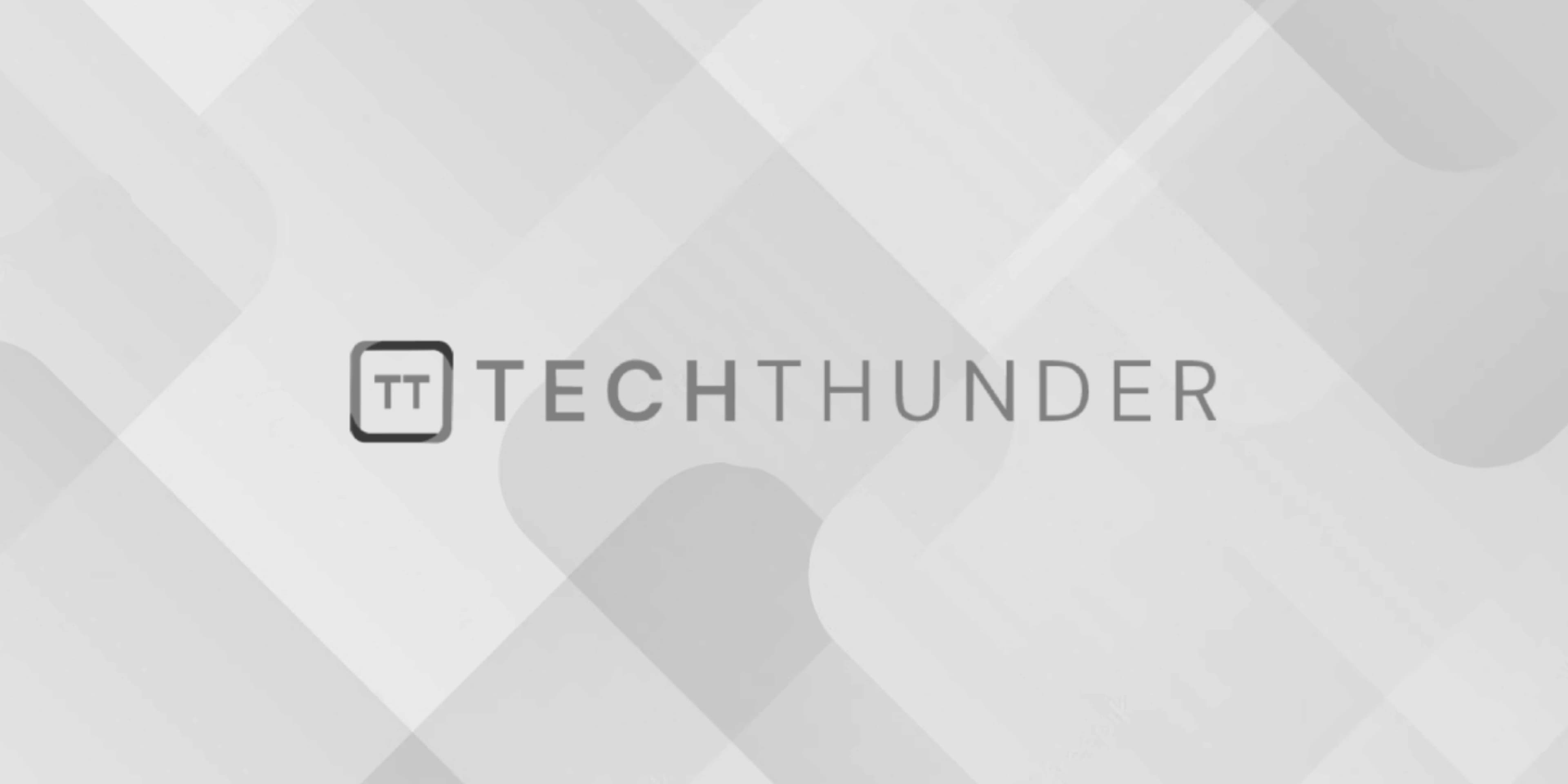
Java List Interface
The Java List
interface is a fundamental part of the Java Collections Framework, which is part of the java.util
package. The List
interface extends the Collection
interface and represents an ordered collection of elements where each element is associated with an index. Unlike sets, lists allow duplicate elements, which means you can have the same element appear multiple times in the list. Here are some key characteristics and methods associated with the List
interface:
- Order:
- Elements in a
List
are ordered by their index. This order is predictable and can be used to access elements in a specific sequence.
- Duplicates:
List
allows duplicate elements. You can have multiple occurrences of the same element in aList
.
- Random Access:
- You can access elements in a
List
by their index, which provides fast, constant-time access to any element in the list.
- Common Methods:
add(E element)
: Appends the specified element to the end of the list.add(int index, E element)
: Inserts the specified element at the specified index.get(int index)
: Retrieves the element at the specified index.set(int index, E element)
: Replaces the element at the specified index with the specified element.remove(int index)
: Removes the element at the specified index.indexOf(Object o)
: Returns the index of the first occurrence of the specified element in the list.lastIndexOf(Object o)
: Returns the index of the last occurrence of the specified element in the list.subList(int fromIndex, int toIndex)
: Returns a view of the portion of the list between the specified indices.listIterator()
: Returns an iterator for the list.
- Iterating:
- You can iterate through the elements in a
List
using various methods, such as enhanced for loops, iterators, and list iterators.
- Implementations:
- There are several classes that implement the
List
interface in Java, includingArrayList
,LinkedList
, andVector
.
The List
interface is widely used in Java programming for situations where you need an ordered collection of elements with the ability to access elements by index and the ability to have duplicate elements. Depending on your specific requirements and use cases, you can choose the appropriate List
implementation that suits your needs.