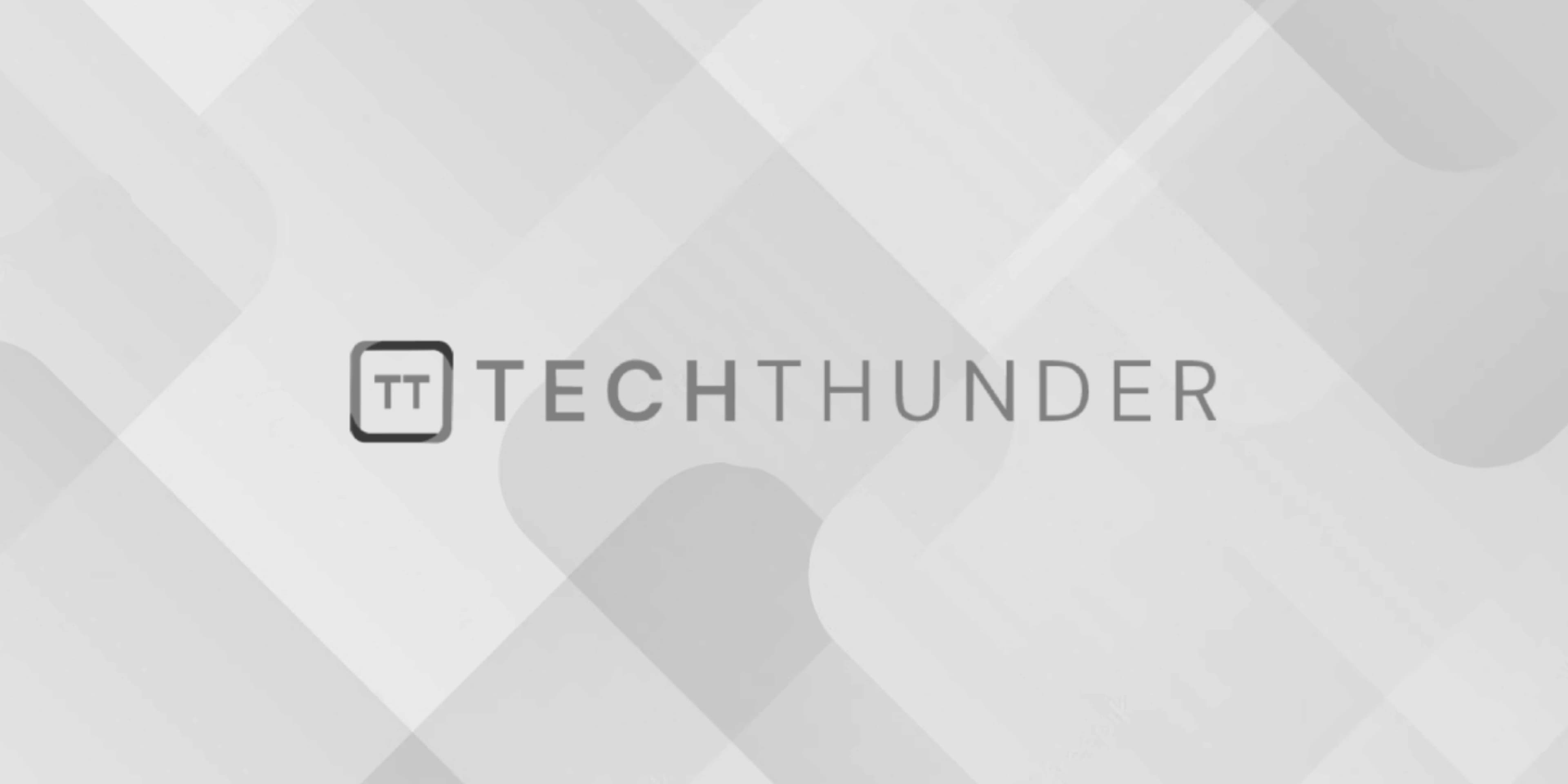
Java ArrayList
The Java ArrayList
is a dynamic array-based data structure that is part of the Java Collections Framework. It provides a resizable array, which means that you can add or remove elements at runtime, and it automatically resizes itself to accommodate the elements. ArrayList
is part of the java.util
package, and it offers several advantages over regular arrays, including dynamic sizing, ease of use, and a wide range of useful methods. Here are some key features and usage guidelines for ArrayList
:
- Dynamic Sizing:
- Unlike regular arrays,
ArrayList
can grow or shrink in size as elements are added or removed. It automatically handles resizing, so you don’t need to worry about managing the array’s capacity.
- Generics:
ArrayList
can be parametrized with a specific data type (using generics) to ensure type safety. For example, you can have anArrayList
of integers, strings, or any other object type.
- Common Operations:
add(E element)
: Appends an element to the end of the list.get(int index)
: Retrieves an element at a specific index.set(int index, E element)
: Replaces the element at a specific index with the provided element.remove(int index)
: Removes the element at a specific index.size()
: Returns the number of elements in the list.isEmpty()
: Checks if the list is empty.contains(Object o)
: Checks if the list contains a specific element.clear()
: Removes all elements from the list.
- Iteration:
- You can use enhanced for loops or iterators to iterate through the elements in an
ArrayList
.
- Performance:
ArrayList
provides O(1) time complexity for adding and accessing elements by index, making it efficient for these operations.- Removing elements from the middle of an
ArrayList
has a time complexity of O(n) because all subsequent elements need to be shifted to fill the gap.
- Resizing:
- When elements are added beyond the initial capacity,
ArrayList
resizes itself by creating a larger underlying array and copying the elements. This resizing process has a time complexity of O(n) in the worst case but is usually amortized to be O(1) for adding an element.
- Null Elements:
ArrayList
allowsnull
elements.
Here’s an example of using ArrayList
:
import java.util.ArrayList;
public class ArrayListExample {
public static void main(String[] args) {
// Creating an ArrayList of integers
ArrayList<Integer> numbers = new ArrayList<>();
// Adding elements
numbers.add(10);
numbers.add(20);
numbers.add(30);
// Accessing elements
int firstNumber = numbers.get(0); // 10
// Iterating through elements
for (int number : numbers) {
System.out.println(number);
}
}
}
ArrayList
is a versatile and widely used data structure in Java for dynamic collections of elements. It’s a fundamental building block for managing lists of data efficiently and is commonly used in a wide range of Java applications.