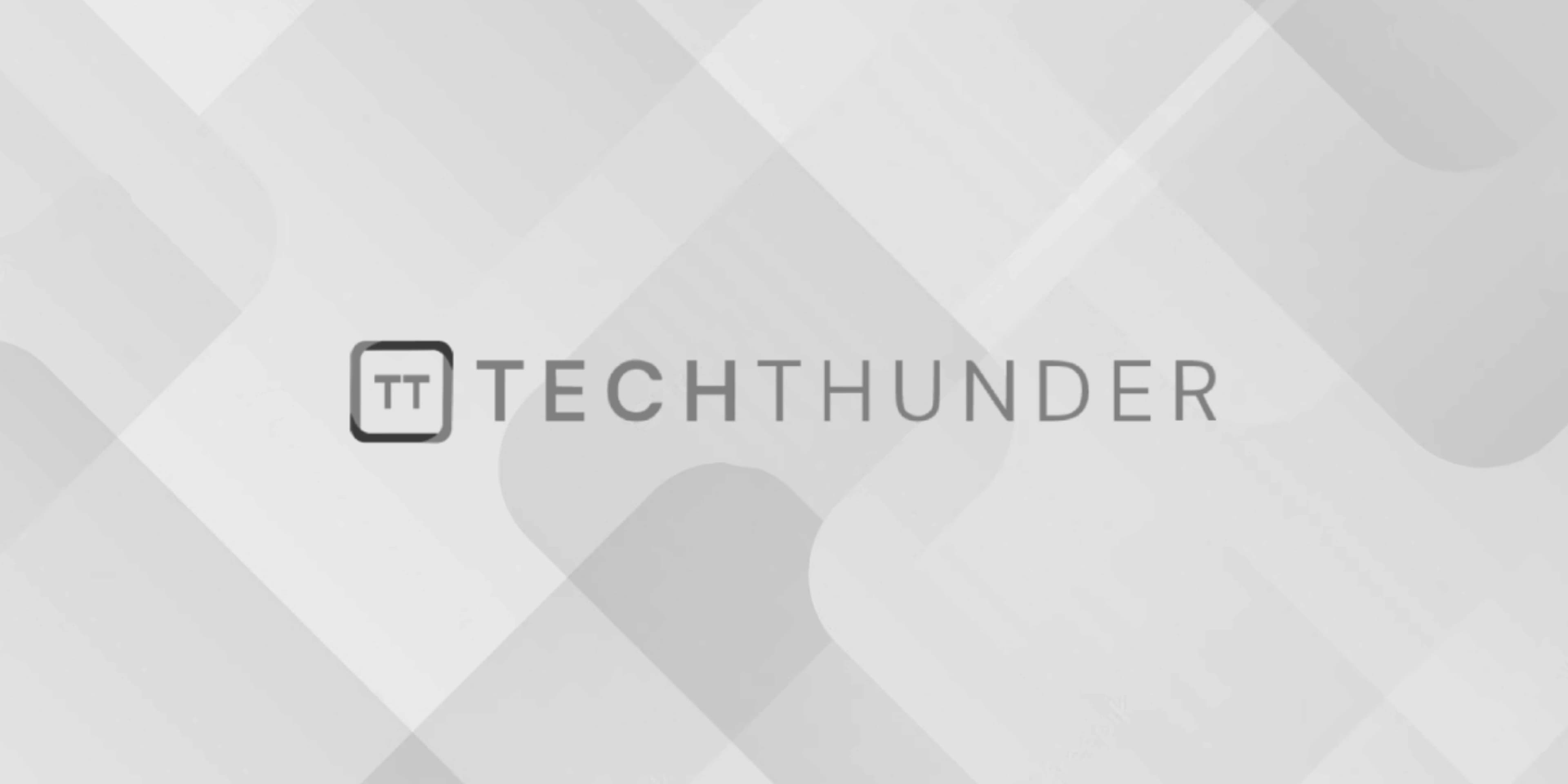
Java ConcurrentLinkedQueue
ConcurrentLinkedQueue
is a class in the Java Collections Framework that provides a highly efficient and thread-safe implementation of a non-blocking, unbounded queue. It is designed for scenarios where multiple threads need to perform enqueue (addition) and dequeue (removal) operations concurrently without explicit synchronization. This class is part of the java.util.concurrent
package introduced in Java 5.
Key features and characteristics of ConcurrentLinkedQueue
include:
- Non-Blocking:
ConcurrentLinkedQueue
is non-blocking, meaning that it doesn’t use locks or synchronized blocks for its operations. Instead, it employs lock-free algorithms to allow multiple threads to operate on the queue concurrently. - Thread-Safe Operations: All basic operations, such as
add
,poll
,peek
, etc., are thread-safe. Multiple threads can safely add and remove elements from the queue concurrently without causing data corruption. - Unbounded Size: Unlike some other queue implementations,
ConcurrentLinkedQueue
is unbounded. This means it can grow dynamically to accommodate any number of elements. - FIFO (First-In-First-Out) Order: The queue follows a FIFO order, meaning that elements are removed in the same order they were added.
- Iterators: Iterating over a
ConcurrentLinkedQueue
using its iterator provides a snapshot view of the data. The iterator is not affected by concurrent modifications made by other threads.
Here’s an example of how you might use ConcurrentLinkedQueue
:
import java.util.concurrent.*;
public class ConcurrentLinkedQueueExample {
public static void main(String[] args) {
ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
queue.add("First");
queue.add("Second");
queue.add("Third");
// Thread-safe operations
String firstElement = queue.peek();
String removedElement = queue.poll();
// Iterate over the queue
for (String element : queue) {
System.out.println(element);
}
}
}
ConcurrentLinkedQueue
is particularly useful in scenarios where multiple threads need to work with a shared queue without blocking each other. It’s important to note that while ConcurrentLinkedQueue
is well-suited for certain use cases, it might not be the best choice for all scenarios. Depending on your application’s specific requirements, you might need to consider other concurrent data structures or mechanisms provided by the Java java.util.concurrent
package.