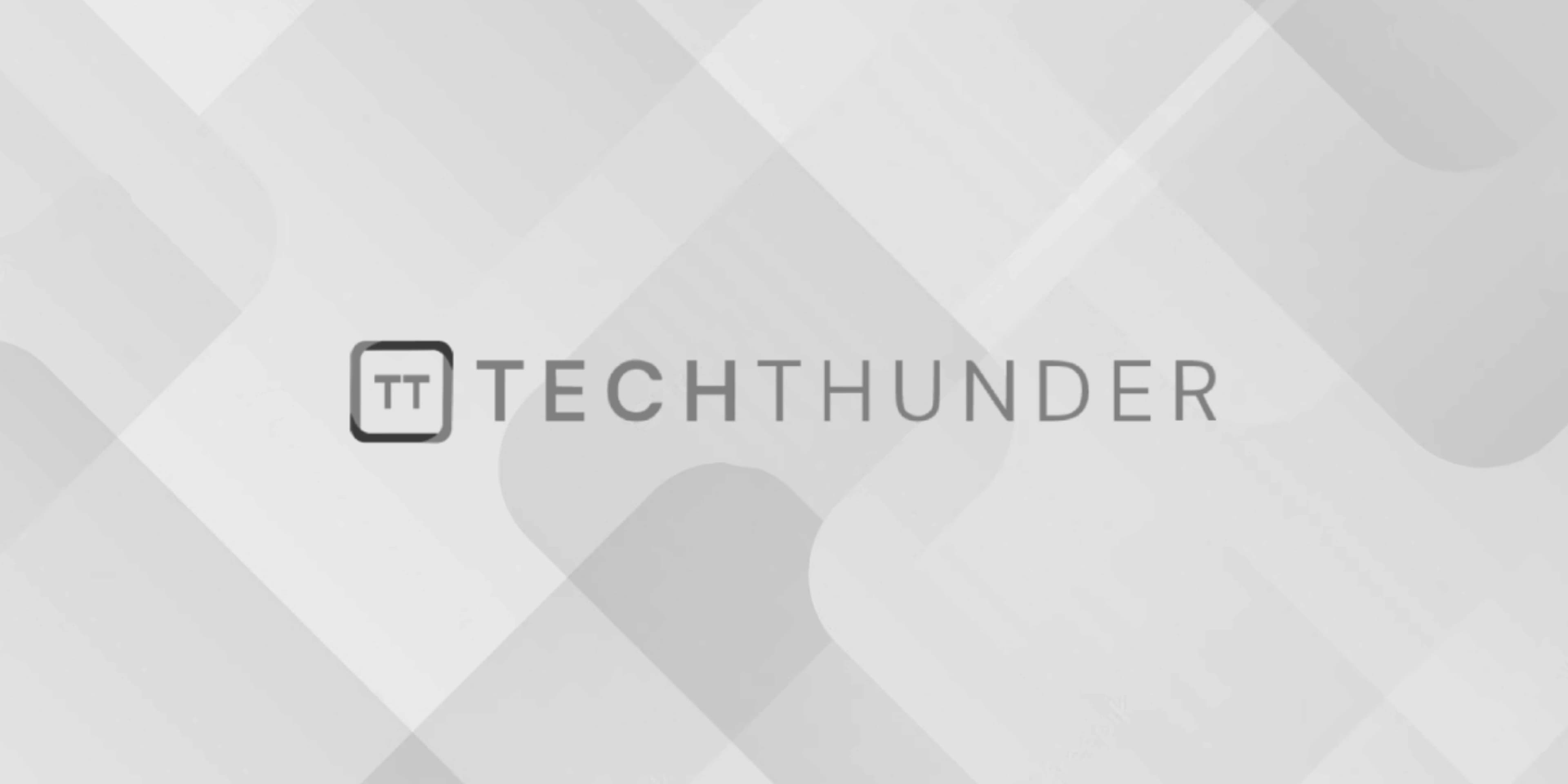
247 views
Add charts in Laravel using Chart JS
To add charts in a Laravel application using Chart.js, follow these steps:
- Install and Include Chart.js: Install Chart.js via npm:
Bash
npm install chart.js
Include Chart.js in your Laravel application by importing it in your JavaScript file (e.g., resources/js/app.js
):
JavaScript
import Chart from 'chart.js';
- Create a Route and Controller: Create a route and controller method that will fetch the data you need for your chart. For example:
PHP
// routes/web.php
Route::get('/chart-data', 'ChartController@chartData');
PHP
// app/Http/Controllers/ChartController.php
public function chartData()
{
$data = [10, 20, 30, 40, 50]; // Example data
return response()->json($data);
}
- Create a Vue Component: Create a Vue component (e.g.,
resources/js/components/ChartComponent.vue
) to render the Chart.js chart:
Vue
<template>
<div>
<canvas ref="chartCanvas"></canvas>
</div>
</template>
<script>
export default {
props: {
apiUrl: String
},
data() {
return {
chart: null
};
},
mounted() {
this.fetchChartData();
},
methods: {
async fetchChartData() {
try {
const response = await axios.get(this.apiUrl);
this.createChart(response.data);
} catch (error) {
console.error('Error fetching chart data:', error);
}
},
createChart(data) {
const ctx = this.$refs.chartCanvas.getContext('2d');
this.chart = new Chart(ctx, {
type: 'bar',
data: {
labels: ['Label 1', 'Label 2', 'Label 3', 'Label 4', 'Label 5'],
datasets: [
{
label: 'Chart Data',
data: data,
backgroundColor: 'rgba(75, 192, 192, 0.2)',
borderColor: 'rgba(75, 192, 192, 1)',
borderWidth: 1
}
]
},
options: {
responsive: true,
maintainAspectRatio: false
}
});
}
}
};
</script>
<style>
/* Add any necessary CSS styling for the chart container */
</style>
- Use the Vue Component: In a Blade template, use the Vue component by passing the API URL for chart data:
Vue
<chart-component api-url="{{ route('chart-data') }}"></chart-component>
Make sure to compile your JavaScript using Laravel Mix after creating or modifying Vue components:
Bash
npm run dev
That’s it! You’ve integrated Chart.js into your Laravel application using a Vue component to display a chart with data fetched from the server. Customize the chart type, styling, and data to fit your needs.