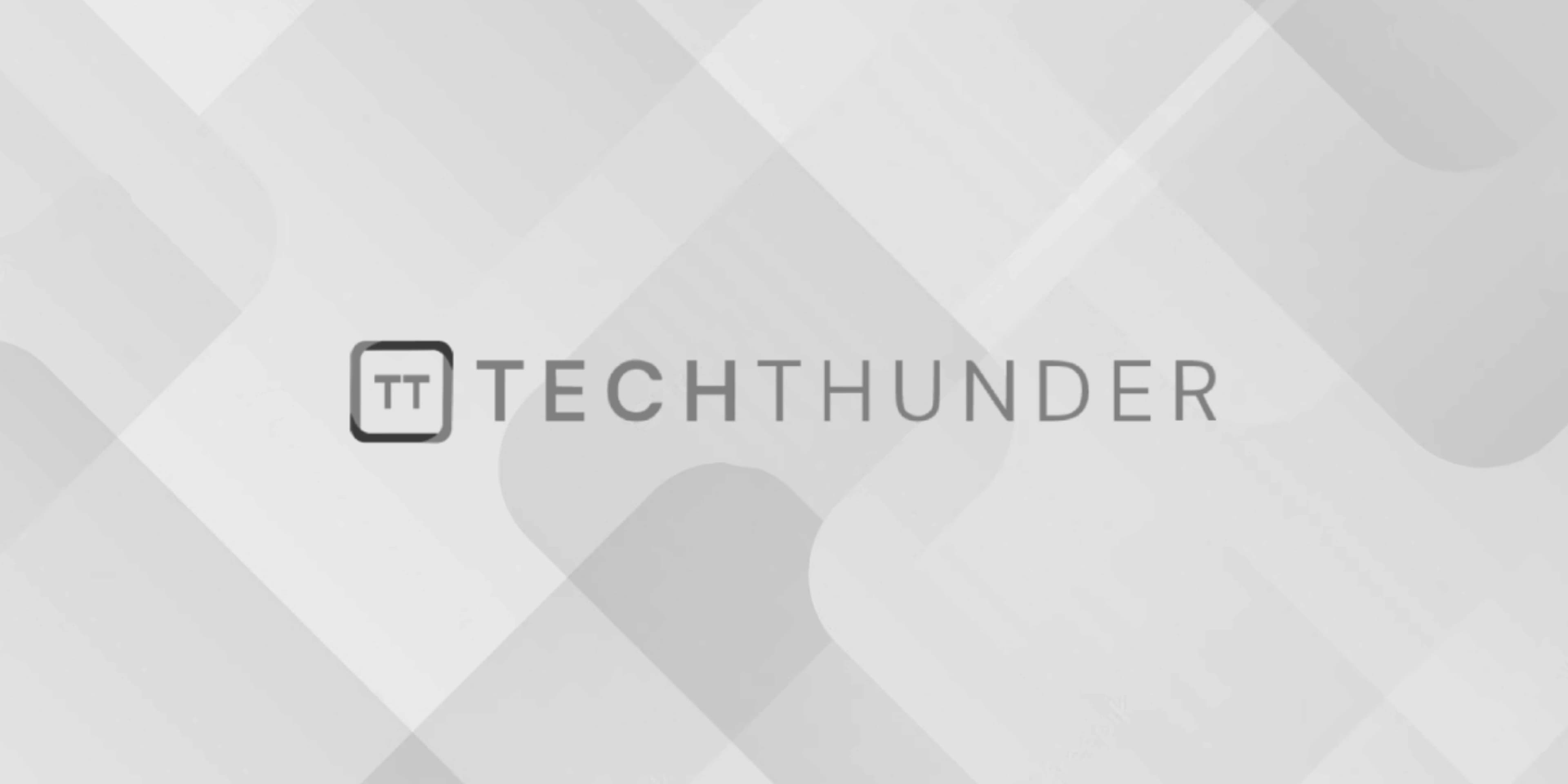
154 views
Java HashMap
The Java HashMap
is a widely used implementation of the Map
interface. It is part of the Java Collections Framework and is located in the java.util
package. HashMap
stores key-value pairs and allows for efficient retrieval, insertion, and removal of elements. Here are some key features and usage guidelines for HashMap
:
- Key-Value Pairs:
HashMap
stores data as key-value pairs, where each key is unique within the map.- Each key is associated with a value.
- Efficient Retrieval:
HashMap
uses a hash table to store and manage its elements, which provides O(1) average-case time complexity for basic operations such asget()
andput()
.
- Null Keys and Values:
HashMap
allows onenull
key and multiplenull
values.- This can be useful in certain situations but requires care to avoid NullPointerExceptions when accessing values.
- Common Methods:
put(K key, V value)
: Associates the specified key with the specified value in the map.get(Object key)
: Retrieves the value associated with the given key.remove(Object key)
: Removes the key-value pair associated with the given key.containsKey(Object key)
: Checks if the map contains the specified key.keySet()
: Returns a set containing all the keys in the map.values()
: Returns a collection of all the values in the map.entrySet()
: Returns a set of key-value pairs (entries).
- Synchronization:
HashMap
is not synchronized by default, which means it’s not thread-safe. If you need thread safety, you can useCollections.synchronizedMap(Map<K, V> map)
to create a synchronized version.
- Iteration:
- You can iterate through the keys or values in a
HashMap
using iterators or enhanced for loops.
Here’s an example of using HashMap
:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Creating a HashMap
Map<String, Integer> ages = new HashMap<>();
// Adding key-value pairs
ages.put("Alice", 25);
ages.put("Bob", 30);
ages.put("Charlie", 35);
// Retrieving values by key
int age = ages.get("Alice");
System.out.println("Alice's age is " + age);
// Checking if a key exists
if (ages.containsKey("David")) {
System.out.println("David's age is " + ages.get("David"));
} else {
System.out.println("David's age is not available.");
}
// Iterating through key-value pairs
for (Map.Entry<String, Integer> entry : ages.entrySet()) {
System.out.println(entry.getKey() + " is " + entry.getValue() + " years old.");
}
}
}
HashMap
is a versatile data structure that is widely used in Java for a variety of purposes, including caching, data indexing, and more. However, it’s important to ensure proper synchronization when used in multi-threaded environments if necessary.