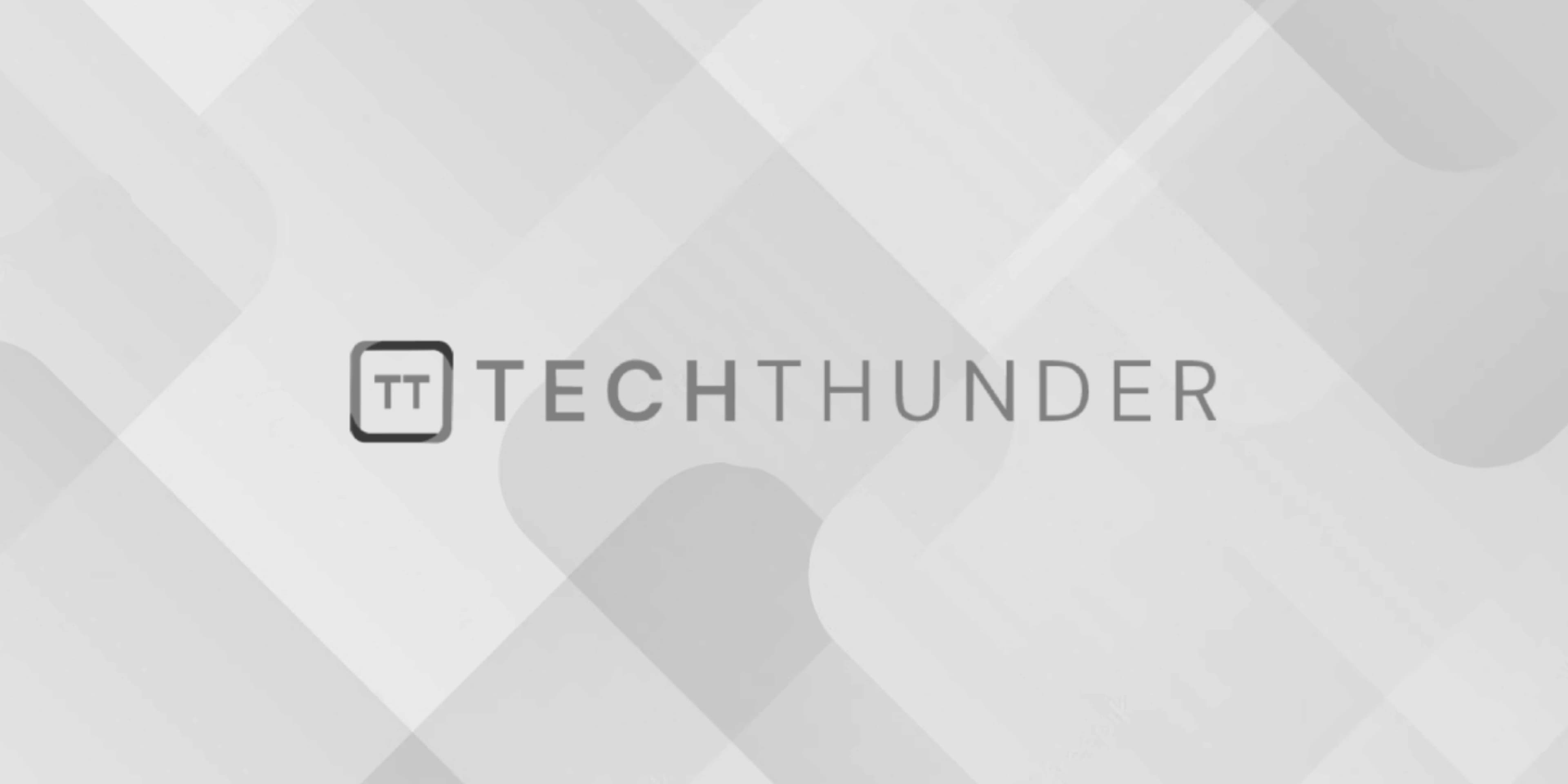
Java Sorting Collections
The sort collections in Java using the java.util.Collections
class or the java.util.Arrays
class, depending on whether you’re dealing with lists or arrays. The sorting is typically done using the natural ordering of the elements or a custom comparator.
Here’s how you can sort collections using the Collections
class:
import java.util.*;
public class SortingCollectionsExample {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.add(5);
numbers.add(2);
numbers.add(8);
numbers.add(1);
Collections.sort(numbers); // Sort in natural order
for (int num : numbers) {
System.out.println(num);
}
}
}
You can also provide a custom comparator to sort based on specific criteria:
import java.util.*;
public class SortingCollectionsCustomComparatorExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
names.add("Eve");
// Sort based on string length
Collections.sort(names, Comparator.comparingInt(String::length));
for (String name : names) {
System.out.println(name);
}
}
}
For sorting arrays, you can use the Arrays
class:
import java.util.*;
public class SortingArraysExample {
public static void main(String[] args) {
int[] numbers = { 5, 2, 8, 1 };
Arrays.sort(numbers);
for (int num : numbers) {
System.out.println(num);
}
}
}
Remember that the sorting methods in both Collections
and Arrays
use the natural ordering by default. If you need a custom ordering, you can provide a comparator as an argument to the sorting methods.
Keep in mind that when sorting objects, they need to implement the Comparable
interface if you’re using the natural ordering, or you need to provide a custom comparator. Also, note that sorting is a potentially expensive operation and may involve different sorting algorithms, so the performance can vary based on the collection size and the chosen sorting algorithm.