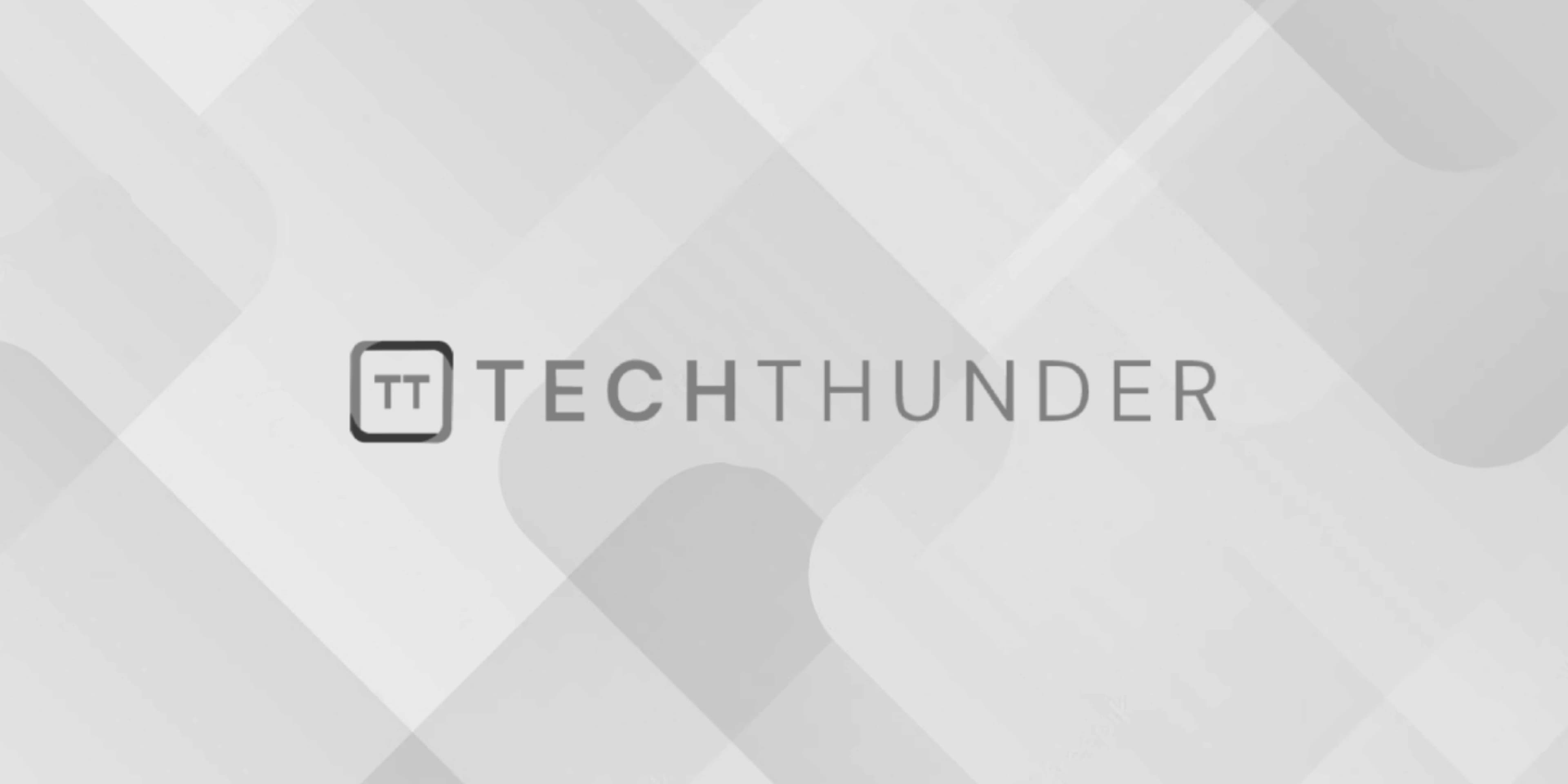
Java TreeSet
The Java TreeSet
is a class that implements the Set
interface and is part of the Java Collections Framework, located in the java.util
package. It is a NavigableSet that uses a Red-Black tree as its underlying data structure. TreeSet
provides a sorted, ordered set of elements with no duplicates. Elements are sorted in natural order or based on a specified comparator. Here are some key characteristics and usage guidelines for TreeSet
:
- Sorted Order:
TreeSet
maintains elements in sorted order. Elements are stored in ascending order by default, or you can provide a customComparator
to specify the sorting order.
- No Duplicates:
- Like other sets,
TreeSet
does not allow duplicate elements. If you attempt to add an element that is already present in the set, it will not be added.
- Balanced Tree:
TreeSet
uses a self-balancing Red-Black tree data structure to store elements. This structure ensures that the set remains balanced, allowing for efficient insertion, deletion, and search operations.
- Null Elements:
TreeSet
does not allownull
elements. If you attempt to add anull
element, it will result in aNullPointerException
.
- Common Methods:
add(E element)
: Adds the specified element to the set if it is not already present.remove(Object o)
: Removes the specified element from the set, if it exists.contains(Object o)
: Checks if the set contains the specified element.size()
: Returns the number of elements in the set.isEmpty()
: Checks if the set is empty.clear()
: Removes all elements from the set.
- NavigableSet:
TreeSet
implements theNavigableSet
interface, which provides navigation methods such asceiling()
,floor()
,higher()
, andlower()
for finding elements based on their values.
- Iterating in Sorted Order:
- You can iterate through the elements in a
TreeSet
to access them in ascending order, which is based on their natural order or the providedComparator
.
- Performance:
- The performance of
TreeSet
operations, such as adding, removing, and searching for elements, is generally O(log n), making it suitable for situations where elements need to be sorted and duplicates are not allowed.
Here’s an example of using TreeSet
:
import java.util.TreeSet;
public class TreeSetExample {
public static void main(String[] args) {
// Creating a TreeSet of integers
TreeSet<Integer> numbers = new TreeSet<>();
// Adding elements
numbers.add(10);
numbers.add(30);
numbers.add(20);
numbers.add(10); // Duplicate, will not be added
// Removing an element
numbers.remove(30);
// Checking for the presence of an element
boolean contains20 = numbers.contains(20); // true
// Iterating through elements (sorted order)
for (int number : numbers) {
System.out.println(number);
}
}
}
TreeSet
is a useful data structure in Java for maintaining a sorted set of elements while preventing duplicates. It is commonly used when elements need to be kept in a specific order, and you want to take advantage of the efficient sorting capabilities provided by the Red-Black tree.