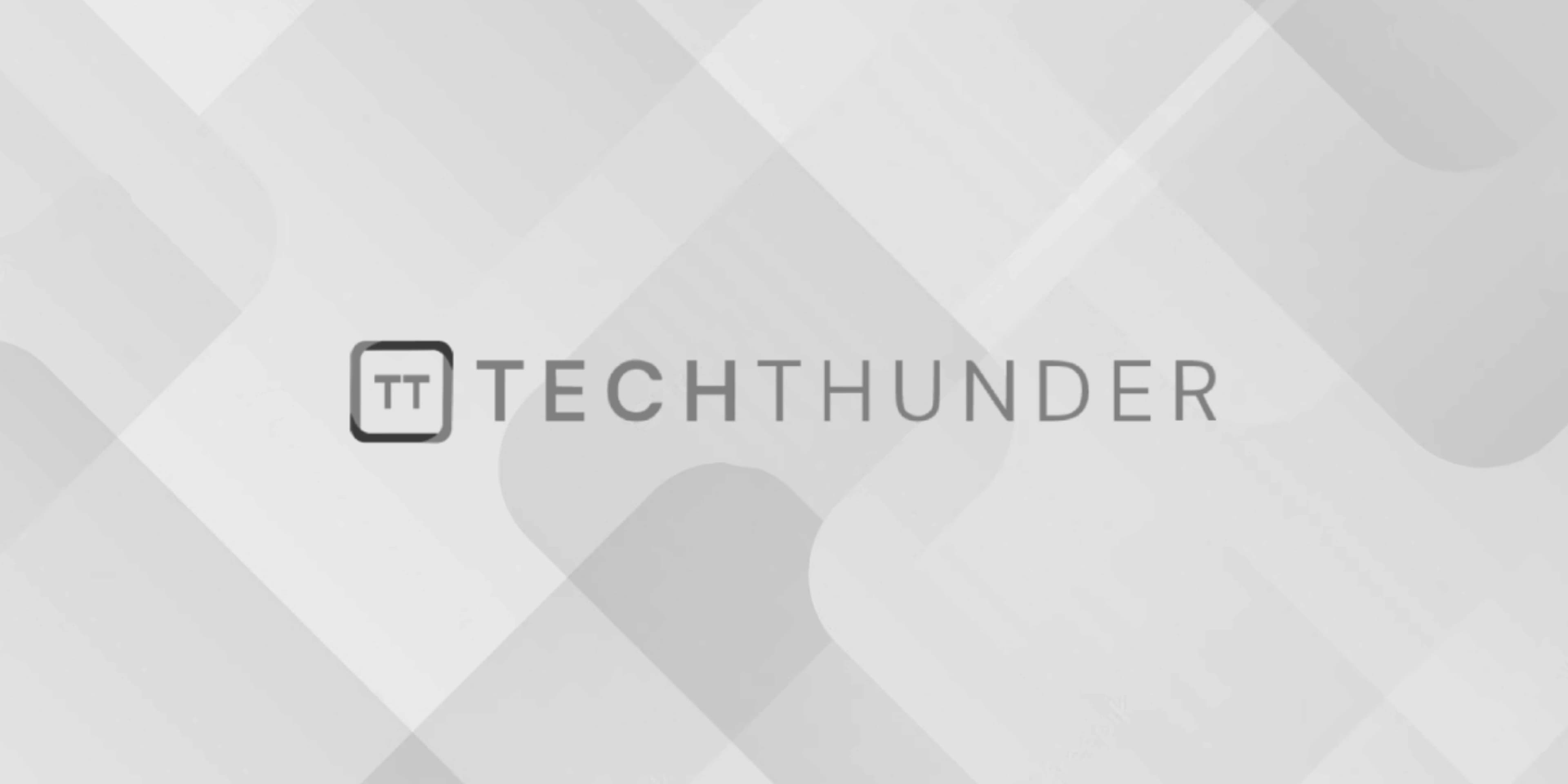
Java Comparable interface
The Comparable
interface in Java is used to define a natural ordering for a class. By implementing the Comparable
interface, a class specifies how its instances should be compared to each other for sorting purposes. This allows objects of that class to be sorted using methods like Collections.sort()
or Arrays.sort()
.
The Comparable
interface has a single method:
int compareTo(T other)
The compareTo
method returns a negative integer, zero, or a positive integer depending on whether the current object is less than, equal to, or greater than the other
object. By convention, if compareTo
returns a negative value, the current object is considered “less than” the other object; if it returns zero, they are considered equal; and if it returns a positive value, the current object is considered “greater than” the other object.
Here’s an example of how you might implement the Comparable
interface to define natural ordering for a custom class:
public class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
// Implement the compareTo method to define natural ordering
@Override
public int compareTo(Student other) {
// Compare based on age
return Integer.compare(this.age, other.age);
}
@Override
public String toString() {
return "Student [name=" + name + ", age=" + age + "]";
}
}
With the compareTo
method implemented, you can now easily sort instances of the Student
class:
import java.util.*;
public class ComparableExample {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 21));
students.add(new Student("Bob", 19));
students.add(new Student("Charlie", 20));
Collections.sort(students); // Sort based on age
for (Student student : students) {
System.out.println(student);
}
}
}
The Comparable
interface is useful when you want to establish a default sorting order for your custom classes. If you need to sort objects using different criteria in different situations, you can use the Comparator
interface to define custom comparison logic without modifying the class itself.