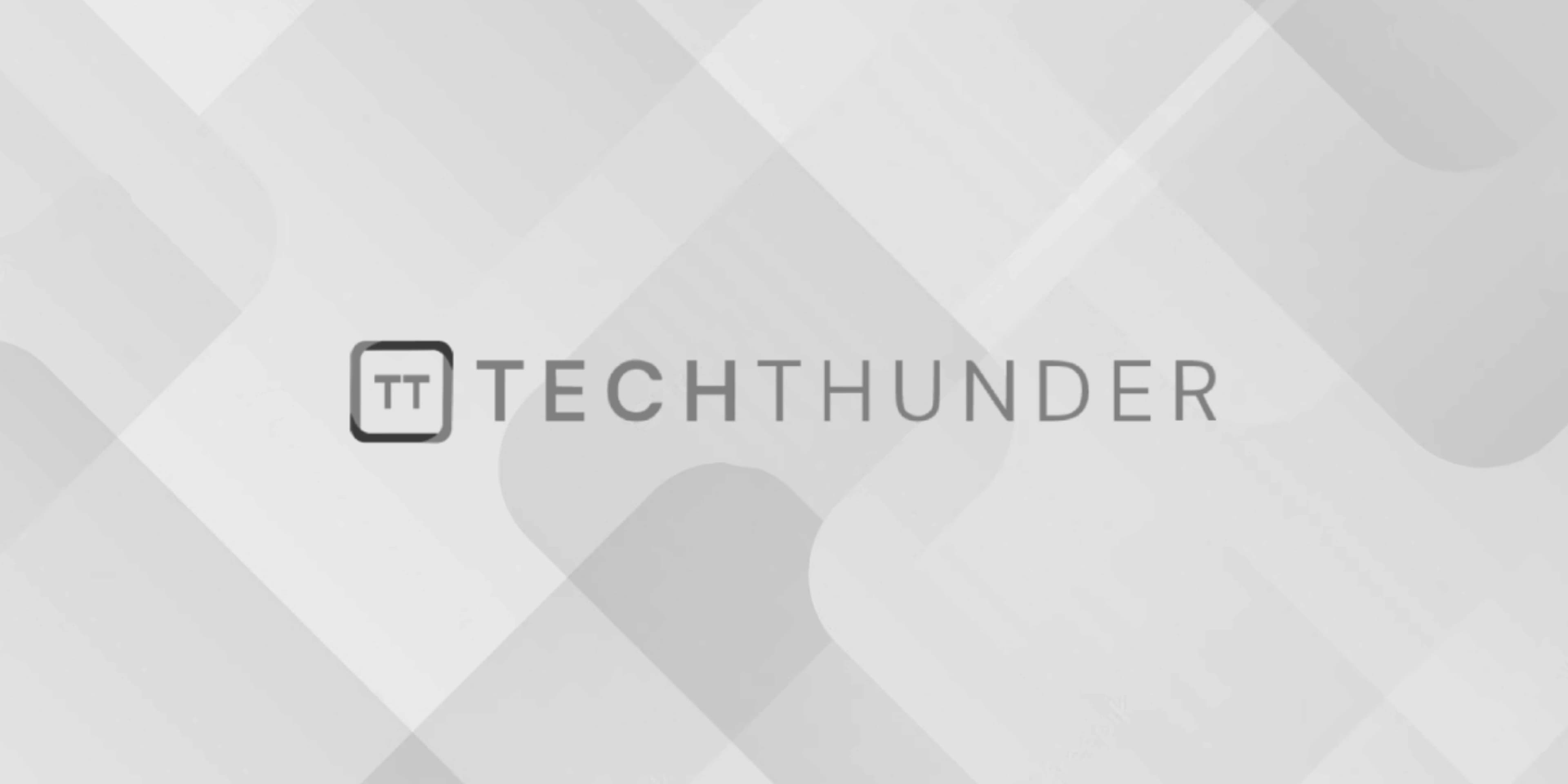
Java HashSet
The Java HashSet
is an implementation of the Set
interface and is part of the Java Collections Framework, found in the java.util
package. It is a collection that does not allow duplicate elements and does not guarantee the order of elements. HashSet
is based on a hash table data structure and provides efficient add, remove, and search operations. Here are some key characteristics and usage guidelines for HashSet
:
- No Duplicates:
- A
HashSet
does not allow duplicate elements. If you attempt to add an element that already exists in the set, it will not be added, and no exception will be thrown.
- Ordering:
- The elements in a
HashSet
are not stored in any specific order. The order in which elements are stored and retrieved may not be the same.
- Hash-Based Implementation:
HashSet
uses a hash table data structure to store its elements. This allows for efficient operations such as adding and searching for elements.
- Null Elements:
HashSet
allows a singlenull
element. In other words, you can addnull
to aHashSet
only once.
- Common Methods:
add(E element)
: Adds the specified element to the set if it is not already present.remove(Object o)
: Removes the specified element from the set, if it exists.contains(Object o)
: Checks if the set contains the specified element.size()
: Returns the number of elements in the set.isEmpty()
: Checks if the set is empty.clear()
: Removes all elements from the set.
- Iteration:
- You can iterate through the elements in a
HashSet
using enhanced for loops or iterators.
- Performance:
- The performance of
HashSet
operations, such as adding, removing, and searching for elements, is generally constant-time on average (O(1)), making it suitable for most use cases.
Here’s an example of using HashSet
:
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
// Creating a HashSet of strings
HashSet<String> colors = new HashSet<>();
// Adding elements
colors.add("Red");
colors.add("Green");
colors.add("Blue");
colors.add("Red"); // Duplicate, will not be added
// Removing an element
colors.remove("Green");
// Checking for the presence of an element
boolean containsBlue = colors.contains("Blue"); // true
// Iterating through elements
for (String color : colors) {
System.out.println(color);
}
}
}
HashSet
is a widely used data structure in Java for situations where you need a collection of elements with no duplicates and where the order of elements does not matter. If you need to maintain the order of elements or allow duplicates, you may consider using LinkedHashSet
or other collection classes such as ArrayList
or LinkedList
.