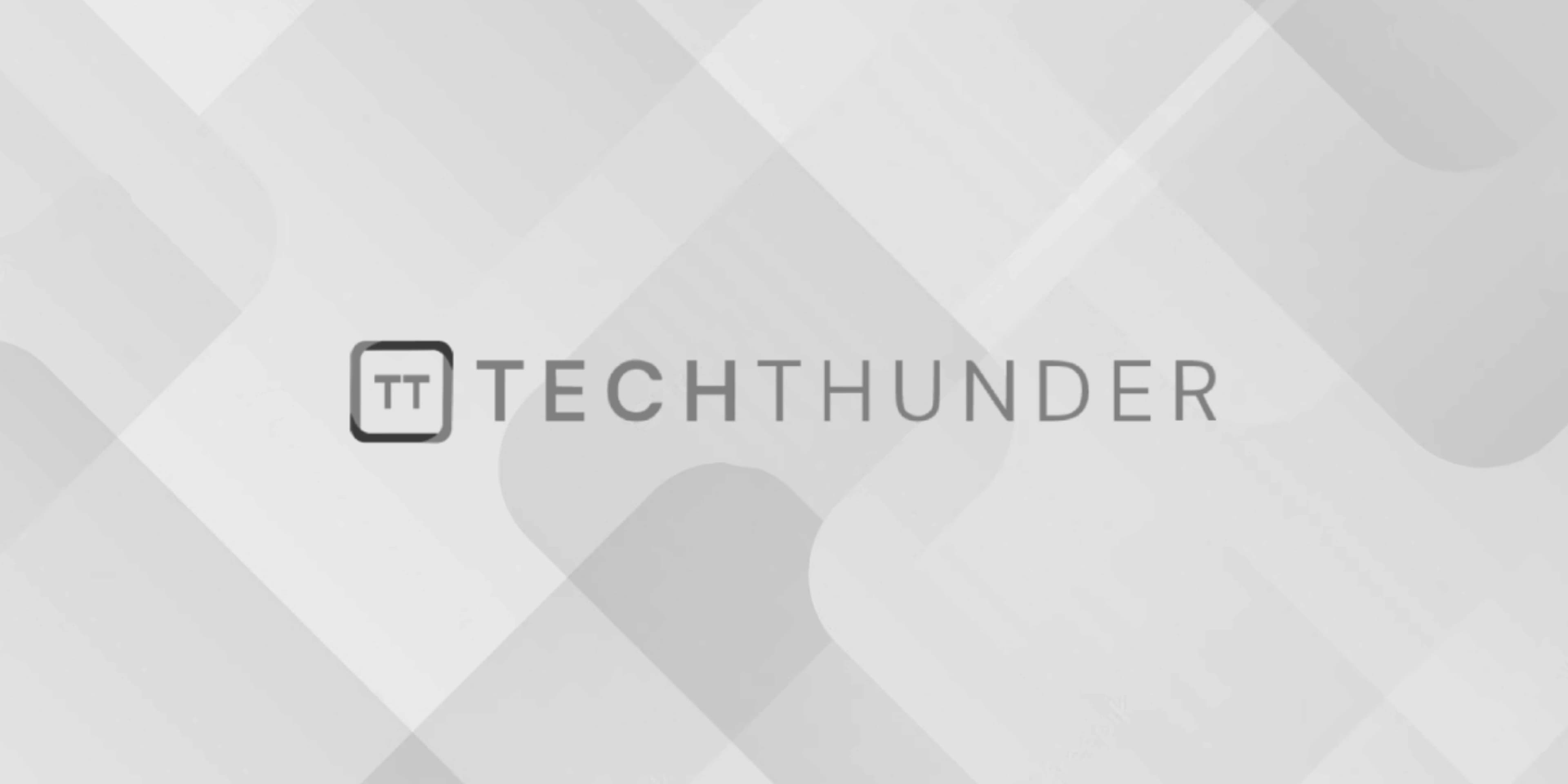
Java EnumSet
EnumSet
is a specialized and efficient implementation of the Set
interface in Java, designed to work specifically with enumerated types (enums). It is part of the Java Collections Framework and provides a way to represent and manipulate a set of elements where each element is an enum constant. Enum sets are highly efficient and have some advantages over general-purpose sets when working with enums. Here are some key points about EnumSet
:
- Restricted to Enum Elements:
EnumSet
is designed to work only with enumerated types (enums). It can store a set of elements, and each element must be one of the enum constants of the specified enum type.
- Efficiency:
EnumSet
is highly efficient and optimized for memory usage and performance. It is implemented as a bit vector, which makes it compact and fast for set operations.
- Type Safe:
- Because it’s restricted to enum elements,
EnumSet
is type-safe. You can’t accidentally add an element of the wrong type to the set.
- Creation:
- You can create an
EnumSet
using various static factory methods such asEnumSet.of()
,EnumSet.noneOf()
, andEnumSet.allOf()
. - Example of creating an
EnumSet
:
EnumSet<Day> daysOff = EnumSet.of(Day.SATURDAY, Day.SUNDAY);
- Methods:
EnumSet
provides methods for set operations likeadd()
,remove()
,contains()
,isEmpty()
,size()
, and more.
- Range Operations:
- You can perform range-based operations using
EnumSet
by specifying a starting and ending enum constant. For example:
EnumSet<Day> weekdays = EnumSet.range(Day.MONDAY, Day.FRIDAY);
- Iteration Order:
EnumSet
maintains the natural iteration order of elements based on their ordinal values. This can be useful when iterating through the elements.
- No Null Elements:
EnumSet
does not allownull
elements. If you attempt to add anull
element, it will throw aNullPointerException
.
Here’s an example of using EnumSet
:
import java.util.EnumSet;
public class EnumSetExample {
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public static void main(String[] args) {
EnumSet<Day> workDays = EnumSet.range(Day.MONDAY, Day.FRIDAY);
EnumSet<Day> weekend = EnumSet.of(Day.SATURDAY, Day.SUNDAY);
System.out.println("Workdays: " + workDays);
System.out.println("Weekend: " + weekend);
// Adding an additional day
workDays.add(Day.SATURDAY);
System.out.println("Workdays (after adding Saturday): " + workDays);
System.out.println("Is Sunday a workday? " + workDays.contains(Day.SUNDAY));
}
}
In this example, we create two EnumSet
instances to represent workdays and weekends. We add a day to the workdays set and check if Sunday is considered a workday using the contains()
method. EnumSet
is a powerful and efficient way to work with enums and sets of enum constants.