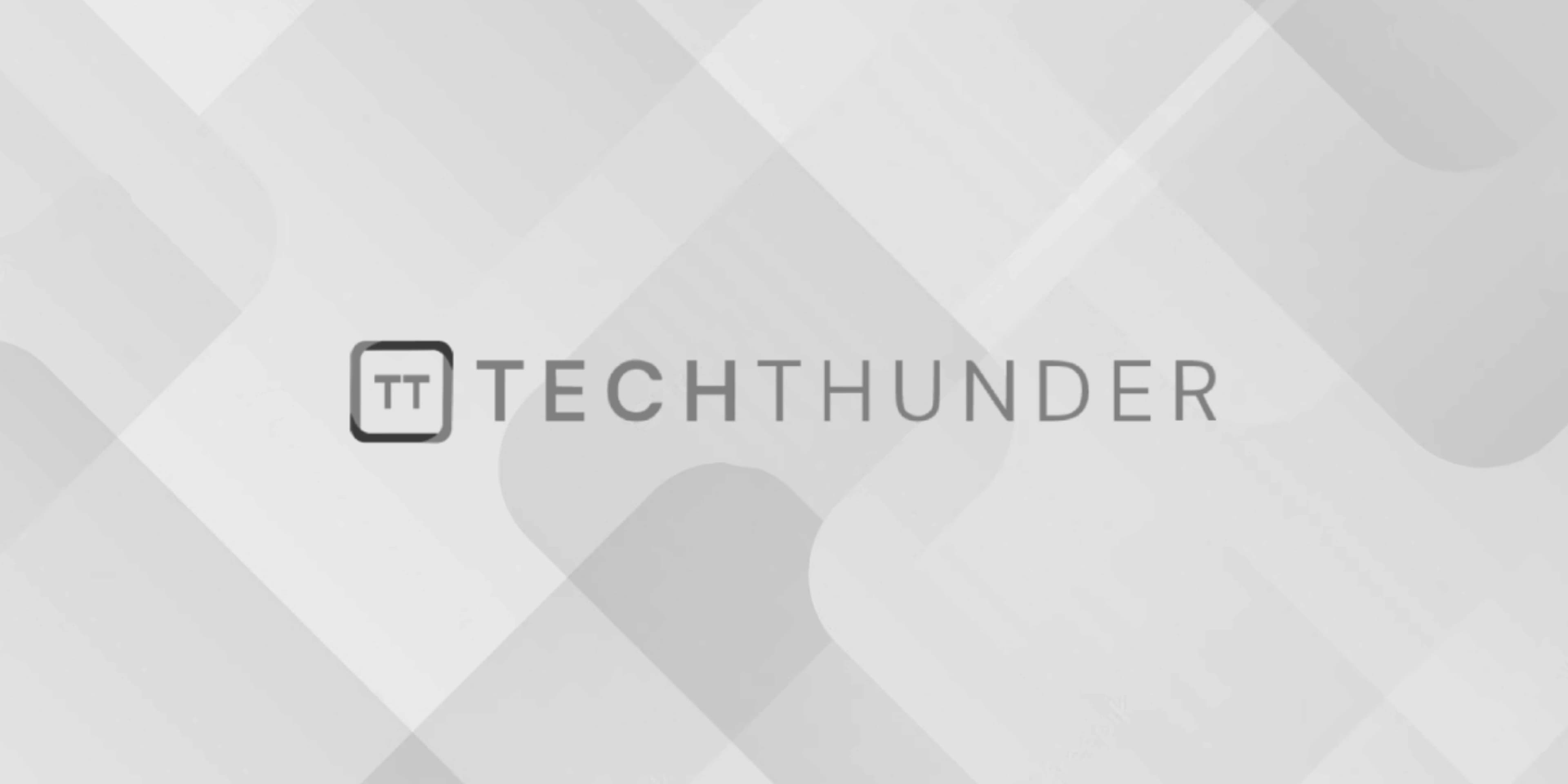
Java Comparator interface
The Comparator
interface in Java is used to define custom comparison logic for objects that don’t have a natural ordering or when you want to sort objects based on different criteria than their natural ordering. The Comparator
interface allows you to create multiple ways to compare objects without altering their class or implementing the Comparable
interface.
The Comparator
interface has two main methods:
int compare(T obj1, T obj2)
boolean equals(Object obj)
compare(T obj1, T obj2)
: This method compares two objects,obj1
andobj2
, and returns a negative integer, zero, or a positive integer, depending on whetherobj1
is less than, equal to, or greater thanobj2
. The same convention applies here as with thecompareTo
method of theComparable
interface.equals(Object obj)
: This method checks if the comparator is equal to another comparator. This is primarily used for comparison optimization and caching.
Here’s an example of how you might implement the Comparator
interface to define custom sorting logic for a class:
import java.util.*;
public class StudentComparator implements Comparator<Student> {
@Override
public int compare(Student student1, Student student2) {
// Compare based on name length
return Integer.compare(student1.getName().length(), student2.getName().length());
}
}
You can then use this custom comparator to sort instances of the Student
class:
import java.util.*;
public class ComparatorExample {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 21));
students.add(new Student("Bob", 19));
students.add(new Student("Charlie", 20));
Comparator<Student> nameLengthComparator = new StudentComparator();
Collections.sort(students, nameLengthComparator); // Sort based on name length
for (Student student : students) {
System.out.println(student);
}
}
}
The Comparator
interface is useful when you want to sort objects using different criteria without modifying their class. It provides flexibility in sorting logic and is particularly handy when you’re working with classes that you can’t modify or classes that don’t have a natural ordering.