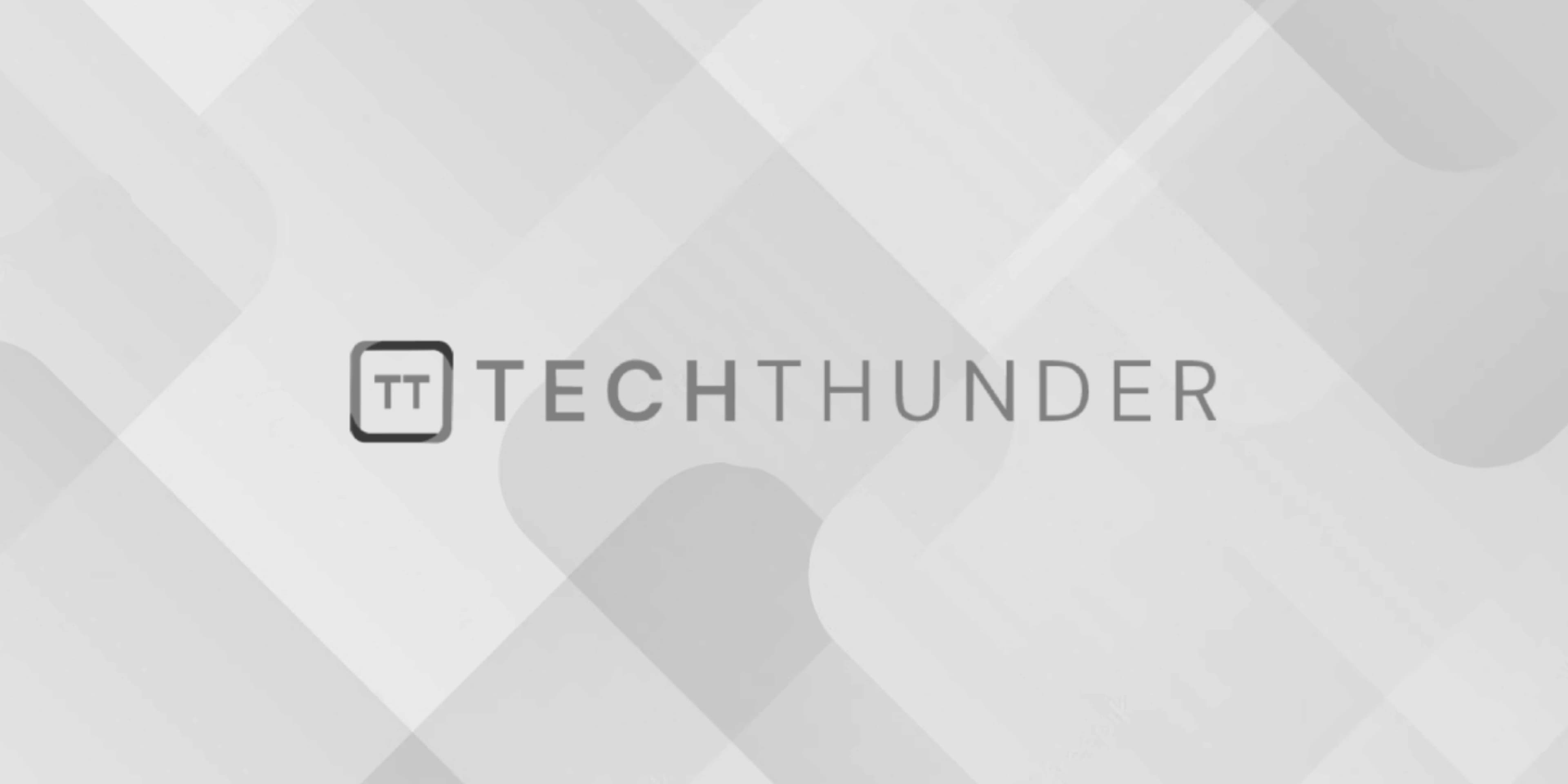
Java LinkedHashMap
The Java LinkedHashMap
is a class that implements the Map
interface and extends the HashMap
class. It combines the features of a hash table and a linked list to provide predictable and ordered traversal of key-value pairs. In other words, a LinkedHashMap
maintains the order in which elements were inserted or the order they were accessed, depending on the constructor used. Here are some key features and usage guidelines for LinkedHashMap
:
- Order Preservation:
- A
LinkedHashMap
maintains the order of elements, which means that you can iterate through the key-value pairs in the order they were inserted or accessed.
- Hash Table Underlying Structure:
- Like a
HashMap
, aLinkedHashMap
uses a hash table to store key-value pairs, allowing for efficient retrieval and storage of elements.
- Constructor Options:
LinkedHashMap
provides two constructors:- The default constructor, which maintains the order in which elements were inserted.
- A constructor that accepts a boolean argument for access-order mode. In access-order mode, elements are ordered based on their access history, with the most recently accessed elements appearing at the end.
- Efficient Retrieval:
- Similar to a
HashMap
, aLinkedHashMap
provides O(1) average-case time complexity for basic operations such asget()
andput()
.
- Null Keys and Values:
LinkedHashMap
allows onenull
key and multiplenull
values.
- Common Methods:
put(K key, V value)
: Associates the specified key with the specified value in the map.get(Object key)
: Retrieves the value associated with the given key.remove(Object key)
: Removes the key-value pair associated with the given key.containsKey(Object key)
: Checks if the map contains the specified key.keySet()
: Returns a set containing all the keys in the map.values()
: Returns a collection of all the values in the map.entrySet()
: Returns a set of key-value pairs (entries).
Here’s an example of using LinkedHashMap
:
import java.util.LinkedHashMap;
import java.util.Map;
public class LinkedHashMapExample {
public static void main(String[] args) {
// Creating a LinkedHashMap that maintains insertion order
Map<String, Integer> insertionOrderedMap = new LinkedHashMap<>();
// Adding key-value pairs
insertionOrderedMap.put("Alice", 25);
insertionOrderedMap.put("Bob", 30);
insertionOrderedMap.put("Charlie", 35);
// Iterating through key-value pairs in insertion order
for (Map.Entry<String, Integer> entry : insertionOrderedMap.entrySet()) {
System.out.println(entry.getKey() + " is " + entry.getValue() + " years old.");
}
// Creating a LinkedHashMap that maintains access order
Map<String, Integer> accessOrderedMap = new LinkedHashMap<>(16, 0.75f, true);
// Adding key-value pairs
accessOrderedMap.put("Alice", 25);
accessOrderedMap.put("Bob", 30);
accessOrderedMap.put("Charlie", 35);
// Accessing "Alice" to change its order
accessOrderedMap.get("Alice");
// Iterating through key-value pairs in access order
for (Map.Entry<String, Integer> entry : accessOrderedMap.entrySet()) {
System.out.println(entry.getKey() + " is " + entry.getValue() + " years old.");
}
}
}
In the example above, the first LinkedHashMap
maintains the order in which elements were inserted, and the second one maintains access order, so the elements are ordered based on their access history. This allows you to choose the order that best suits your needs when using LinkedHashMap
.