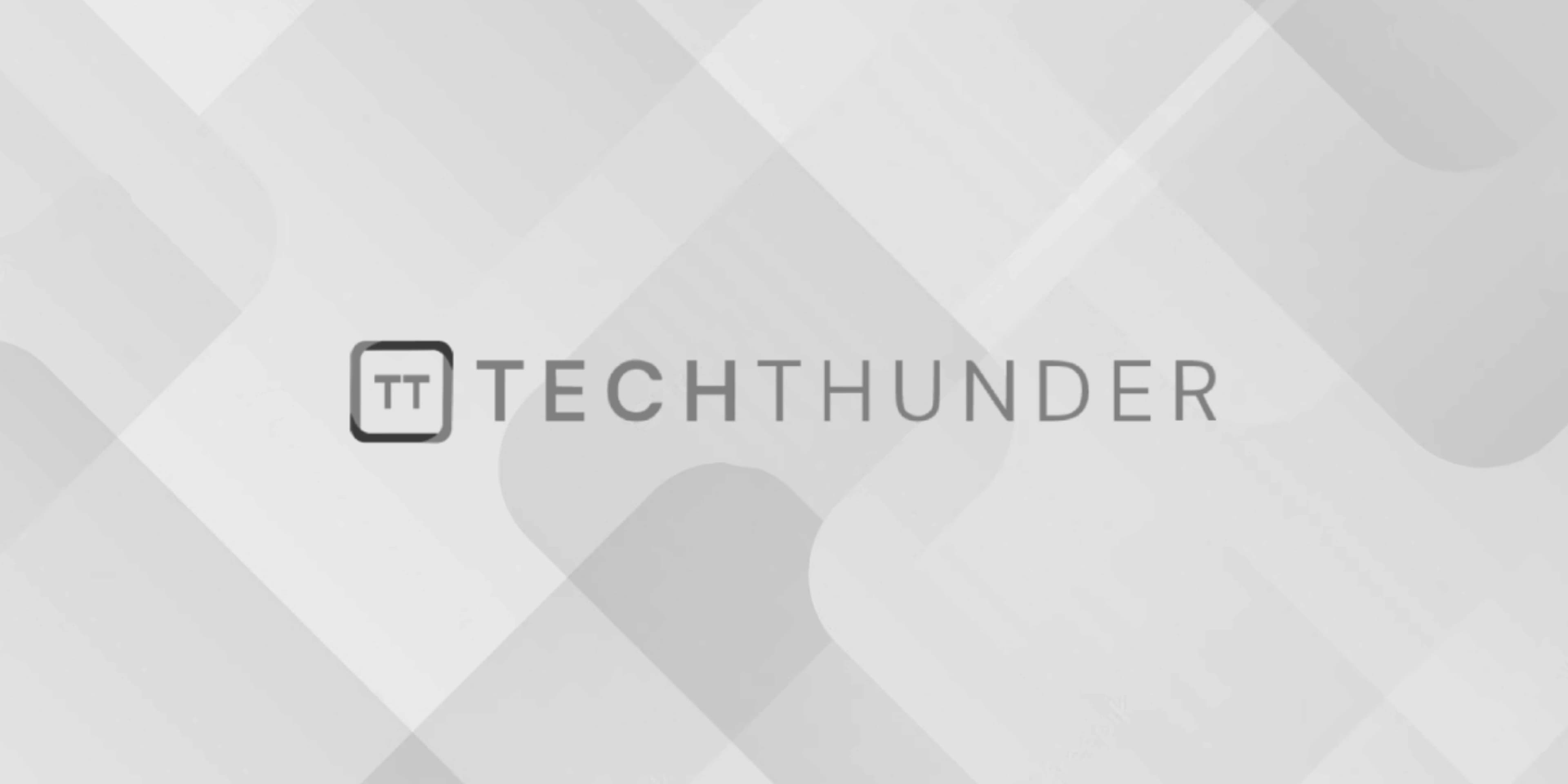
167 views
Java Map Interface
Th Java Map
interface is a part of the Java Collections Framework and is located in the java.util
package. It represents a collection of key-value pairs, where each key is associated with a value. The Map
interface is designed to allow efficient retrieval and manipulation of key-value pairs, and it does not allow duplicate keys.
Here are some key characteristics and methods associated with the Map
interface:
- Key-Value Pairs:
- A
Map
is a collection of key-value pairs, where each key is unique within the map. - Each key is associated with exactly one value.
- Common Implementations:
- Some commonly used implementations of the
Map
interface include:HashMap
: A widely used implementation based on a hash table that provides fast retrieval times for keys.TreeMap
: An implementation based on a Red-Black tree that stores keys in a sorted order.LinkedHashMap
: Combines features of a hash map and a linked list to maintain insertion order.Hashtable
: A legacy implementation that is thread-safe, but it’s generally recommended to useHashMap
in modern Java.
- Methods:
put(K key, V value)
: Associates the specified key with the specified value in the map.get(Object key)
: Retrieves the value associated with the given key.remove(Object key)
: Removes the key-value pair associated with the given key.containsKey(Object key)
: Checks if the map contains the specified key.containsValue(Object value)
: Checks if the map contains the specified value.keySet()
: Returns a set containing all the keys in the map.values()
: Returns a collection of all the values in the map.entrySet()
: Returns a set of key-value pairs (entries).
Here is an example of using a HashMap
:
import java.util.HashMap;
import java.util.Map;
public class MapExample {
public static void main(String[] args) {
// Creating a HashMap
Map<String, Integer> ages = new HashMap<>();
// Adding key-value pairs
ages.put("Alice", 25);
ages.put("Bob", 30);
ages.put("Charlie", 35);
// Retrieving values by key
int age = ages.get("Alice");
System.out.println("Alice's age is " + age);
// Checking if a key exists
if (ages.containsKey("David")) {
System.out.println("David's age is " + ages.get("David"));
} else {
System.out.println("David's age is not available.");
}
// Iterating through key-value pairs
for (Map.Entry<String, Integer> entry : ages.entrySet()) {
System.out.println(entry.getKey() + " is " + entry.getValue() + " years old.");
}
}
}
The Map
interface and its implementations are fundamental for various data storage and retrieval needs in Java. They allow efficient access to values based on their associated keys, making them valuable in a wide range of applications.