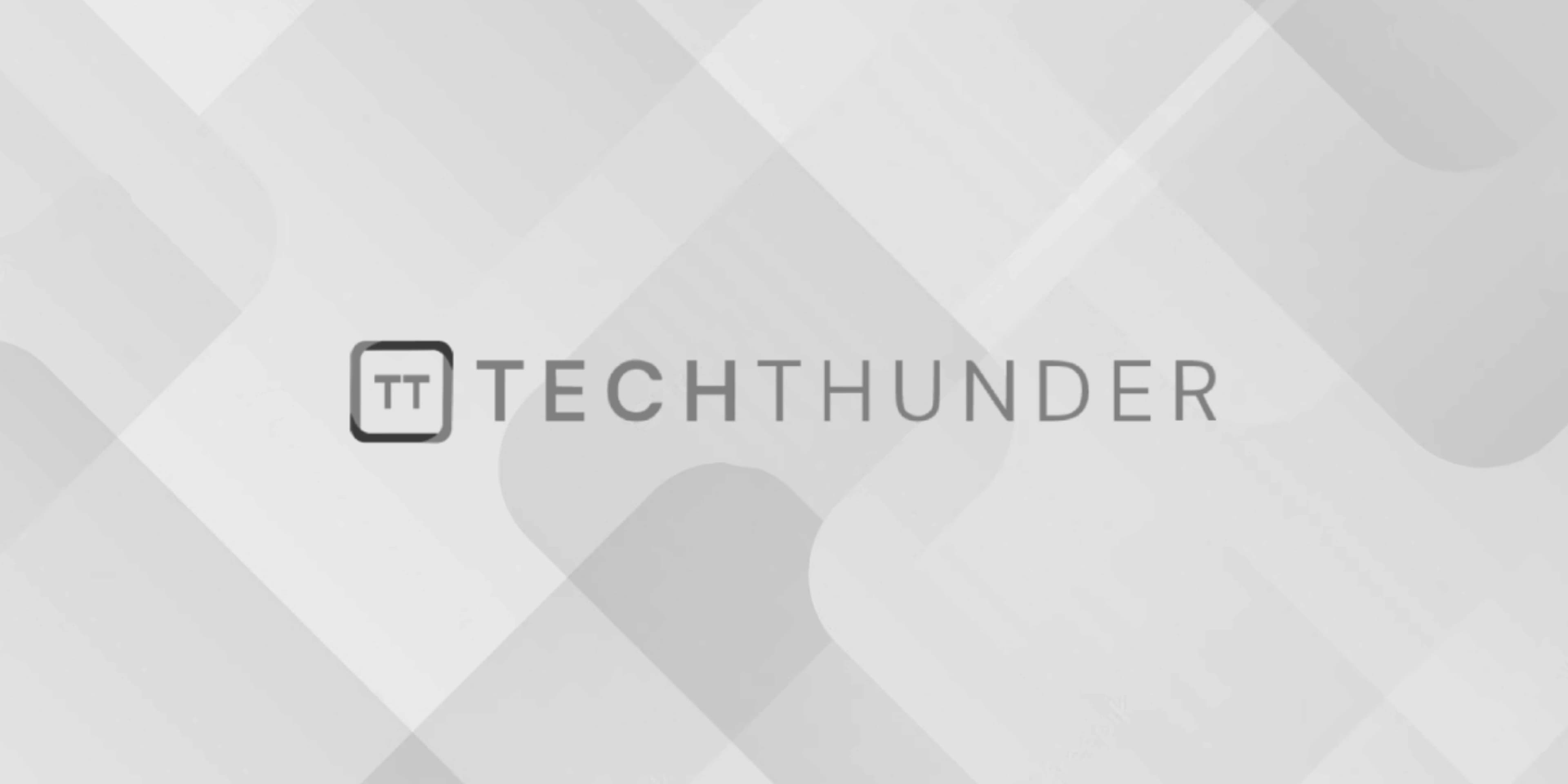
143 views
Java Collections class
The Java java.util.Collections
class is a utility class that provides various static methods to manipulate and operate on collections, including List
, Set
, and Map
. These methods help you perform common operations such as sorting, searching, shuffling, and more. Here are some of the key methods and operations provided by the Collections
class:
- Sorting:
sort(List<T> list)
: Sorts the elements in aList
in natural order using their natural ordering (comparable) or a custom comparator.reverse(List<T> list)
: Reverses the order of elements in aList
.shuffle(List<T> list)
: Shuffles the elements in aList
randomly.swap(List<?> list, int i, int j)
: Swaps the elements at the specified positions in aList
.
- Searching and Retrieval:
binarySearch(List<? extends T> list, T key)
: Performs a binary search on a sortedList
to find the index of the specified element.max(Collection<? extends T> coll)
: Returns the maximum element in the collection.min(Collection<? extends T> coll)
: Returns the minimum element in the collection.
- Synchronization:
synchronizedCollection(Collection<T> c)
: Returns a synchronized (thread-safe) view of a collection.synchronizedList(List<T> list)
: Returns a synchronized (thread-safe) view of a list.synchronizedSet(Set<T> s)
: Returns a synchronized (thread-safe) view of a set.synchronizedMap(Map<K,V> m)
: Returns a synchronized (thread-safe) view of a map.
- Unmodifiable Collections:
unmodifiableCollection(Collection<? extends T> c)
: Returns an unmodifiable view of a collection.unmodifiableList(List<? extends T> list)
: Returns an unmodifiable view of a list.unmodifiableSet(Set<? extends T> s)
: Returns an unmodifiable view of a set.unmodifiableMap(Map<? extends K, ? extends V> m)
: Returns an unmodifiable view of a map.
- Singleton Collections:
singleton(T o)
: Returns an immutable set containing a single specified element.singletonList(T o)
: Returns an immutable list containing a single specified element.singletonMap(K key, V value)
: Returns an immutable map containing a single key-value pair.
- Miscellaneous:
fill(List<? super T> list, T obj)
: Replaces all elements in the list with the specified object.frequency(Collection<?> c, Object o)
: Returns the number of times the specified element appears in the collection.addAll(Collection<? super T> c, T... elements)
: Adds all elements in the array to the collection.
The Collections
class provides a wide range of utility methods that simplify common operations on collections, making it easier to work with them. It’s especially useful when you need to perform operations that are not provided directly by the collection interfaces.