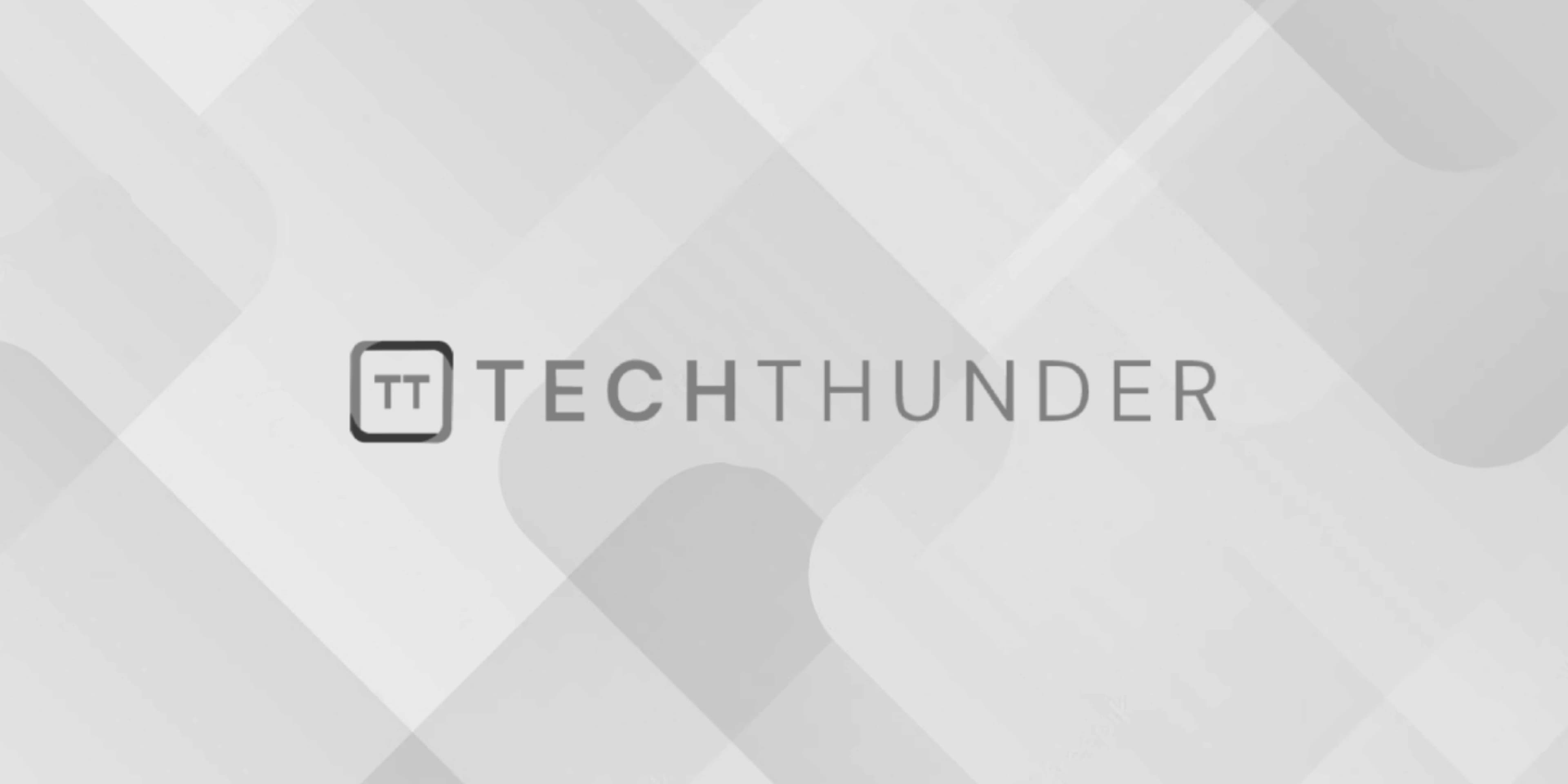
Python Tkinter PanedWindow
The Tkinter PanedWindow
widget provides a container for arranging and managing a set of horizontal or vertical panes. Each pane can contain other widgets, and users can adjust the size of panes by dragging the divider between them. This is useful for creating resizable user interfaces with flexible layouts. Here’s an example of how to use the PanedWindow
widget:
import tkinter as tk
from tkinter import PanedWindow
root = tk.Tk()
root.title("PanedWindow Example")
# Create a horizontal PanedWindow
paned_window = PanedWindow(root, orient="horizontal")
paned_window.pack(fill="both", expand=True)
# Create two frames to place inside the PanedWindow
frame1 = tk.Frame(paned_window, background="red")
frame2 = tk.Frame(paned_window, background="blue")
# Add the frames to the PanedWindow
paned_window.add(frame1)
paned_window.add(frame2)
# Configure the weight of the panes
paned_window.paneconfig(frame1, weight=1)
paned_window.paneconfig(frame2, weight=2)
root.mainloop()
In this example:
- Import the necessary modules from
tkinter
. - Create the main application window using
tk.Tk()
. - Create a
PanedWindow
widget usingPanedWindow(root, orient="horizontal")
. Theorient
parameter specifies the orientation of the panes, which can be"horizontal"
or"vertical"
. - Pack the
PanedWindow
widget using.pack()
withfill="both"
andexpand=True
to make it resize with the window. - Create two
Frame
widgets to place inside thePanedWindow
. These frames will act as the panes within the paned window. - Add the frames to the
PanedWindow
using.add()
. - Configure the weight of the panes using
.paneconfig()
. The weight determines how the available space is distributed among the panes. In this example, the second pane will receive twice as much space as the first one. - Start the main event loop using
root.mainloop()
.
When you run this code, you’ll see a horizontal PanedWindow
with two resizable panes: one with a red background and another with a blue background. You can drag the divider between the panes to adjust their sizes.
Remember that the PanedWindow
allows you to create more complex layouts with multiple nested panes and widgets within them. You can customize the appearance and behavior of the panes, dividers, and other aspects to suit your application’s needs.