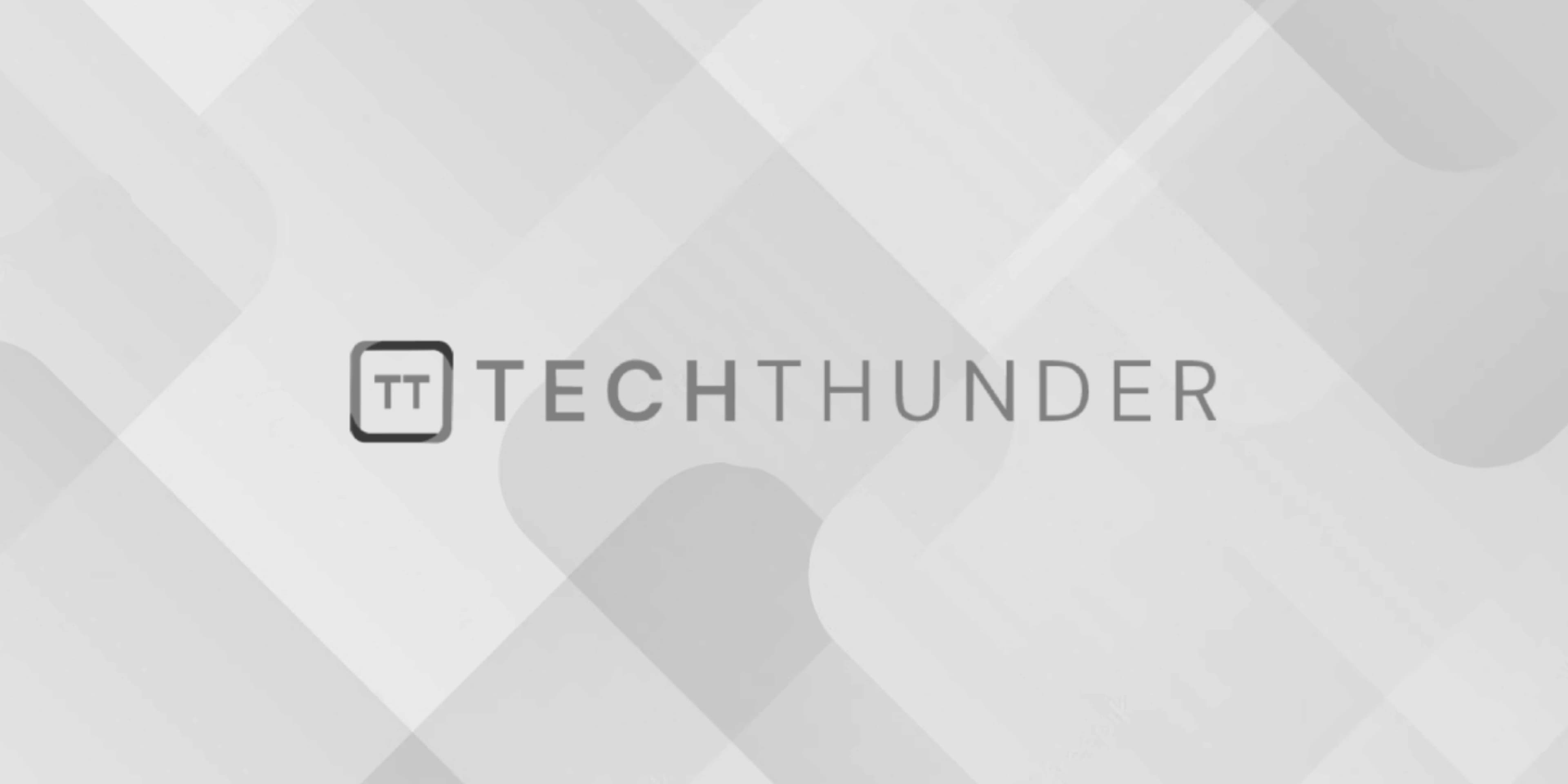
Java Queue & PriorityQueue
The Java Queue
is an interface that represents a collection of elements with two primary operations: adding elements to the back (enqueue) and removing elements from the front (dequeue). It follows a First-In-First-Out (FIFO) order, meaning that the element added first will be the first to be removed. The Queue
interface is part of the java.util
package and is extended by several implementing classes, including PriorityQueue
. Here, we’ll discuss Queue
and its implementing class PriorityQueue
:
Queue Interface:
- Common Methods:
add(E element)
: Adds the specified element to the queue if space is available; otherwise, it throws an exception.offer(E element)
: Adds the specified element to the queue if space is available; returnstrue
if successful, orfalse
if the queue is full.remove()
: Removes and returns the element at the front of the queue; throws an exception if the queue is empty.poll()
: Removes and returns the element at the front of the queue; returnsnull
if the queue is empty.element()
: Retrieves, but does not remove, the element at the front of the queue; throws an exception if the queue is empty.peek()
: Retrieves, but does not remove, the element at the front of the queue; returnsnull
if the queue is empty.size()
: Returns the number of elements in the queue.isEmpty()
: Checks if the queue is empty.
- Implementing Classes:
- Implementations of the
Queue
interface includeLinkedList
andPriorityQueue
.LinkedList
provides a basic FIFO queue, whilePriorityQueue
allows you to define a custom priority order for elements.
PriorityQueue:
- Ordering:
PriorityQueue
is an implementation of theQueue
interface that provides a priority queue behavior. Elements in aPriorityQueue
are ordered based on their natural order (if they areComparable
) or a specifiedComparator
. The highest-priority element is at the front.
- Common Methods:
add(E element)
(also known asoffer(E element))
: Adds the specified element to the queue according to its priority.remove()
: Removes and returns the highest-priority element.poll()
: Removes and returns the highest-priority element; returnsnull
if the queue is empty.peek()
: Retrieves, but does not remove, the highest-priority element; returnsnull
if the queue is empty.
- Custom Priority Order:
- You can use a custom
Comparator
to define a specific order of elements in thePriorityQueue
. This allows you to prioritize elements based on your own criteria.
Here’s an example of using PriorityQueue
to create a priority queue of tasks with custom priorities:
import java.util.PriorityQueue;
import java.util.Comparator;
class Task {
String name;
int priority;
public Task(String name, int priority) {
this.name = name;
this.priority = priority;
}
}
public class PriorityQueueExample {
public static void main(String[] args) {
PriorityQueue<Task> taskQueue = new PriorityQueue<>(Comparator.comparingInt(task -> task.priority));
taskQueue.offer(new Task("Task A", 3));
taskQueue.offer(new Task("Task B", 1));
taskQueue.offer(new Task("Task C", 2));
while (!taskQueue.isEmpty()) {
Task task = taskQueue.poll();
System.out.println("Processing: " + task.name);
}
}
}
The tasks are added to a PriorityQueue
based on their priority values. The tasks are processed in ascending order of priority, as defined by the custom Comparator
. This demonstrates the use of PriorityQueue
for managing tasks with custom priorities.