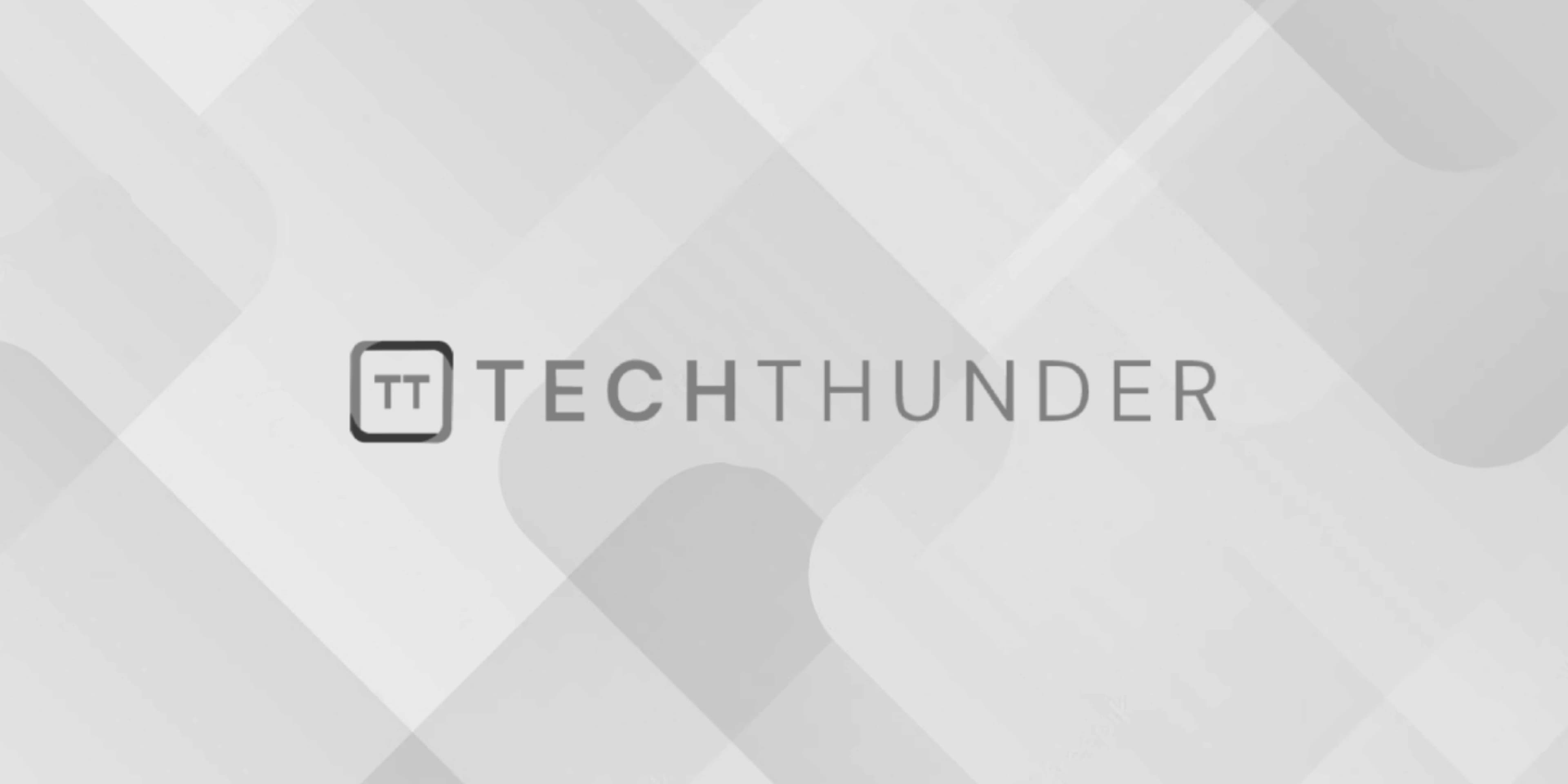
Java Collection Interface
The Collection
interface is a fundamental part of the Java Collections Framework. It serves as the root interface for most of the common collection types in Java, providing a common set of methods that define the basic operations that can be performed on a collection of elements. The Java Collections Framework provides various concrete implementations of the Collection
interface, such as List
, Set
, and Queue
.
Here are some key points about the Collection
interface:
- Basic Methods: The
Collection
interface defines methods that allow you to perform common operations on collections, such as adding, removing, checking for containment, and checking the size. Common methods include:
boolean add(E e)
: Adds an element to the collection.boolean remove(Object o)
: Removes an element from the collection.boolean contains(Object o)
: Checks if an element is present in the collection.int size()
: Returns the number of elements in the collection.
- Iteration: The
Collection
interface provides methods for iterating over its elements using anIterator
or enhanced for loop. Common methods include:
Iterator<E> iterator()
: Returns an iterator over the elements in the collection.forEach(Consumer<? super E> action)
: Applies a given action to each element in the collection.
- Bulk Operations: The
Collection
interface also includes methods for performing bulk operations, such as adding all elements from another collection, checking if one collection is a subset of another, and more. Common methods include:
boolean addAll(Collection<? extends E> c)
: Adds all elements from another collection to this collection.boolean containsAll(Collection<?> c)
: Checks if this collection contains all elements from another collection.
- Empty and Clear: The
isEmpty()
method checks if the collection is empty, and theclear()
method removes all elements from the collection. - Array Conversion: The
toArray()
method allows you to convert the collection into an array. - Different Collection Types: The
Collection
interface is extended by other interfaces likeList
,Set
, andQueue
, which define more specific behaviors for different types of collections. For example,List
maintains ordered elements,Set
enforces uniqueness, andQueue
models a queue data structure.
Here’s a simple example of how you might use the Collection
interface:
import java.util.*;
public class CollectionExample {
public static void main(String[] args) {
Collection<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
System.out.println("Collection size: " + names.size()); // Output: 3
names.remove("Bob");
System.out.println("Is Charlie in the collection? " + names.contains("Charlie")); // Output: true
for (String name : names) {
System.out.println(name);
}
}
}
Keep in mind that the Collection
interface provides a generic abstraction for working with collections of elements, but the specific behavior and characteristics depend on the concrete implementation you choose, such as ArrayList
, HashSet
, and others.