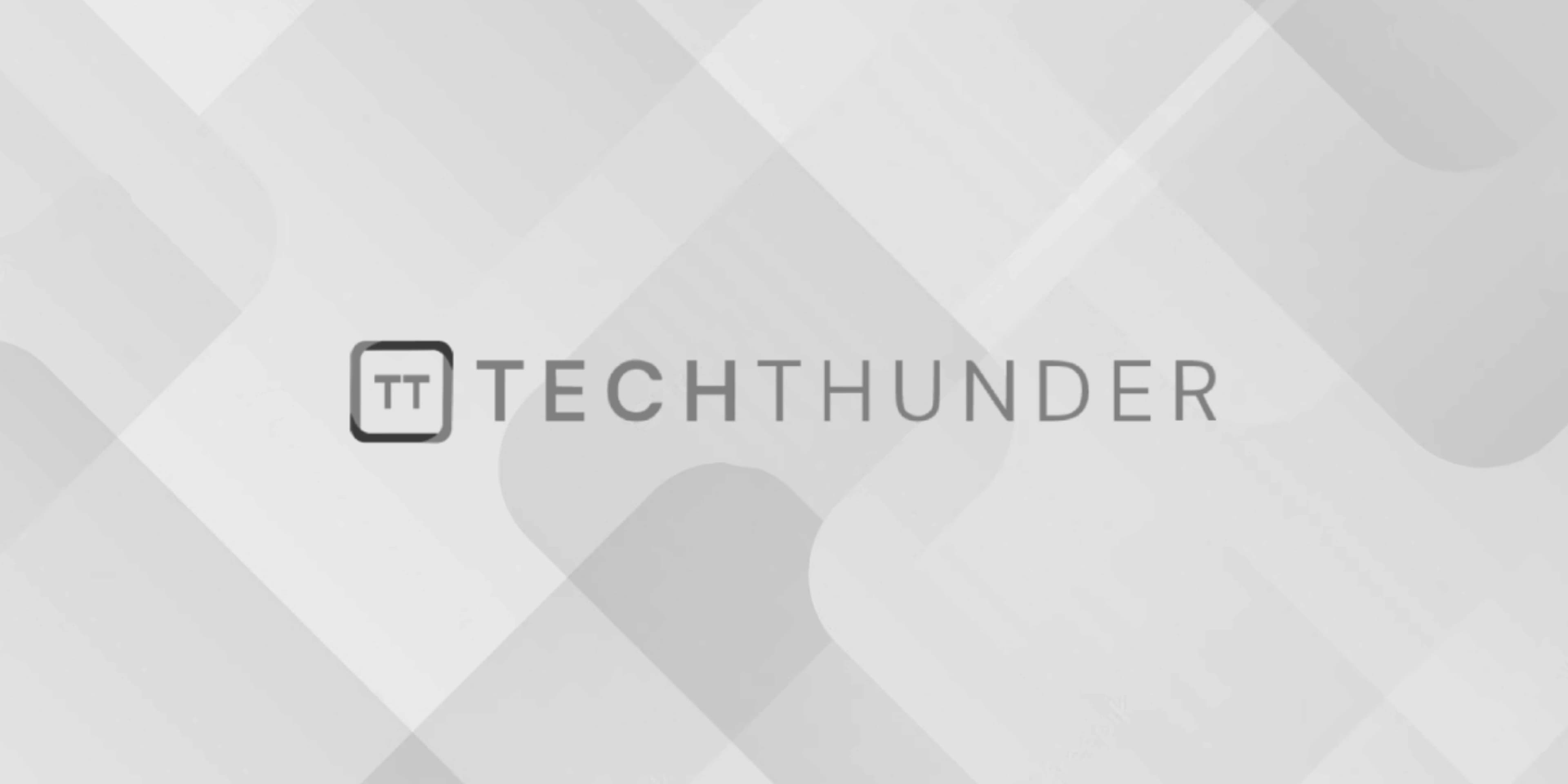
Python Progressbar Module
The progressbar
module in Python is a simple and easy-to-use library for creating text-based progress bars in command-line interfaces. It provides a way to visualize the progress of tasks, such as file downloads, data processing, or any other operation that can be tracked with a progress bar.
Here’s how you can use the progressbar
module to create a basic progress bar:
- Install the
progressbar2
package usingpip
:
pip install progressbar2
- Create a Python script to use the
progressbar
module:
import time
import progressbar
# Create a progress bar widget
bar = progressbar.ProgressBar(max_value=100)
# Simulate a time-consuming task
for i in range(101):
time.sleep(0.1) # Simulate some work
bar.update(i) # Update the progress bar
print("Task complete")
In this example:
- We import the
progressbar
module. - We create a progress bar widget with a maximum value of 100 using
progressbar.ProgressBar(max_value=100)
. - Inside the loop, we update the progress bar using
bar.update(i)
, wherei
is the current progress value. - The
time.sleep(0.1)
simulates some work being done.
When you run the script, you will see a text-based progress bar in the console that updates as the loop progresses.
The progressbar
module provides various configuration options and customization features, such as changing the appearance of the progress bar, displaying additional information, and handling exceptions. You can refer to the documentation for more details and examples.
Please note that there are different versions of the progressbar
library available (e.g., progressbar2
, progressbar33
, etc.), so make sure to install the appropriate version based on your requirements.