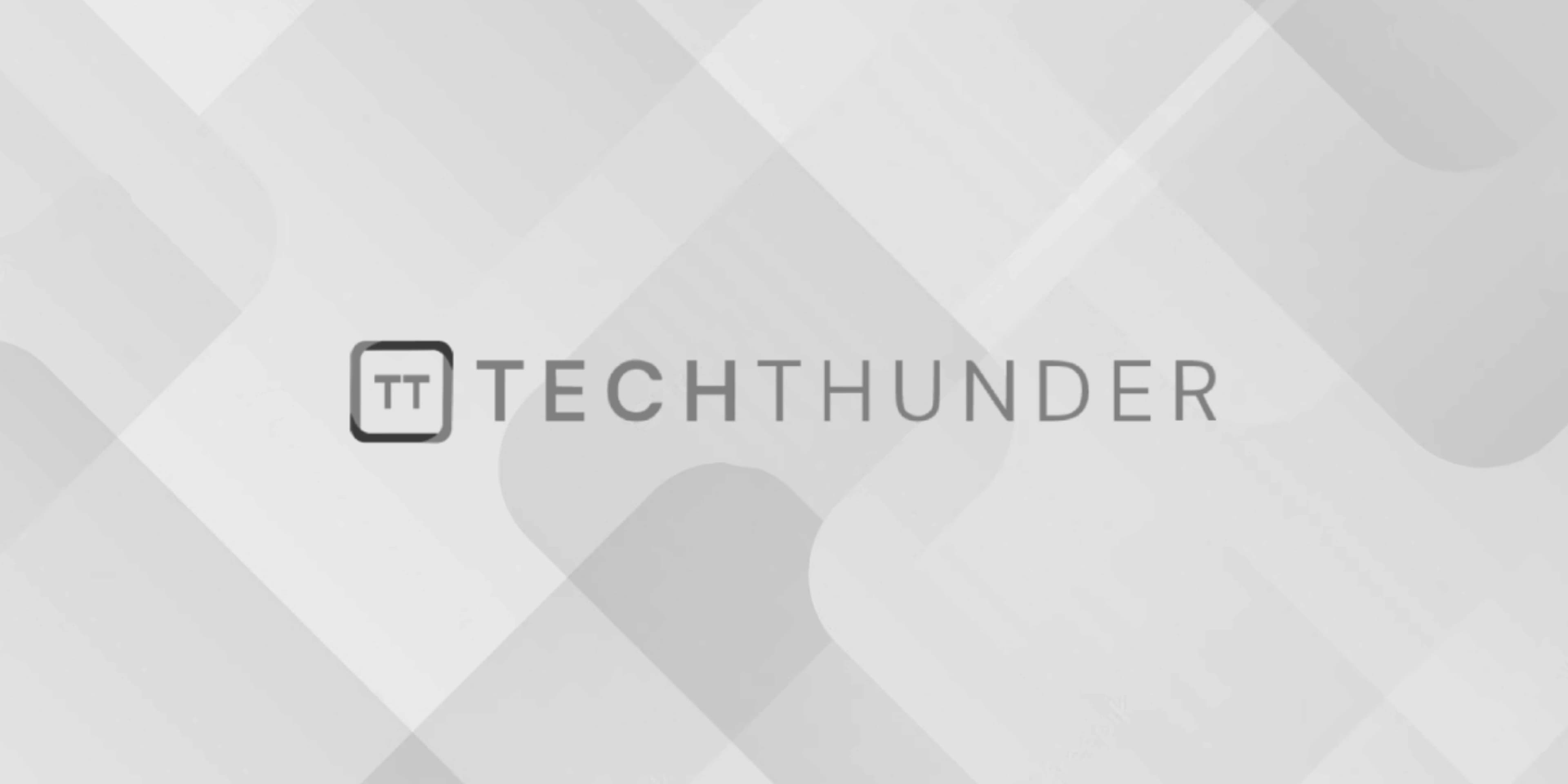
Java Properties class
The java.util.Properties
class in Java is a part of the Java Standard Library and is used to manage and manipulate configuration or settings information in key-value pairs. It is commonly used to load and save configuration data from files or other data sources.
The Properties
class extends the Hashtable
class and provides some additional methods for working specifically with key-value pairs in the context of configuration. Each key and value in a Properties
object is a String
, and they are usually used to represent properties of a system or application.
Here are some key features and methods of the Properties
class:
- Loading Properties from Files:
You can load properties from a file using methods likeload(InputStream)
orload(Reader)
. The properties file should be in a key=value format. - Storing Properties to Files:
You can store properties to a file using methods likestore(OutputStream, String)
orstore(Writer, String)
. This allows you to save configuration changes back to the file. - Getting and Setting Properties:
You can get property values using thegetProperty(String key)
method. If a property doesn’t exist, you can provide a default value. You can set properties using thesetProperty(String key, String value)
method. - Enumeration of Properties:
You can obtain an enumeration of all keys using thepropertyNames()
method, which allows you to iterate through the keys and retrieve their values.
Here’s a simple example of using the Properties
class to load and store configuration data:
import java.io.*;
import java.util.Properties;
public class PropertiesExample {
public static void main(String[] args) {
Properties properties = new Properties();
try (InputStream input = new FileInputStream("config.properties")) {
properties.load(input);
// Getting property values
String username = properties.getProperty("username");
String password = properties.getProperty("password");
System.out.println("Username: " + username);
System.out.println("Password: " + password);
} catch (IOException e) {
e.printStackTrace();
}
try (OutputStream output = new FileOutputStream("config.properties")) {
// Setting property values
properties.setProperty("username", "myusername");
properties.setProperty("password", "mypassword");
properties.store(output, "Updated configuration");
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, the Properties
class is used to load and store configuration data from a properties file named “config.properties”. The load
method is used to read properties from the file, and the store
method is used to update and save properties back to the file.
Keep in mind that while Properties
is a straightforward way to manage configuration data, for more complex scenarios, you might consider using more advanced configuration management libraries.