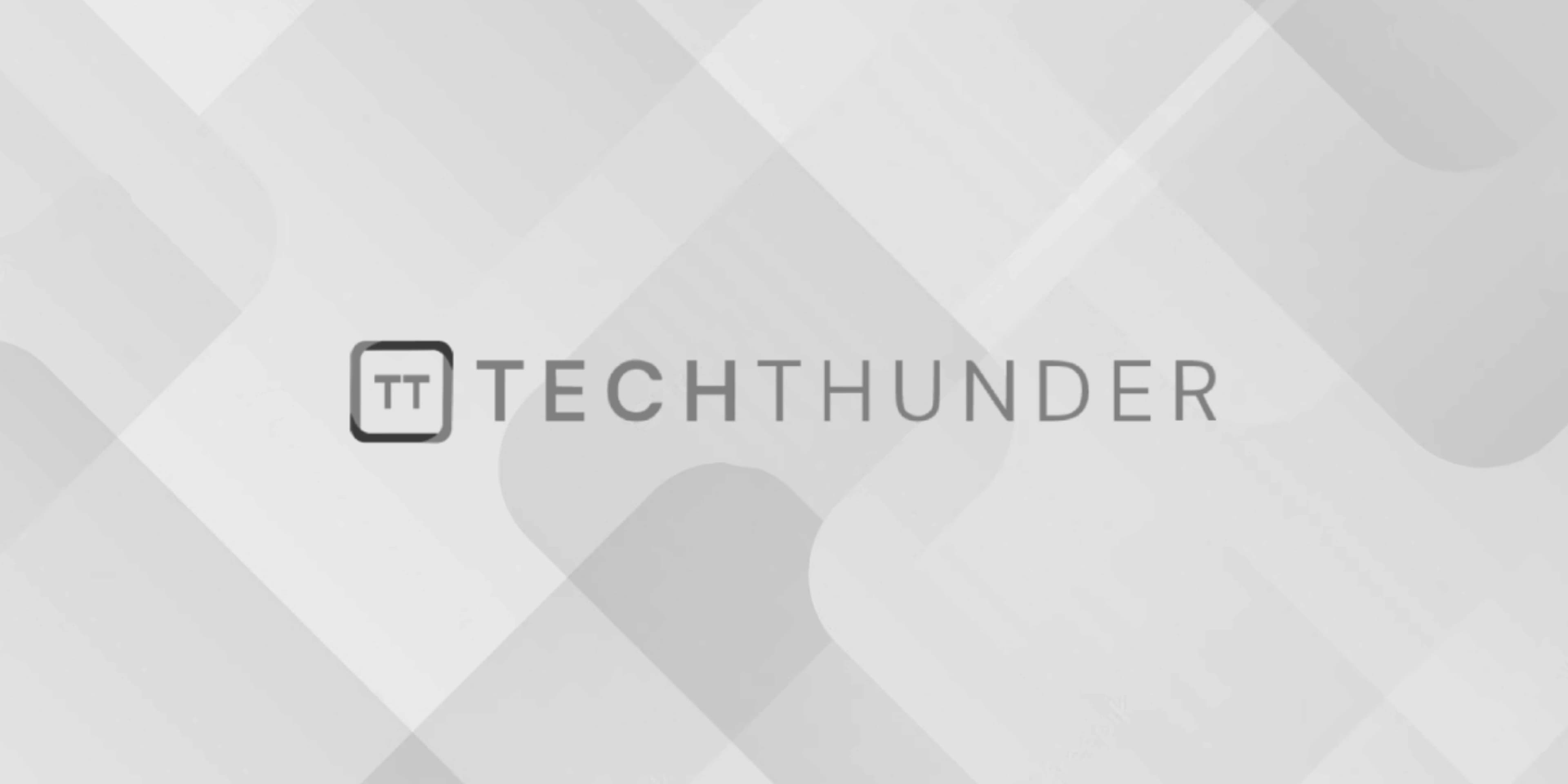
Java Deque & ArrayDeque
The Java has a Deque
(short for “double-ended queue”) is an interface that represents a double-ended queue, which allows elements to be added or removed from both ends. It extends the Queue
interface and provides additional methods to support operations at both ends of the queue. Deque
is part of the Java Collections Framework and is available in the java.util
package.
One of the most commonly used implementations of the Deque
interface is the ArrayDeque
class, which is a resizable-array implementation of a double-ended queue. Here are some important points to understand about Deque
and ArrayDeque
:
- Interface Deque:
Deque
is an interface in Java that defines methods for performing operations like adding, removing, and inspecting elements at both ends of the queue.- It extends the
Queue
interface, so it inherits methods for basic queue operations.
- Class ArrayDeque:
ArrayDeque
is a class in Java that implements theDeque
interface using a resizable-array approach.- It provides constant-time (O(1)) operations for adding and removing elements at both ends, making it efficient for use as a stack or a queue.
- Key Methods:
addFirst(E e)
andaddLast(E e)
: These methods add an element to the beginning and end of the deque, respectively.offerFirst(E e)
andofferLast(E e)
: These methods are similar to the above ones but return a special value (usuallytrue
orfalse
) to indicate if the operation succeeded.removeFirst()
andremoveLast()
: These methods remove and return the first and last elements from the deque, respectively.pollFirst()
andpollLast()
: These methods are similar to the above ones but returnnull
if the deque is empty.getFirst()
andgetLast()
: These methods return, but do not remove, the first and last elements from the deque, respectively.peekFirst()
andpeekLast()
: These methods are similar to the above ones but returnnull
if the deque is empty.
Here’s an example of using ArrayDeque
:
import java.util.ArrayDeque;
import java.util.Deque;
public class ArrayDequeExample {
public static void main(String[] args) {
Deque<String> deque = new ArrayDeque<>();
// Adding elements
deque.addLast("Apple");
deque.addFirst("Banana");
deque.addLast("Cherry");
// Removing and displaying elements
System.out.println(deque.removeFirst()); // Removes Banana
System.out.println(deque.removeLast()); // Removes Cherry
// Peeking at the first and last elements
System.out.println(deque.getFirst()); // Apple
System.out.println(deque.getLast()); // Apple
}
}
Deque
and ArrayDeque
are versatile data structures that can be used in a variety of scenarios to efficiently manage collections of elements with double-ended queue semantics.