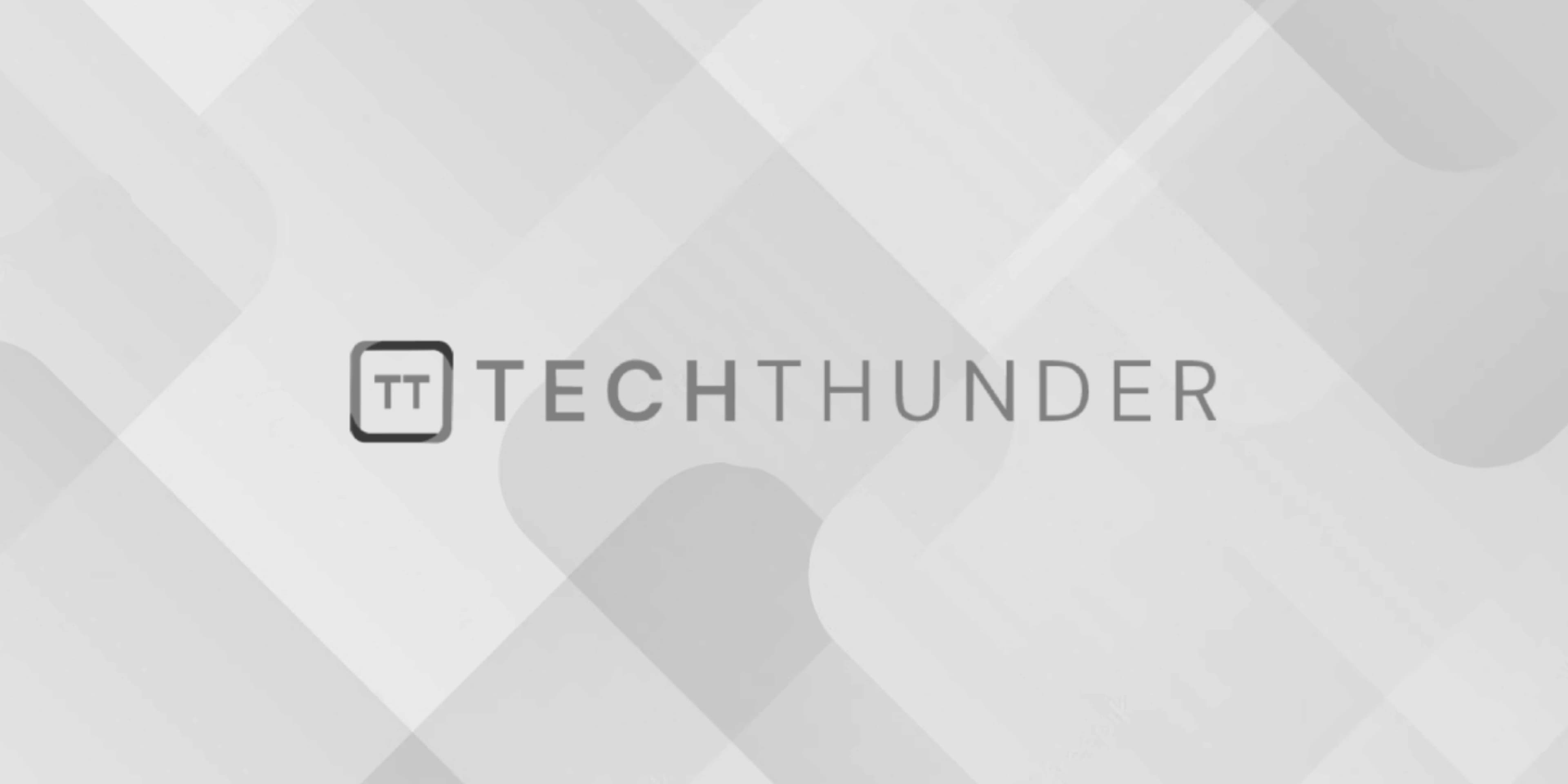
Git Modules in Python
Git modules in Python allow you to interact with Git repositories programmatically, enabling you to automate Git-related tasks or integrate Git functionality into your Python applications. You can work with Git modules using libraries or modules designed for this purpose. Here’s an overview of how to use Git modules in Python:
- GitPython:
GitPython is a popular Python library for working with Git repositories. It provides a high-level and Pythonic API for interacting with Git. You can install it using pip:
pip install GitPython
Here’s a basic example of how to use GitPython to clone a Git repository and perform some operations:
import git
# Clone a Git repository
repo = git.Repo.clone_from("https://github.com/username/repo.git", "/path/to/destination")
# Check the current branch
current_branch = repo.active_branch
print(f"Current branch: {current_branch}")
# Pull the latest changes
repo.remotes.origin.pull()
# Create and commit changes
repo.index.add(["file1.txt", "file2.txt"])
repo.index.commit("Commit message")
# Push changes to the remote repository
repo.remotes.origin.push()
GitPython provides a comprehensive set of features for working with Git repositories, including creating branches, merging, tagging, and more.
- pygit2:
pygit2 is another Python library that provides bindings to the libgit2 C library, allowing you to work with Git repositories programmatically. You can install it using pip:
pip install pygit2
Here’s a basic example of how to use pygit2:
import pygit2
# Clone a Git repository
repo = pygit2.clone_repository("https://github.com/username/repo.git", "/path/to/destination")
# Check the current branch
current_branch = repo.head.shorthand
print(f"Current branch: {current_branch}")
# Pull the latest changes
remote = repo.remotes["origin"]
remote.fetch()
remote_master = repo.lookup_reference(f"refs/remotes/origin/{current_branch}")
repo.checkout(remote_master)
# Create and commit changes
index = repo.index
index.add(["file1.txt", "file2.txt"])
index.write()
author = pygit2.Signature("Your Name", "your.email@example.com")
committer = author
tree = index.write_tree()
oid = repo.create_commit("refs/heads/master", author, committer, "Commit message", tree, [repo.head.target])
# Push changes to the remote repository
remote.push(["refs/heads/master"])
pygit2 provides lower-level access to Git operations compared to GitPython, and it can be more flexible but may require more code to accomplish some tasks.
These are two popular libraries for working with Git repositories in Python. You can choose the one that best suits your project’s needs based on your familiarity with Git and your specific requirements.