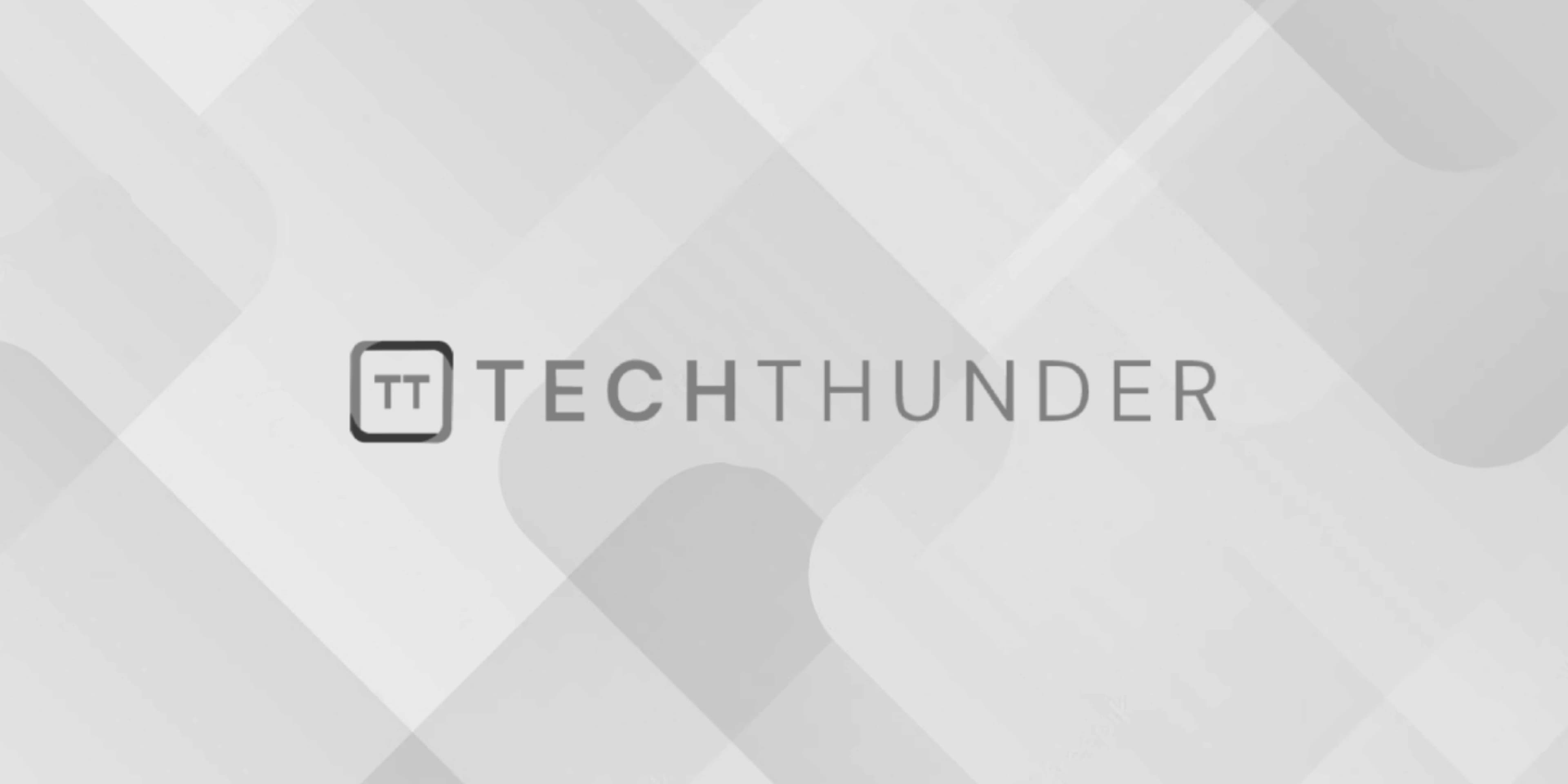
Java Comparable vs Comparator
Comparable
and Comparator
are both Java interfaces used for sorting objects, but they serve slightly different purposes. They provide ways to define custom comparison logic for objects in order to facilitate sorting in various scenarios. Let’s explore the differences between Comparable
and Comparator
:
1. Comparable:
- The
Comparable
interface is implemented by the objects themselves to define their natural ordering. - The
compareTo
method is used to compare the current object with another object. - Objects that implement
Comparable
can be sorted using methods likeCollections.sort()
orArrays.sort()
. - It provides a single way of comparing objects and establishes a default sorting order for the class.
- You can only have one natural ordering for an object.
Example:
public class Student implements Comparable<Student> {
@Override
public int compareTo(Student other) {
// Compare based on some criteria
// Return negative, zero, or positive value
}
}
2. Comparator:
- The
Comparator
interface is implemented separately from the objects being compared. - The
compare
method is used to define custom comparison logic between two objects. Comparator
instances can be used to sort objects of a class without modifying the class itself.- It allows for multiple ways of sorting objects based on different criteria.
- You can define multiple comparators for the same class to enable sorting using various criteria.
Example:
public class StudentComparator implements Comparator<Student> {
@Override
public int compare(Student student1, Student student2) {
// Compare based on some criteria
// Return negative, zero, or positive value
}
}
In summary, the main difference between Comparable
and Comparator
lies in how and where the comparison logic is defined. Use Comparable
when you want to provide a natural ordering for objects and you have control over their class. Use Comparator
when you want to define different sorting criteria for objects or when you can’t modify the class itself.
Remember that if a class implements Comparable
, you can still use a Comparator
to sort its instances in ways other than their natural order. This provides greater flexibility when working with sorting and allows you to adapt to different requirements without modifying existing code.