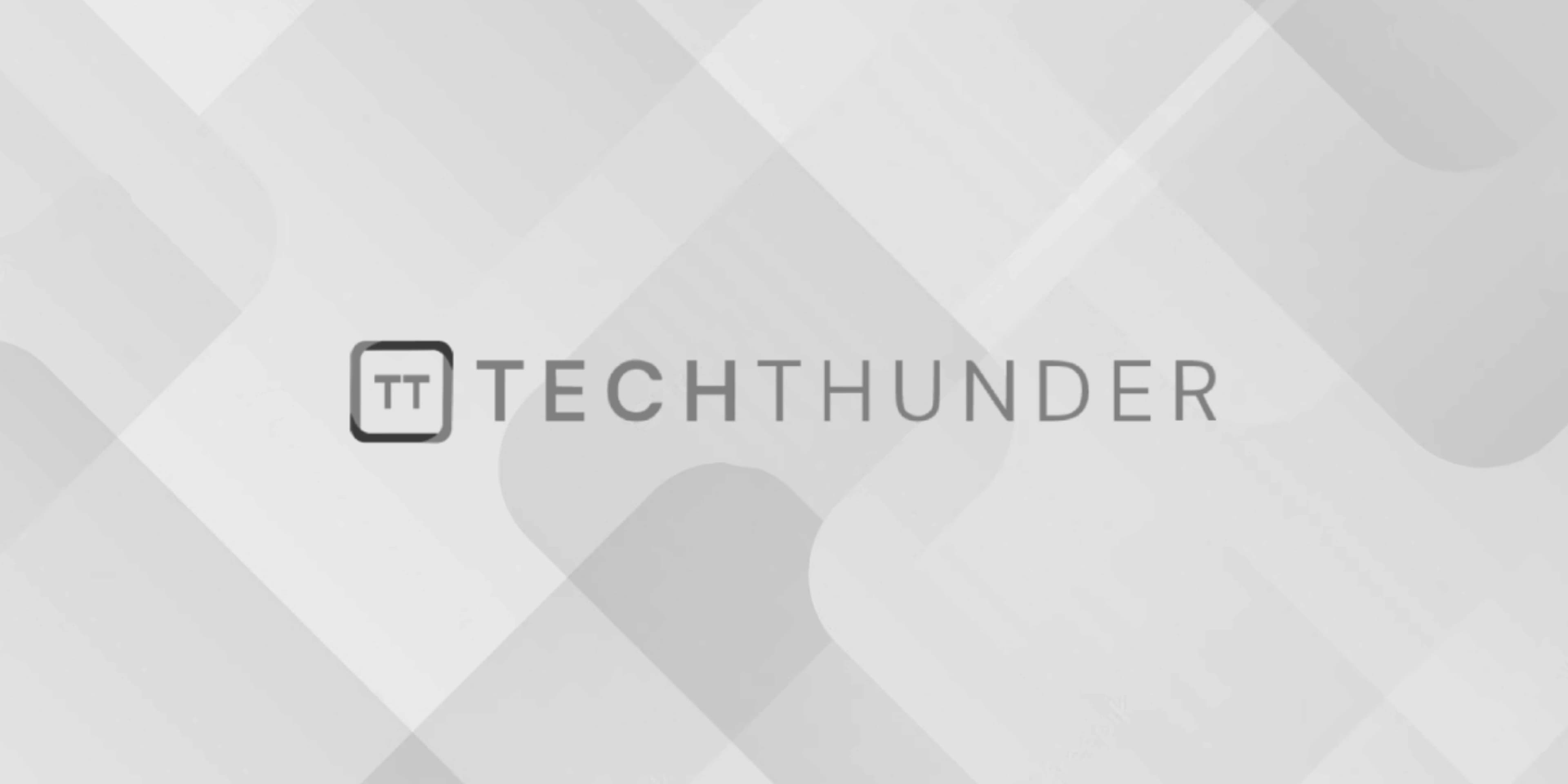
Java LinkedList
The Java LinkedList
is a data structure that implements the List
and Deque
interfaces. It is part of the Java Collections Framework and is provided by the java.util
package. A LinkedList
is a doubly-linked list, which means that each element in the list is connected to both the previous and the next elements. This structure allows for efficient insertion and deletion of elements at both the beginning and the end of the list. Here are some key features and usage guidelines for LinkedList
:
- Doubly-Linked List:
- Each element in a
LinkedList
is represented as a node that contains a reference to the previous and next elements in the list. This structure allows for fast insertions and removals at both ends.
- Efficient Insertions and Deletions:
- Inserting or deleting elements at the beginning or end of a
LinkedList
is an O(1) operation, making it more efficient than anArrayList
for these operations.
- Random Access:
- Unlike
ArrayList
,LinkedList
does not offer efficient random access to elements (e.g.,get(index)
). Accessing elements by index has a time complexity of O(n) as you have to traverse the list from either the beginning or end to reach the desired element.
- Iterating and Traversing:
- You can use iterators or enhanced for loops to iterate through the elements in a
LinkedList
. Forward and backward traversal is equally efficient.
- Common Methods:
add(E element)
: Adds an element to the end of the list.addFirst(E element)
: Adds an element to the beginning of the list.addLast(E element)
: Adds an element to the end of the list.remove()
: Removes and returns the first element in the list.removeFirst()
: Removes and returns the first element in the list.removeLast()
: Removes and returns the last element in the list.size()
: Returns the number of elements in the list.
- Null Elements:
LinkedList
allowsnull
elements.
- Queue Operations:
- Because
LinkedList
implements theDeque
interface, it can be used as a double-ended queue or a queue (FIFO) for managing elements. It provides methods likeoffer()
,poll()
, andpeek()
for queue operations.
Here’s an example of using LinkedList
:
import java.util.LinkedList;
public class LinkedListExample {
public static void main(String[] args) {
// Creating a LinkedList of strings
LinkedList<String> names = new LinkedList<>();
// Adding elements
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Inserting at the beginning
names.addFirst("David");
// Removing and returning the first element
String first = names.removeFirst();
System.out.println("First name: " + first);
// Iterating through elements
for (String name : names) {
System.out.println(name);
}
}
}
LinkedList
is a useful data structure when you need efficient insertions and deletions at both ends and when random access is not a critical requirement. It’s commonly used for implementing queues, stacks, and as a general-purpose list in various Java applications.