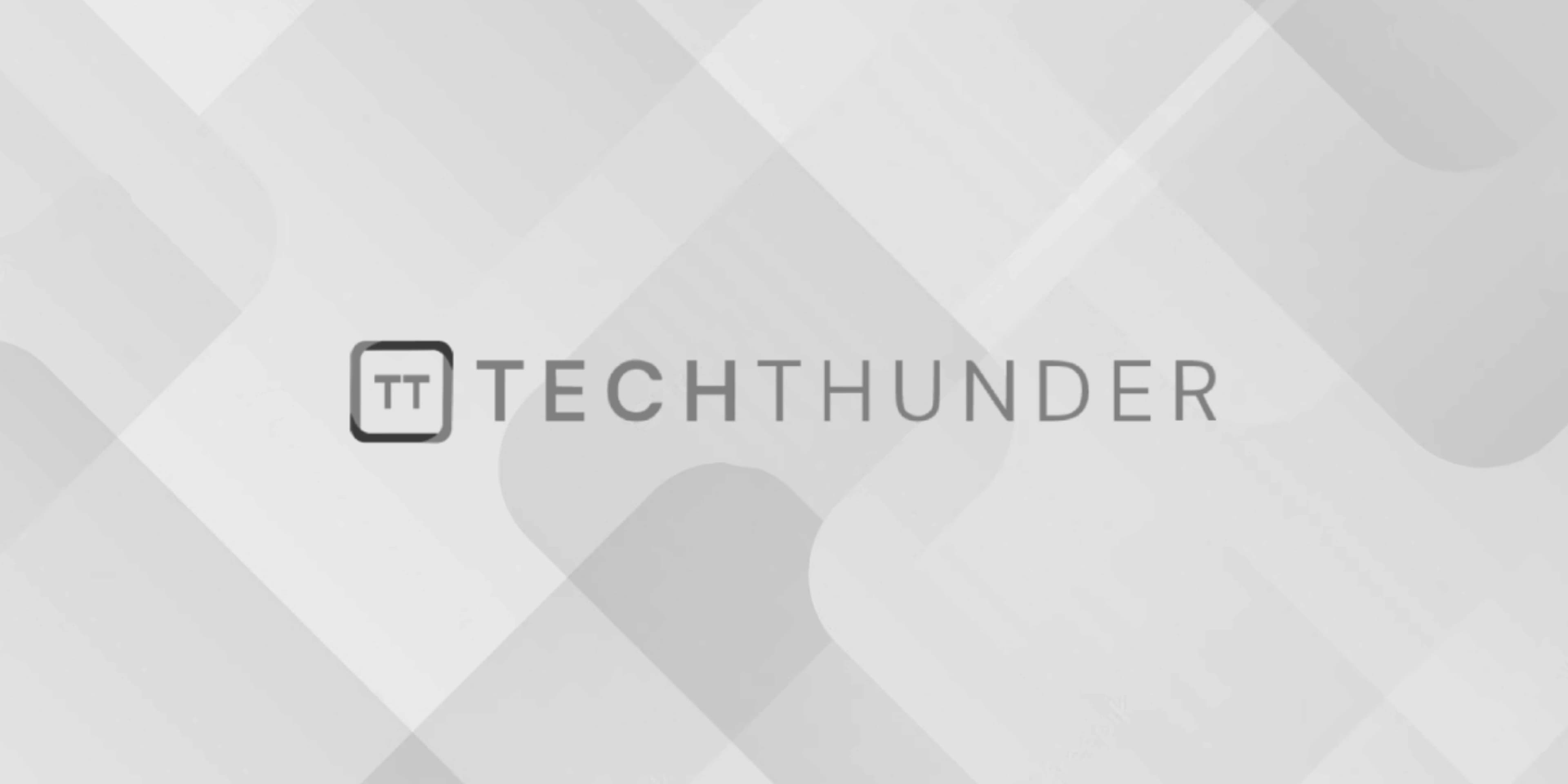
Java EnumMap
EnumMap
is a specialized and efficient implementation of the Map
interface in Java, designed to work specifically with enumerated types (enums). It is part of the Java Collections Framework and provides a way to represent and manipulate key-value pairs where the keys are enum constants and the values are associated data. Enum maps are highly efficient and provide some advantages over general-purpose maps when working with enums. Here are some key points about EnumMap
:
- Restricted to Enum Keys:
EnumMap
is designed to work only with enumerated types (enums). The keys in anEnumMap
must be enum constants of a specific enum type.
- Efficiency:
EnumMap
is highly efficient and optimized for memory usage and performance. It is implemented as an array, where the indices correspond to the ordinal values of the enum constants.
- Type Safe:
- Because it’s restricted to enum keys,
EnumMap
is type-safe. You can’t accidentally use keys of the wrong type.
- Creation:
- You can create an
EnumMap
by specifying the enum class for which the map is created. The enum class defines the set of valid keys. - Example of creating an
EnumMap
:
EnumMap<Day, String> dayActivities = new EnumMap<>(Day.class);
- Methods:
EnumMap
provides methods for map operations likeput()
,get()
,remove()
,containsKey()
,isEmpty()
, and more.
- Iteration Order:
EnumMap
maintains the natural iteration order of elements based on the order in which the enum constants are declared. This can be useful when iterating through the map.
- No Null Keys:
EnumMap
does not allownull
keys. If you attempt to use anull
key, it will throw aNullPointerException
.
- Null Values:
EnumMap
allowsnull
values.
Here’s an example of using EnumMap
:
import java.util.EnumMap;
public class EnumMapExample {
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public static void main(String[] args) {
EnumMap<Day, String> dayActivities = new EnumMap<>(Day.class);
// Adding key-value pairs
dayActivities.put(Day.MONDAY, "Go to work");
dayActivities.put(Day.FRIDAY, "Relax and have fun");
// Retrieving values by key
String mondayActivity = dayActivities.get(Day.MONDAY);
System.out.println("Monday's activity: " + mondayActivity);
// Iterating through key-value pairs
for (Day day : Day.values()) {
String activity = dayActivities.get(day);
if (activity != null) {
System.out.println(day + ": " + activity);
}
}
}
}
In this example, we create an EnumMap
to represent daily activities. The keys are enum constants from the Day
enum, and the values are associated activities. EnumMap
is a powerful and efficient way to work with enums and key-value pairs associated with enum constants.