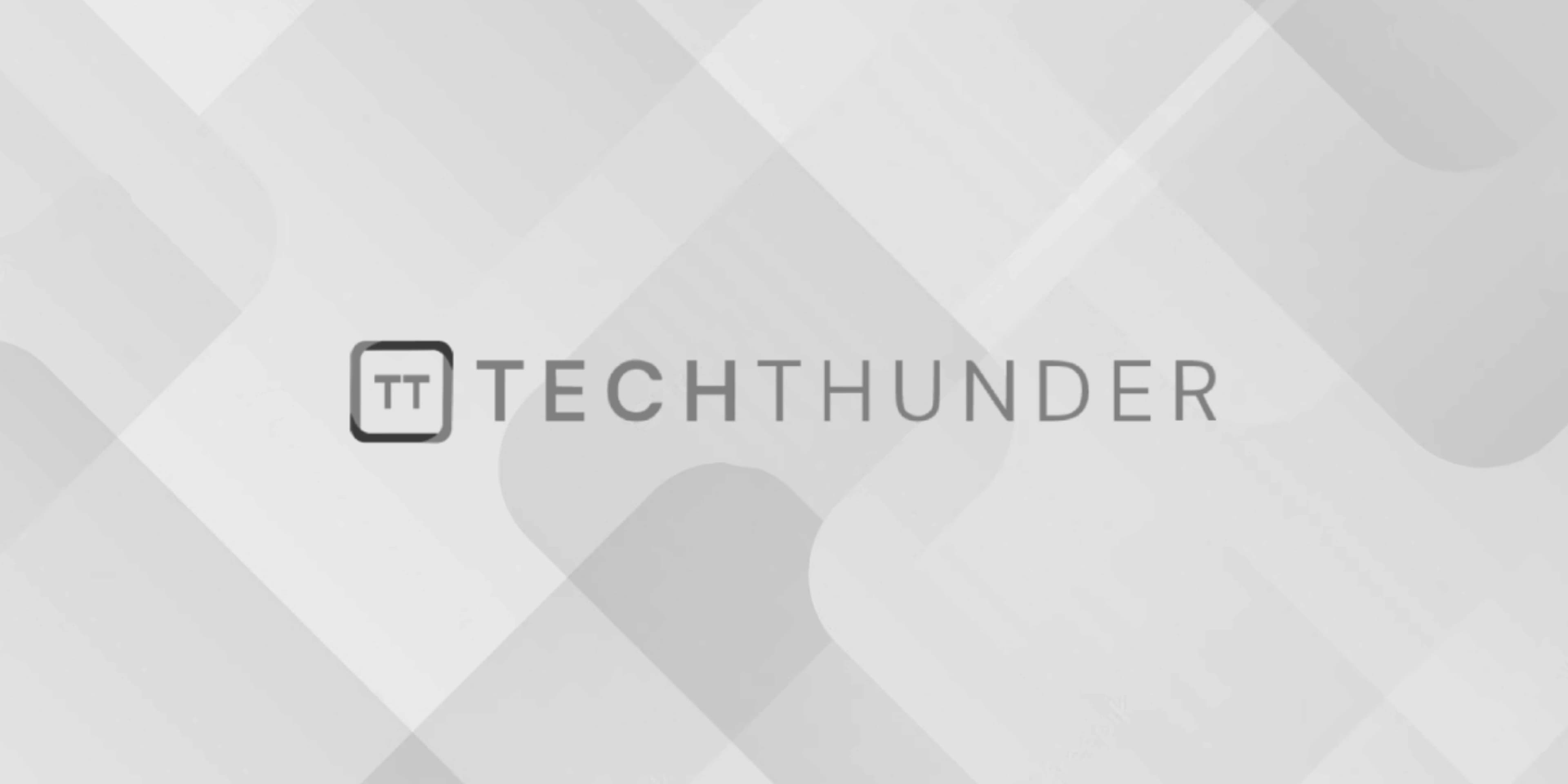
Java ConcurrentHashMap
The ConcurrentHashMap
is a class in the Java Collections Framework that provides a highly efficient and thread-safe way to manage and manipulate a hash table that can be accessed by multiple threads concurrently. It was introduced in Java 5 to address the need for a concurrent version of the traditional HashMap
.
The key features and characteristics of ConcurrentHashMap
include:
- Concurrency:
ConcurrentHashMap
is designed to be used in multi-threaded environments. It allows multiple threads to perform operations concurrently without the need for explicit synchronization. - Segmented Structure: Internally,
ConcurrentHashMap
is divided into segments, each of which acts as an independent hash table. This allows different threads to operate on different segments concurrently, reducing contention. - Thread-Safe Operations: All basic operations (such as
get
,put
,remove
, etc.) are thread-safe. This means that multiple threads can safely read and modify the map without causing data corruption. - Fine-Grained Locking: Instead of using a single lock for the entire map,
ConcurrentHashMap
uses a finer-grained approach where locks are applied to individual segments. This minimizes contention and improves performance. - Iterators: Iterating over a
ConcurrentHashMap
using its iterator provides a snapshot view of the data. The iterator is not affected by concurrent modifications made by other threads. - Scalability:
ConcurrentHashMap
is designed to work well with a large number of threads, providing good scalability for concurrent access patterns.
Here’s an example of how you might use ConcurrentHashMap
:
import java.util.concurrent.*;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
// Thread-safe operations
map.putIfAbsent("Four", 4);
map.remove("Two", 2);
// Iterate over the map
for (String key : map.keySet()) {
int value = map.get(key);
System.out.println(key + ": " + value);
}
}
}
Keep in mind that while ConcurrentHashMap
is suitable for many multi-threaded scenarios, you should still carefully design your application to ensure correct synchronization and to choose the appropriate concurrency level based on your specific requirements. Additionally, for applications with high read-to-write ratios or when you require more advanced features, you might also consider using other concurrent data structures or mechanisms provided by the Java java.util.concurrent
package.