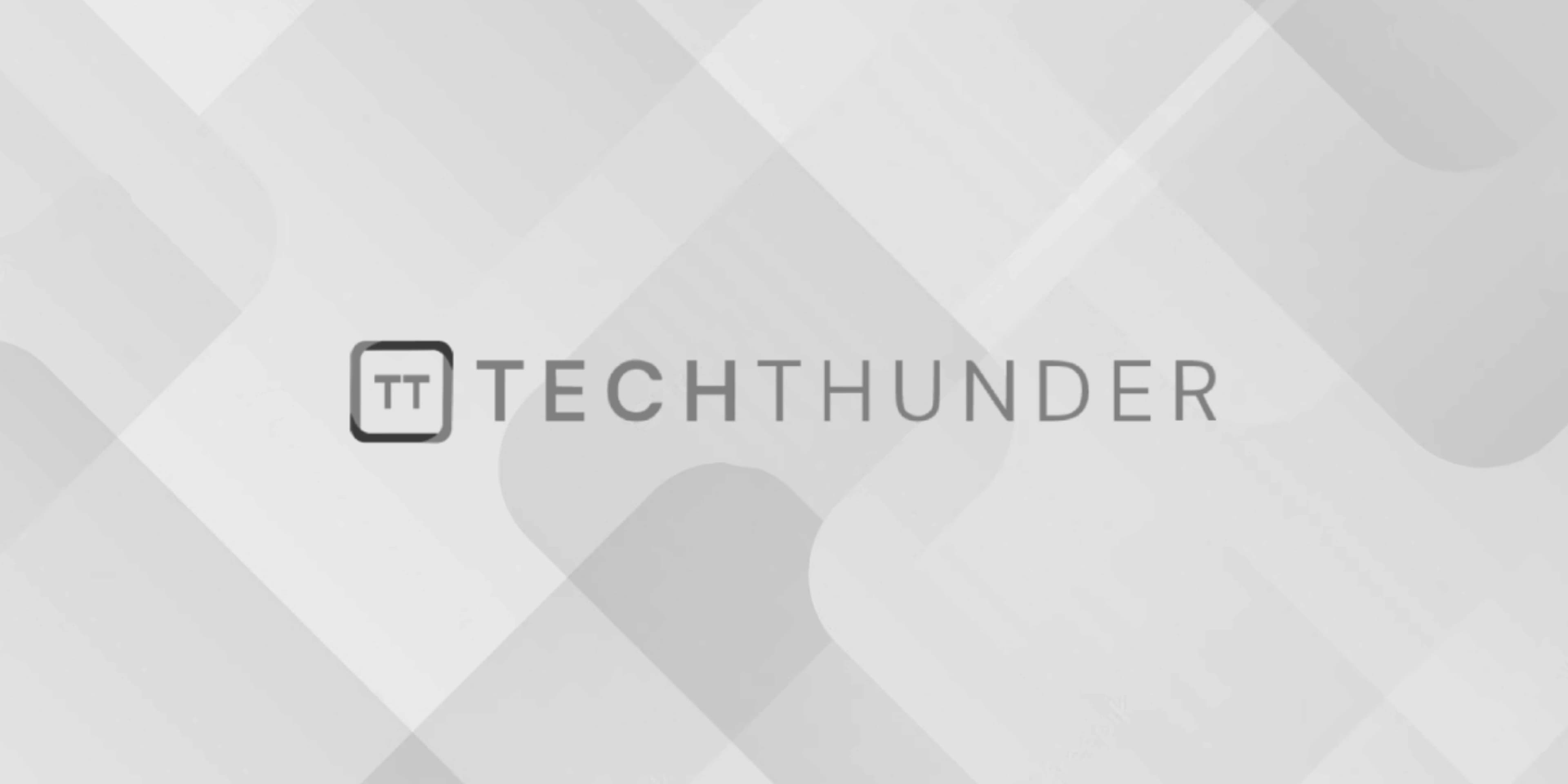
Python Asynchronous Programming – asyncio and await
Asynchronous programming in Python using asyncio
and await
allows you to write concurrent and non-blocking code, making it easier to work with I/O-bound or high-concurrency tasks. asyncio
is a Python library that provides an event loop and other asynchronous programming tools. The await
keyword is used to pause the execution of a coroutine until a particular asynchronous operation is complete.
Here’s a brief introduction to asynchronous programming with asyncio
and await
:
- Defining Coroutines: In asynchronous programming, functions are typically defined as asynchronous coroutines using the
async
keyword. Coroutines are functions that can be paused and resumed.
import asyncio
async def my_coroutine():
print("Start Coroutine")
await asyncio.sleep(2)
print("Coroutine resumed after 2 seconds")
- The Event Loop:
asyncio
relies on an event loop to manage and coordinate the execution of asynchronous tasks. You can create and run an event loop usingasyncio.run()
:
asyncio.run(my_coroutine())
The event loop manages the execution of asynchronous tasks, scheduling and running them concurrently.
- Using
await
: Inside a coroutine, you can use theawait
keyword to pause the execution of the coroutine until a specific asynchronous operation is complete. In the example above,await asyncio.sleep(2)
pauses the coroutine for 2 seconds. - Asynchronous Functions: You can await other asynchronous functions and coroutines within a coroutine. For example, you might use
await
with I/O-bound operations like file reading, network requests, or database queries. - Concurrency: You can run multiple coroutines concurrently using
asyncio.gather()
or other concurrency primitives provided byasyncio
. This allows you to perform tasks concurrently without blocking the event loop.
async def main():
coro1 = my_coroutine()
coro2 = my_coroutine()
await asyncio.gather(coro1, coro2)
- Error Handling: Proper error handling is essential in asynchronous code. You can use
try...except
blocks and handle exceptions raised by asynchronous operations. - Non-Blocking I/O: Asynchronous code allows you to perform I/O operations without blocking the event loop, making it suitable for network communication, web scraping, and other I/O-bound tasks.
- Concurrency vs. Parallelism: Asynchronous programming is not the same as parallelism. It enables concurrency, which means multiple tasks can be executed in overlapping time periods on a single thread. If you need true parallelism, you might consider using the
concurrent.futures
module or multiprocessing.
Asynchronous programming can be particularly beneficial for building scalable network applications, web servers, and performing I/O-bound operations efficiently. However, it requires a good understanding of how asyncio
works and how to structure your code to take full advantage of asynchronous capabilities while handling potential complexities like race conditions and deadlocks.