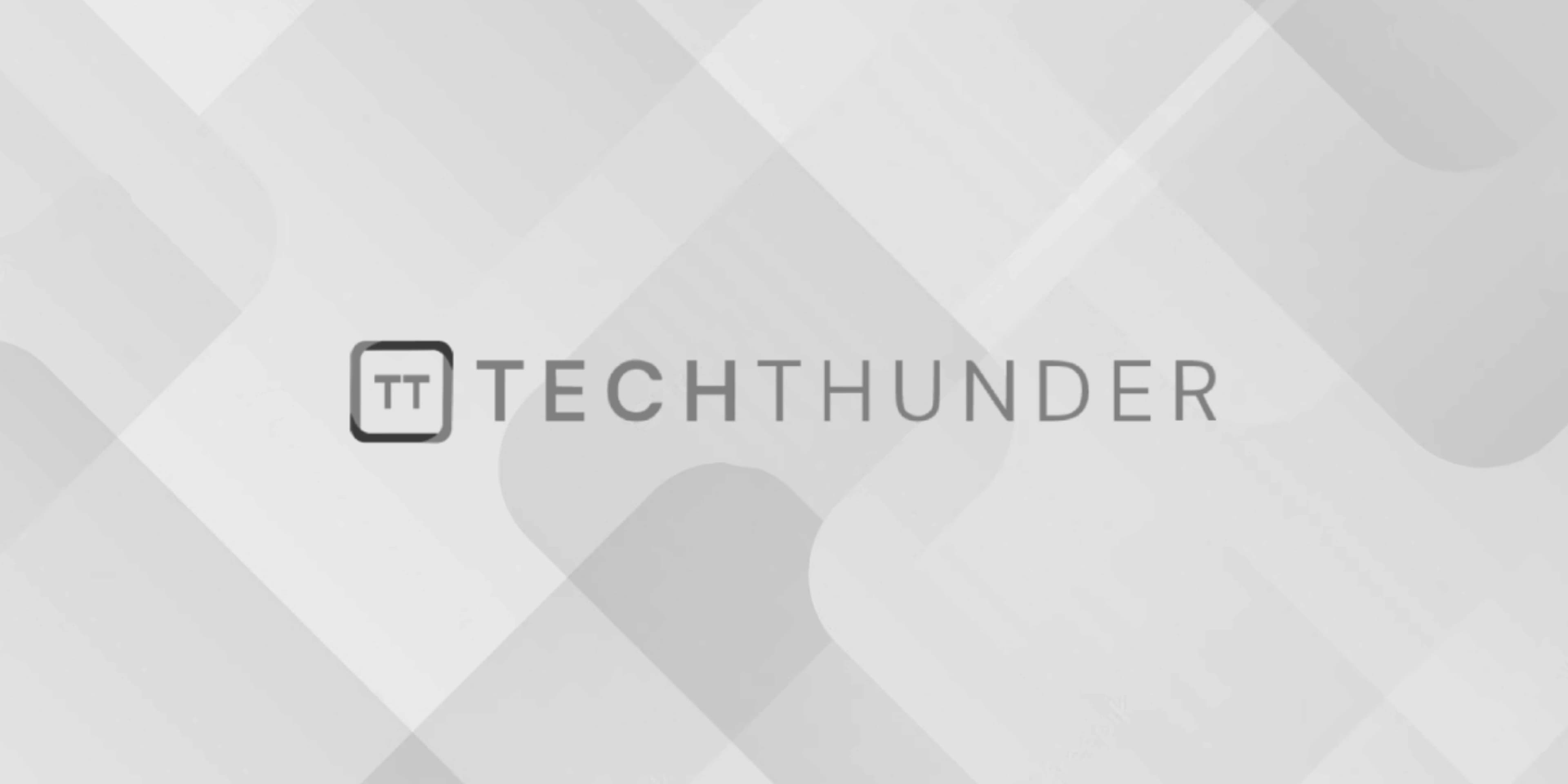
Java TreeMap
The TreeMap
class in Java is a part of the Java Collections Framework and implements the NavigableMap
interface. It is a sorted map that uses a red-black tree as its underlying data structure. This tree-based structure allows TreeMap
to maintain its elements in a sorted order based on their natural ordering or a custom comparator.
Here are some key features of the TreeMap
class:
- Sorted Order:
TreeMap
maintains its elements in sorted order based on either their natural ordering (if they implementComparable
) or a custom comparator provided when creating the map. - Balanced Tree Structure: The underlying red-black tree structure ensures that the operations (addition, deletion, lookup) are performed in O(log n) time, making it efficient for large datasets.
- Navigational Methods:
TreeMap
provides various methods for navigating the map, such as finding the next or previous element relative to a given key. - Custom Ordering: You can specify a custom comparator to define the sorting order of elements that do not implement
Comparable
. - Submaps:
TreeMap
allows you to create submaps based on a range of keys, which can be useful for working with a specific subset of the map’s keys. - Thread-Safety: Like most standard collections,
TreeMap
is not thread-safe by default. If you need thread-safe behavior, you can useCollections.synchronizedSortedMap()
to obtain a synchronized version ofTreeMap
.
Here’s a simple example of how you might use the TreeMap
class:
import java.util.*;
public class TreeMapExample {
public static void main(String[] args) {
TreeMap<Integer, String> treeMap = new TreeMap<>();
treeMap.put(3, "Three");
treeMap.put(1, "One");
treeMap.put(2, "Two");
// Iterate through the map in sorted order of keys
for (Map.Entry<Integer, String> entry : treeMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
// Finding the next key
System.out.println("Next key after 2: " + treeMap.higherKey(2)); // Output: 3
}
}
In this example, the TreeMap
maintains the elements in sorted order of their keys. The higherKey()
method is used to find the next key after a given key.
Keep in mind that while TreeMap
is a useful data structure for maintaining a sorted map, it has a slight performance overhead due to the underlying tree structure. If you don’t require sorted order and just need efficient key-value lookups, you might consider using HashMap
or LinkedHashMap
.