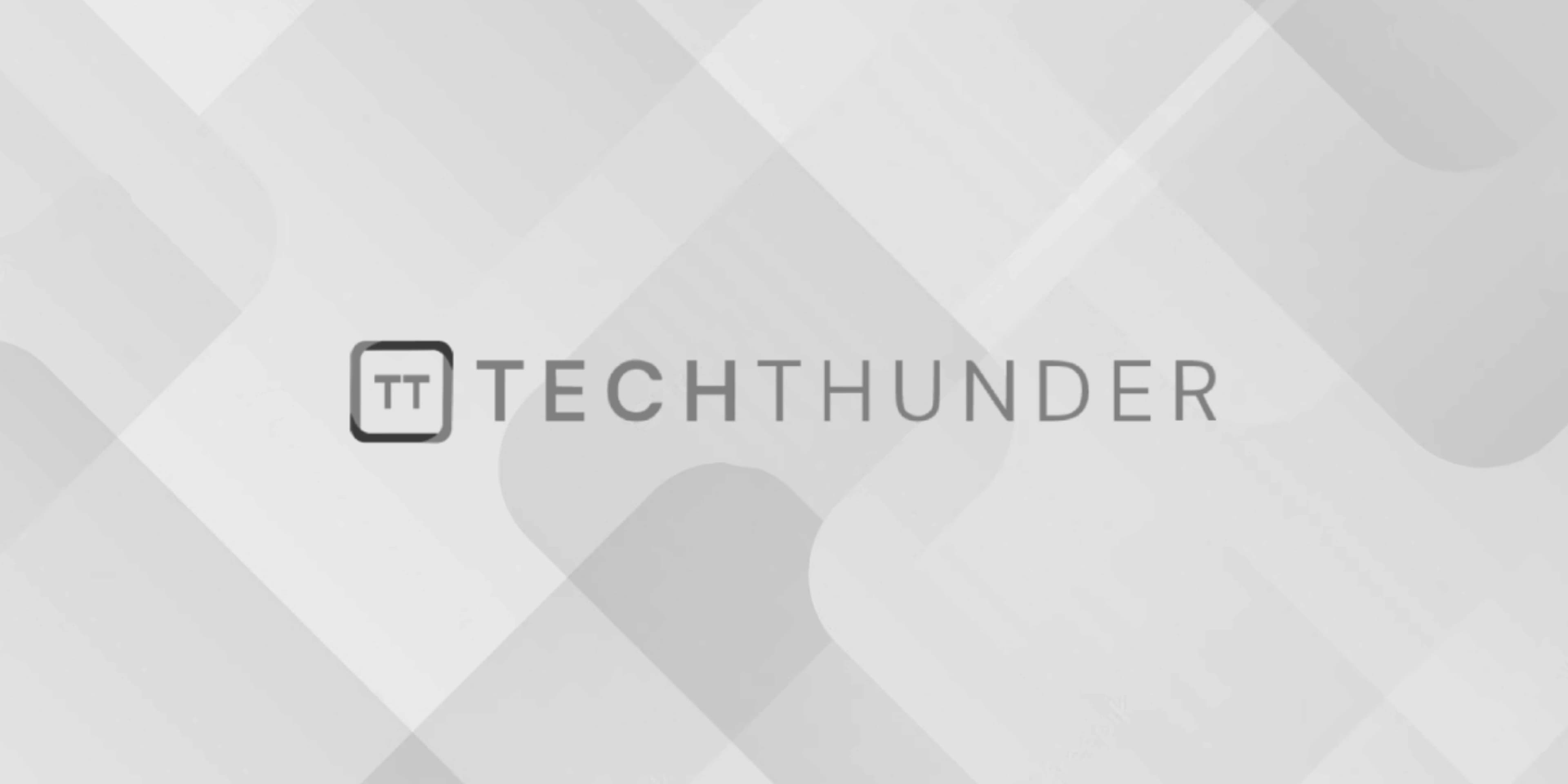
Java Deque Interface
The Java Deque
interface, short for “double-ended queue,” is a part of the Java Collections Framework. It extends the Queue
interface and represents a linear collection that supports element insertion and removal at both ends. In other words, it provides a flexible way to work with a queue-like structure where elements can be added or removed from both the front and the back.
The Deque
interface defines several methods for performing various operations on the double-ended queue, including:
- Adding Elements:
addFirst(E e)
/offerFirst(E e)
: Inserts the specified element at the front of the deque.addLast(E e)
/offerLast(E e)
: Inserts the specified element at the end of the deque.
- Removing Elements:
removeFirst()
/pollFirst()
: Removes and returns the first element from the deque.removeLast()
/pollLast()
: Removes and returns the last element from the deque.
- Peeking Elements:
getFirst()
/peekFirst()
: Retrieves the first element without removing it.getLast()
/peekLast()
: Retrieves the last element without removing it.
- Stack-like Operations:
push(E e)
: Adds an element to the front of the deque (similar to stack’s push operation).pop()
: Removes and returns the first element from the front of the deque (similar to stack’s pop operation).
The Deque
interface is implemented by several classes in Java, including:
ArrayDeque
: A resizable array-based implementation of a double-ended queue.LinkedList
: A linked-list-based implementation of a double-ended queue.
Here’s an example of how you might use the Deque
interface:
import java.util.*;
public class DequeExample {
public static void main(String[] args) {
Deque<String> deque = new LinkedList<>(); // You can also use ArrayDeque
deque.addLast("Element 1");
deque.addFirst("Element 2");
deque.addLast("Element 3");
System.out.println(deque); // Output: [Element 2, Element 1, Element 3]
System.out.println(deque.pollFirst()); // Output: Element 2
System.out.println(deque); // Output: [Element 1, Element 3]
System.out.println(deque.peekLast()); // Output: Element 3
}
}
Remember that the Deque
interface provides both queue-like and stack-like operations, making it a versatile choice for scenarios where you need to manipulate elements from both ends of the collection.