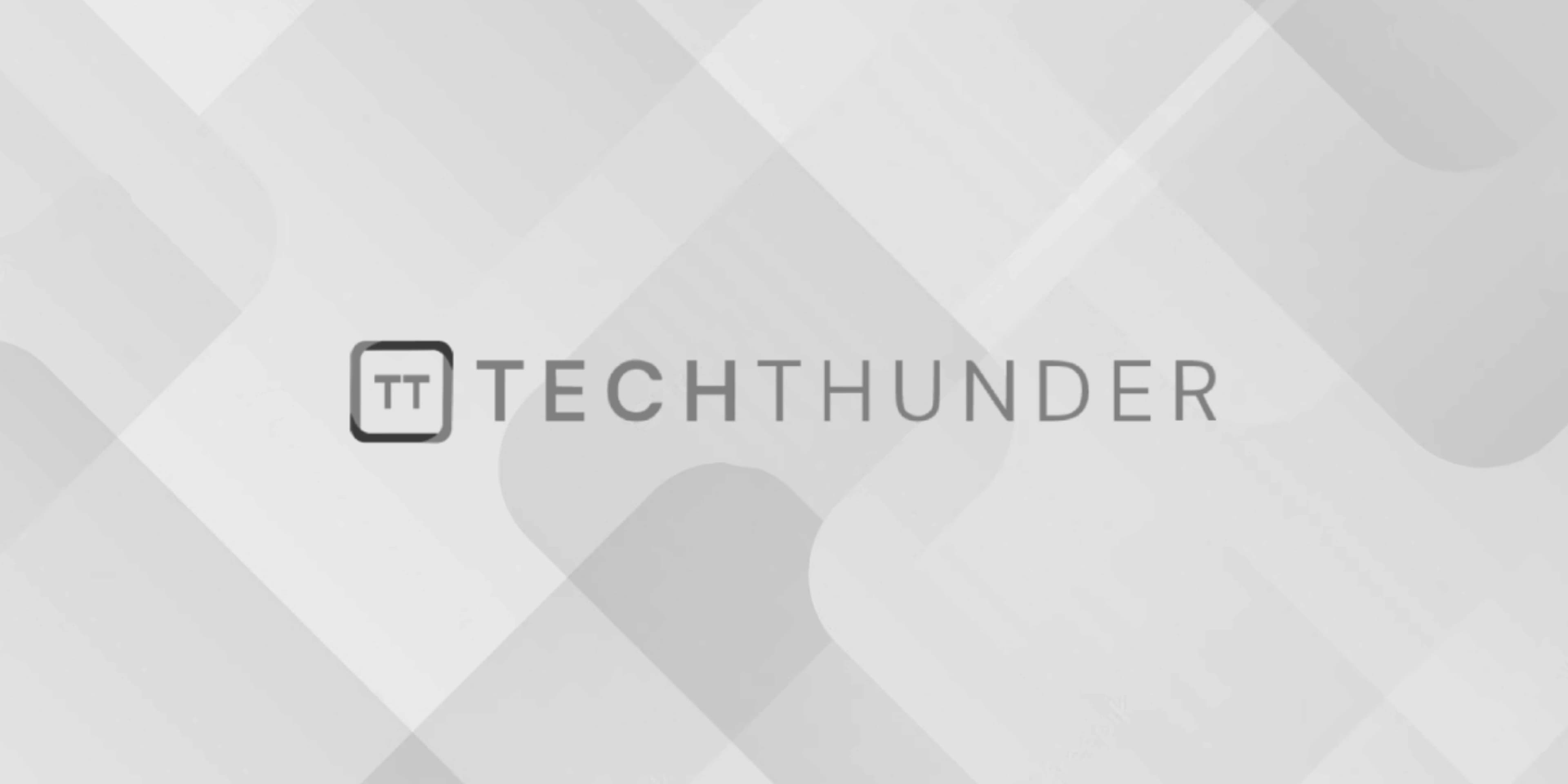
Java Vector
Vector
is a class in the Java Collections Framework that provides a dynamic array-like structure that can hold elements of any data type. It is part of the Java Standard Library and was one of the earliest collection classes introduced in Java.
Here are some key features of the Vector
class:
- Dynamic Array: Like an array, a
Vector
can store elements in an ordered sequence. Unlike arrays, however, aVector
can dynamically resize itself to accommodate more elements as needed. - Synchronization:
Vector
is synchronized by default. This means that its methods are synchronized, making it thread-safe for concurrent access by multiple threads. This also means that there might be performance overhead due to synchronization, especially in single-threaded scenarios. - Legacy Class:
Vector
is one of the older collection classes in Java and was introduced in the early versions of the language. It predates the introduction of the more modernArrayList
class, which is similar in functionality but lacks the synchronization overhead ofVector
. - Enumeration:
Vector
provides an enumeration for iterating through its elements using theEnumeration
interface. - Deprecated Methods: Some methods in
Vector
have been deprecated in favor of newer collection classes and the enhanced for loop introduced in later Java versions.
Here’s a simple example of how you might use the Vector
class:
import java.util.*;
public class VectorExample {
public static void main(String[] args) {
Vector<String> fruits = new Vector<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
System.out.println("Size: " + fruits.size());
Enumeration<String> enumeration = fruits.elements();
while (enumeration.hasMoreElements()) {
System.out.println(enumeration.nextElement());
}
}
}
While Vector
is still available and can be used, it’s worth considering other collection classes provided in the Java Collections Framework and the java.util.concurrent
package, which offer more advanced features and better performance in many scenarios. If you need synchronization, you might also consider using other thread-safe collection classes that provide more fine-grained synchronization and improved performance compared to the default synchronization in Vector
.