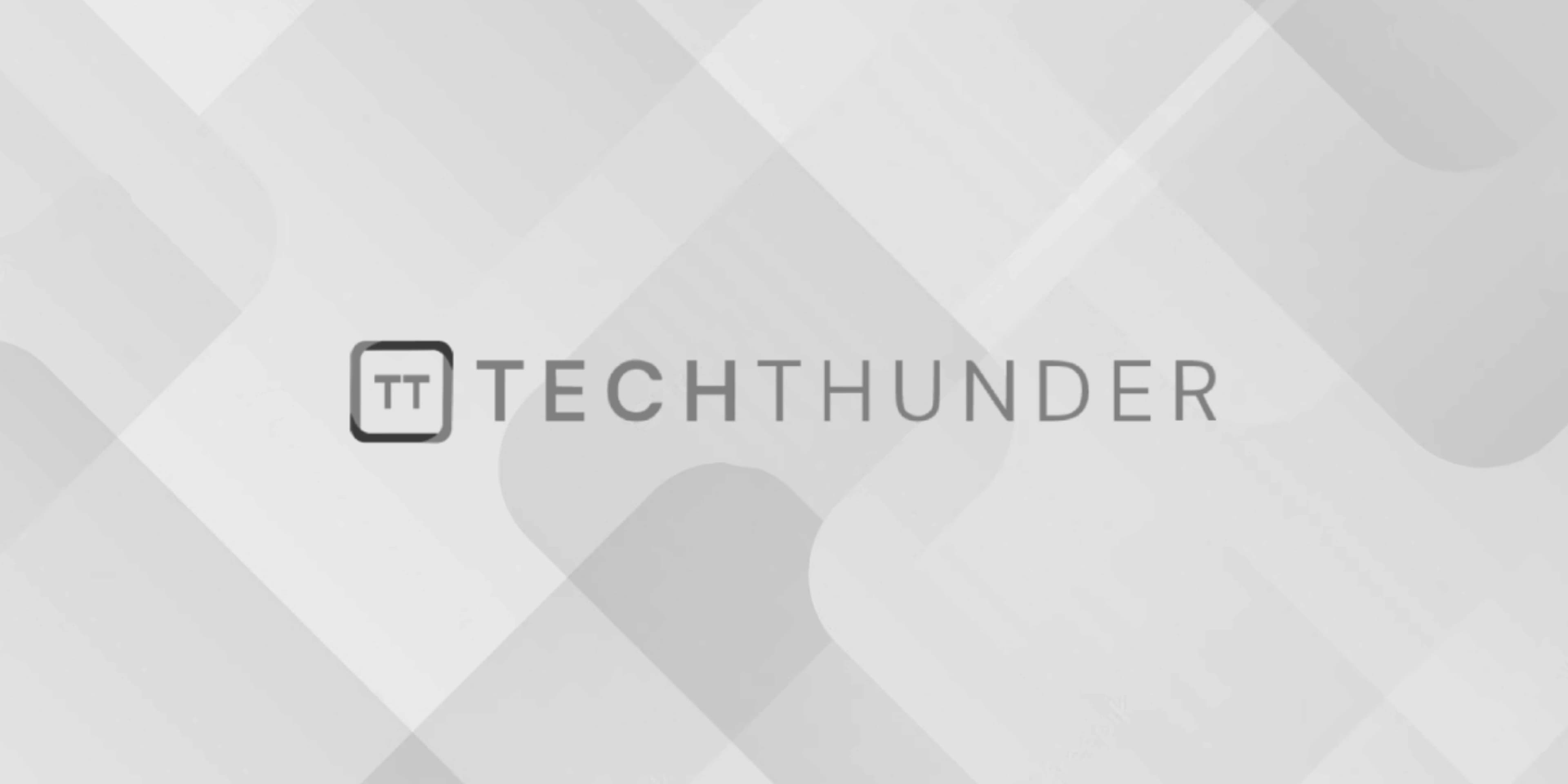
Java LinkedHashSet
The Java LinkedHashSet
is a class that implements the Set
interface and is part of the Java Collections Framework, found in the java.util
package. LinkedHashSet
is an extension of HashSet
with the added feature of maintaining the order in which elements were inserted, while still ensuring that no duplicate elements are allowed. It combines the features of a hash table and a linked list, providing efficient access and predictable iteration order. Here are some key characteristics and usage guidelines for LinkedHashSet
:
- Order Preservation:
LinkedHashSet
maintains the order of elements in the order they were inserted. When you iterate through aLinkedHashSet
, the elements are returned in the order in which they were added.
- No Duplicates:
- Like
HashSet
,LinkedHashSet
does not allow duplicate elements. If you attempt to add an element that already exists in the set, it will not be added, and no exception will be thrown.
- Hash-Based Implementation:
LinkedHashSet
is based on a hash table data structure for storing elements, which allows for efficient operations such as adding and searching for elements.
- Null Elements:
LinkedHashSet
allows a singlenull
element. In other words, you can addnull
to aLinkedHashSet
only once.
- Common Methods:
add(E element)
: Adds the specified element to the set if it is not already present.remove(Object o)
: Removes the specified element from the set, if it exists.contains(Object o)
: Checks if the set contains the specified element.size()
: Returns the number of elements in the set.isEmpty()
: Checks if the set is empty.clear()
: Removes all elements from the set.
- Iteration Order:
- The order of elements in a
LinkedHashSet
is determined by the insertion order. When you iterate through the set, the elements are returned in the order they were added.
- Performance:
- The performance of
LinkedHashSet
operations, such as adding, removing, and searching for elements, is generally constant-time on average (O(1)), making it suitable for most use cases.
Here’s an example of using LinkedHashSet
:
import java.util.LinkedHashSet;
public class LinkedHashSetExample {
public static void main(String[] args) {
// Creating a LinkedHashSet of strings
LinkedHashSet<String> colors = new LinkedHashSet<>();
// Adding elements
colors.add("Red");
colors.add("Green");
colors.add("Blue");
colors.add("Red"); // Duplicate, will not be added
// Removing an element
colors.remove("Green");
// Checking for the presence of an element
boolean containsBlue = colors.contains("Blue"); // true
// Iterating through elements (order is maintained)
for (String color : colors) {
System.out.println(color);
}
}
}
LinkedHashSet
is useful when you need to maintain the insertion order of elements and ensure that no duplicate elements are allowed. It is commonly used in situations where you need predictable iteration order in addition to set semantics.