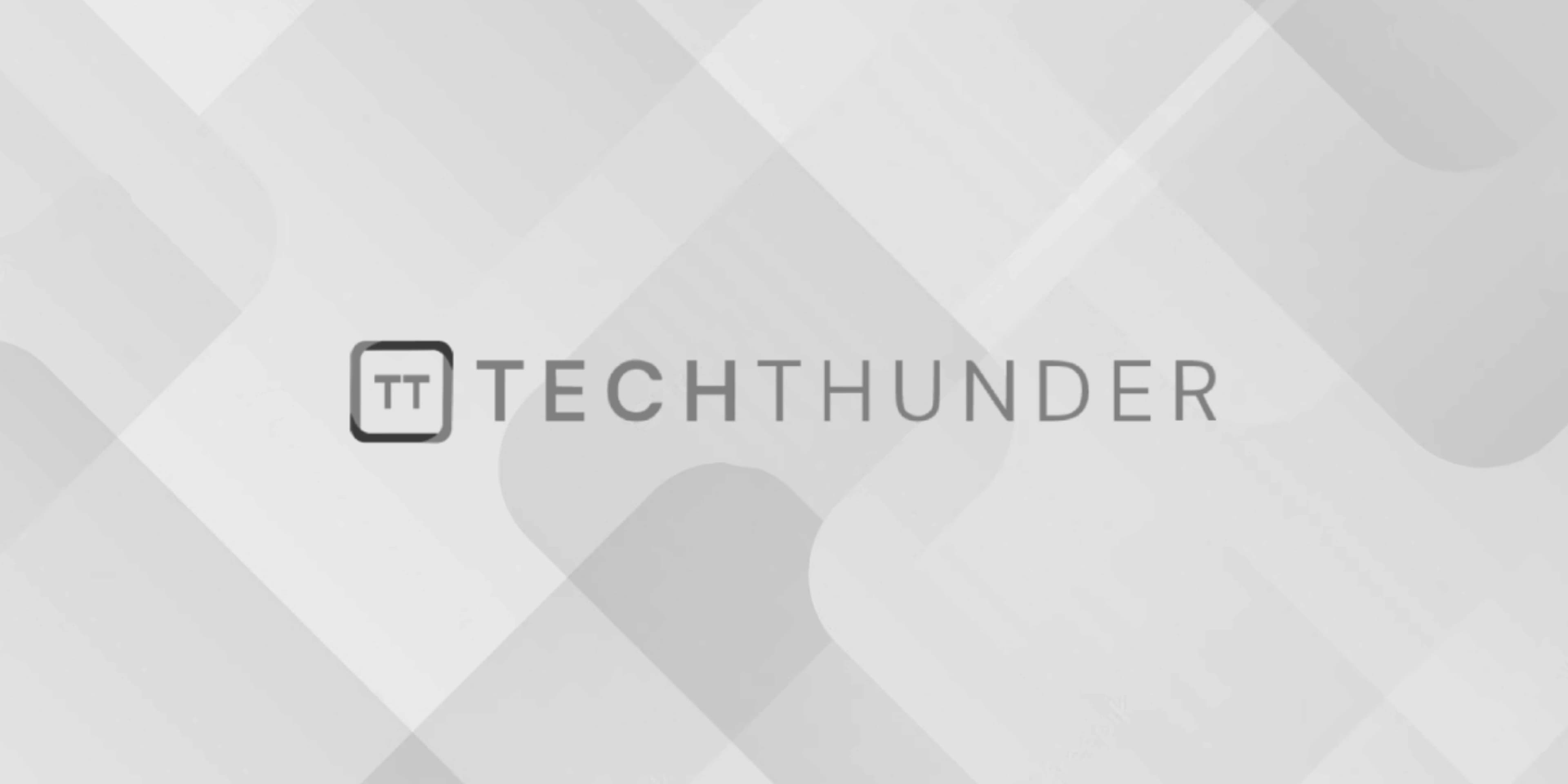
Collection Framework
The Java Collections Framework is a set of classes and interfaces in Java that provide a standardized way to handle and manipulate collections of objects. It was introduced in Java 2 to address the need for more efficient, flexible, and consistent data structures for storing and manipulating groups of objects. The framework is part of the java.util
package and is a fundamental part of Java programming. Key components of the Java Collections Framework include:
- Interfaces:
- Collection: The root interface for all collection classes. It defines the basic methods that all collections will have, such as
add()
,remove()
,contains()
, andsize()
. - Set: Represents a collection of elements with no duplicates. Implementations include
HashSet
,LinkedHashSet
, andTreeSet
. - List: Represents an ordered collection of elements, allowing duplicates. Implementations include
ArrayList
,LinkedList
, andVector
. - Queue: Represents a collection used for holding elements prior to processing. Implementations include
LinkedList
andPriorityQueue
. - Map: Represents a collection of key-value pairs. Implementations include
HashMap
,LinkedHashMap
, andTreeMap
. - Deque: Represents a double-ended queue, allowing elements to be added or removed from both ends. The primary implementation is
ArrayDeque
.
- Classes:
Collections
: A utility class that provides static methods for performing operations on collections, such as sorting, searching, and creating unmodifiable collections.Arrays
: A utility class for working with arrays, providing methods likeasList()
for converting arrays to lists.
- Concrete Implementations:
- A variety of concrete classes implement the collection interfaces, offering different trade-offs in terms of performance, order, and uniqueness.
- Common concrete classes include
ArrayList
,LinkedList
,HashSet
,TreeSet
,HashMap
, andTreeMap
, among others.
- Legacy Collections:
- Some older, less commonly used collection classes are part of the framework, such as
Hashtable
,Vector
, andStack
. While these classes are still available for compatibility reasons, newer alternatives likeHashMap
,ArrayList
, andLinkedList
are generally preferred.
- Iterators:
- The framework provides iterators for traversing collections. You can use these to loop through the elements of a collection without needing to know the underlying data structure.
- Generics:
- Generics were introduced in Java 5 to add type safety to the collections framework. You can specify the type of elements a collection will hold to avoid type-related errors.
The Java Collections Framework provides a rich set of data structures for storing and manipulating collections of objects, making it easier to manage data in a structured and efficient way. It plays a crucial role in Java programming and is a fundamental concept for anyone working with Java.