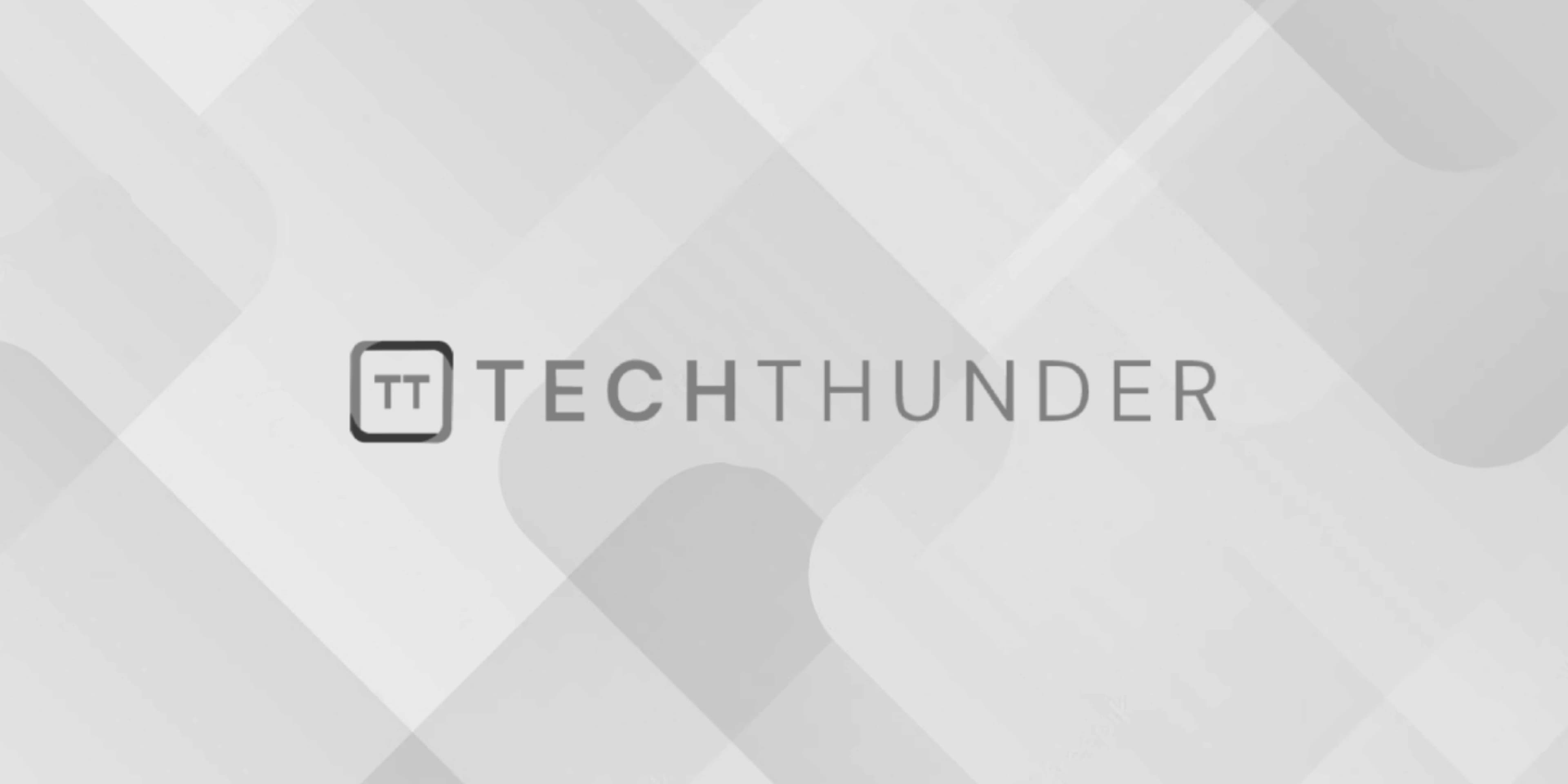
Generating Migrations in Laravel
The laravel migrations are a way to version and manage your database schema. You can use migrations to create, modify, and delete database tables and columns in an organized and incremental manner. Here’s how to generate migrations in Laravel:
1. Creating a Migration:
You can create a new migration file using the make:migration
Artisan command. By convention, migrations are stored in the database/migrations
directory. To create a new migration, open your terminal and run:
php artisan make:migration create_table_name
Replace table_name
with the name of the table you want to create. For example, to create a migration for a “posts” table:
php artisan make:migration create_posts_table
2. Editing the Migration File:
The previous command will generate a new migration file in the database/migrations
directory with a name like 2023_09_15_000000_create_posts_table.php
(the actual timestamp may vary). Open this file in a text editor or code editor.
In the generated migration file, you’ll see an up
method. This method defines the schema changes you want to make to the database. You can use Laravel’s Schema builder methods to create tables, define columns, and set constraints.
Here’s an example of a migration file for creating a “posts” table:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('posts');
}
}
In this example, we’re creating a “posts” table with columns for “id,” “title,” “content,” and timestamps for “created_at” and “updated_at.”
3. Running Migrations:
To execute the migrations and apply the schema changes to the database, run the following Artisan command:
php artisan migrate
This command will execute all pending migrations. Laravel keeps track of which migrations have been run in the database, so it will only run new migrations.
4. Rolling Back Migrations:
If you need to undo a migration, you can use the migrate:rollback
Artisan command. This will reverse the last batch of migrations:
php artisan migrate:rollback
You can also specify the --step
option to rollback a specific number of batches:
php artisan migrate:rollback --step=2
This command will rollback the last two batches of migrations.
5. Viewing Migration Status:
You can check the status of your migrations using the migrate:status
Artisan command. It shows which migrations have been run and which are pending:
php artisan migrate:status
These are the basic steps to generate and run migrations in Laravel. Migrations provide a structured and version-controlled way to manage your database schema, making it easier to work collaboratively and keep your database schema in sync with your application’s code.