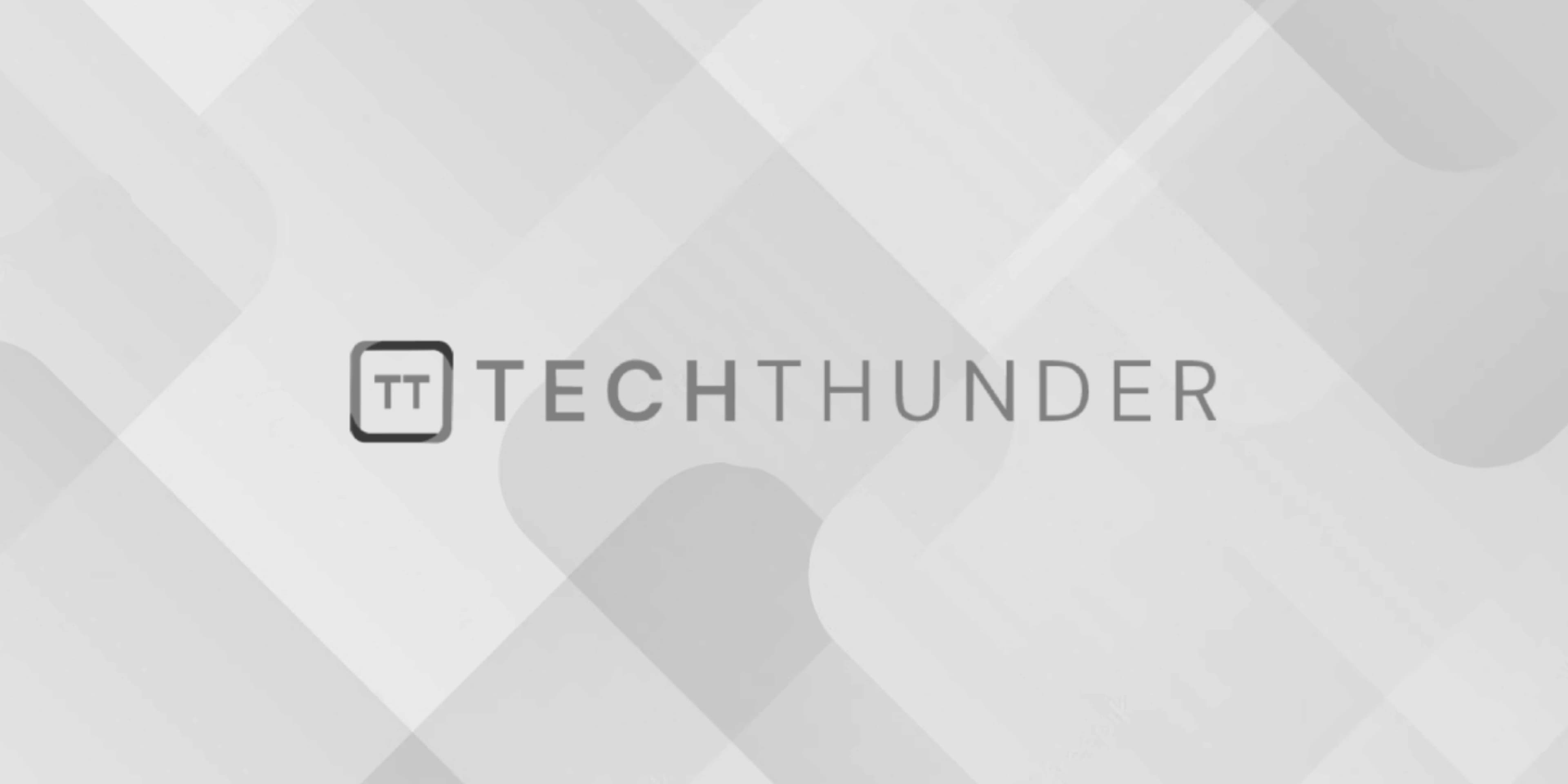
138 views
Python Find in List
The Python can use various methods to find elements in a list based on specific criteria. Here are some common approaches to find elements in a list:
- Using a Loop:
- You can use a
for
loop to iterate through the elements of a list and check each element against your criteria. This is a basic method to find elements.
Python
numbers = [1, 2, 3, 4, 5]
target = 3
found = False
for num in numbers:
if num == target:
found = True
break
if found:
print(f"{target} found in the list.")
else:
print(f"{target} not found in the list.")
- Using the
in
Operator:
- You can use the
in
operator to check if an element exists in a list.
Python
numbers = [1, 2, 3, 4, 5]
target = 3
if target in numbers:
print(f"{target} found in the list.")
else:
print(f"{target} not found in the list.")
- Using List Comprehension:
- You can use list comprehension to create a new list containing elements that match a specific condition.
Python
numbers = [1, 2, 3, 4, 5]
target = 3
found_numbers = [num for num in numbers if num == target]
if found_numbers:
print(f"{target} found in the list.")
else:
print(f"{target} not found in the list.")
- Using the
index()
Method:
- The
index()
method returns the index of the first occurrence of an element in a list.
Python
numbers = [1, 2, 3, 4, 5]
target = 3
try:
index = numbers.index(target)
print(f"{target} found at index {index}.")
except ValueError:
print(f"{target} not found in the list.")
- Using the
filter()
Function:
- The
filter()
function can be used to filter elements from a list based on a given function or condition.
Python
numbers = [1, 2, 3, 4, 5]
target = 3
found_numbers = list(filter(lambda x: x == target, numbers))
if found_numbers:
print(f"{target} found in the list.")
else:
print(f"{target} not found in the list.")
Choose the method that best fits your specific requirements and coding style when searching for elements in a list. The choice may depend on factors such as performance, readability, and the complexity of your search criteria.