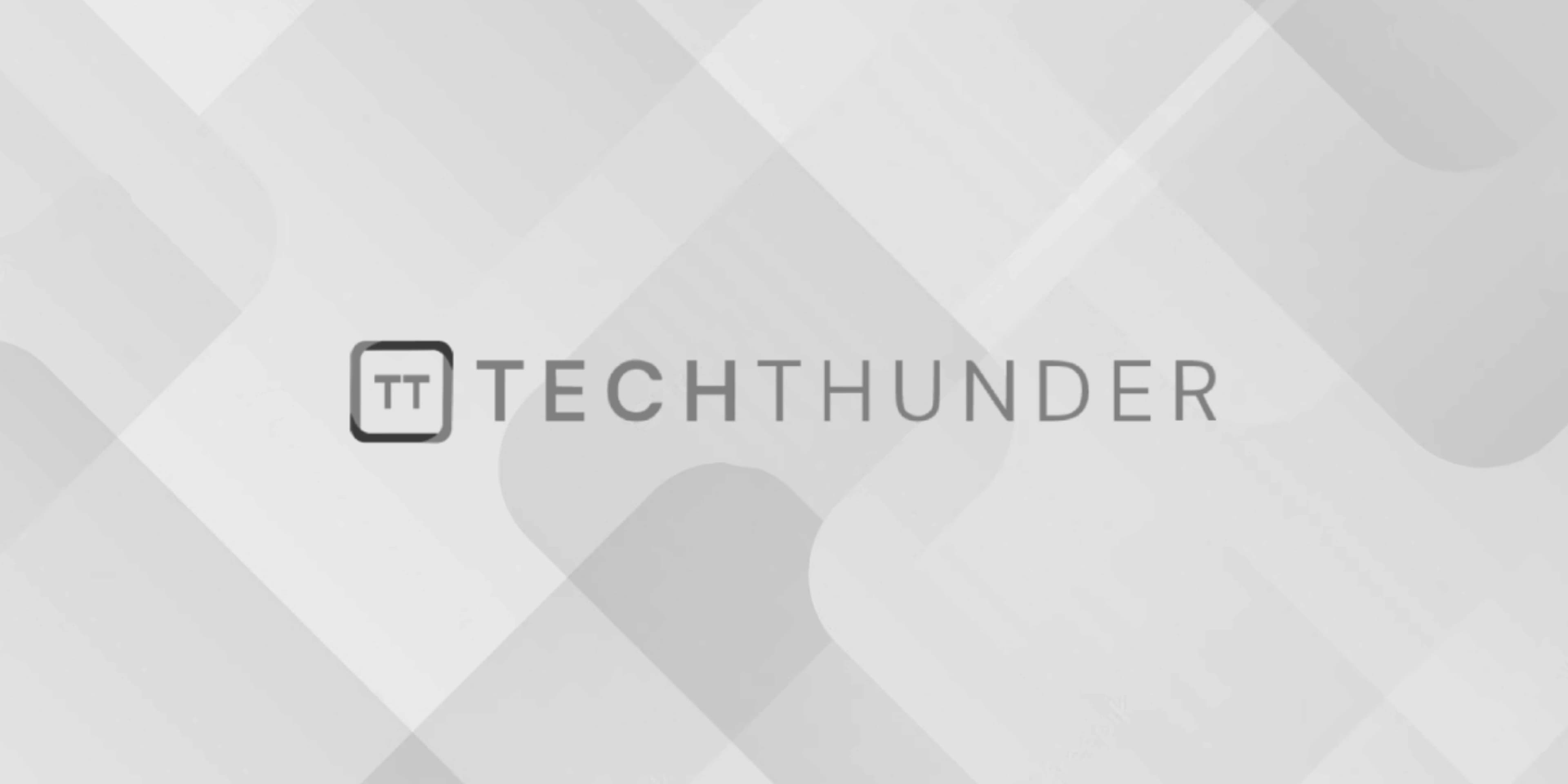
219 views
Java Hashtable
The Java Hashtable
is a legacy class that implements the Map
interface. It provides a way to store and manage key-value pairs similar to a HashMap
, but Hashtable
has some distinct characteristics and differences, including its synchronization and null value handling. Here are key points about Hashtable
:
- Synchronization:
- Unlike
HashMap
, which is not synchronized by default,Hashtable
is synchronized. This means that it is thread-safe, and multiple threads can safely access and modify aHashtable
concurrently without the need for external synchronization.
- Null Keys and Values:
Hashtable
does not allownull
keys or values. If you attempt to insert anull
key or value, it will throw aNullPointerException
.
- Underlying Data Structure:
Hashtable
uses a hash table data structure to store key-value pairs, similar toHashMap
. This allows for efficient key-based retrieval.
- Order:
- The order of key-value pairs in a
Hashtable
is not guaranteed. It does not maintain the order in which elements were inserted or accessed.
- Legacy:
Hashtable
is considered a legacy class and is not commonly used in modern Java development. Instead,HashMap
is preferred for its better performance in most use cases. However, if you need thread safety and are working with older code or legacy systems,Hashtable
may still be encountered.
- Common Methods:
put(K key, V value)
: Associates the specified key with the specified value in the map.get(Object key)
: Retrieves the value associated with the given key.remove(Object key)
: Removes the key-value pair associated with the given key.containsKey(Object key)
: Checks if the map contains the specified key.keys()
: Returns an enumeration of the keys in the map.elements()
: Returns an enumeration of the values in the map.
Here’s an example of using Hashtable
:
import java.util.Hashtable;
import java.util.Enumeration;
public class HashtableExample {
public static void main(String[] args) {
// Creating a Hashtable
Hashtable<String, Integer> ages = new Hashtable<>();
// Adding key-value pairs
ages.put("Alice", 25);
ages.put("Bob", 30);
ages.put("Charlie", 35);
// Retrieving a value
int aliceAge = ages.get("Alice"); // Retrieves the value associated with the key "Alice" (25)
// Iterating through keys using Enumeration
Enumeration<String> keys = ages.keys();
while (keys.hasMoreElements()) {
String key = keys.nextElement();
int value = ages.get(key);
System.out.println(key + " is " + value + " years old.");
}
}
}
It’s worth noting that while Hashtable
provides thread safety, in modern Java, ConcurrentHashMap
is often preferred for concurrent use cases because it offers better performance and more fine-grained control over locking.