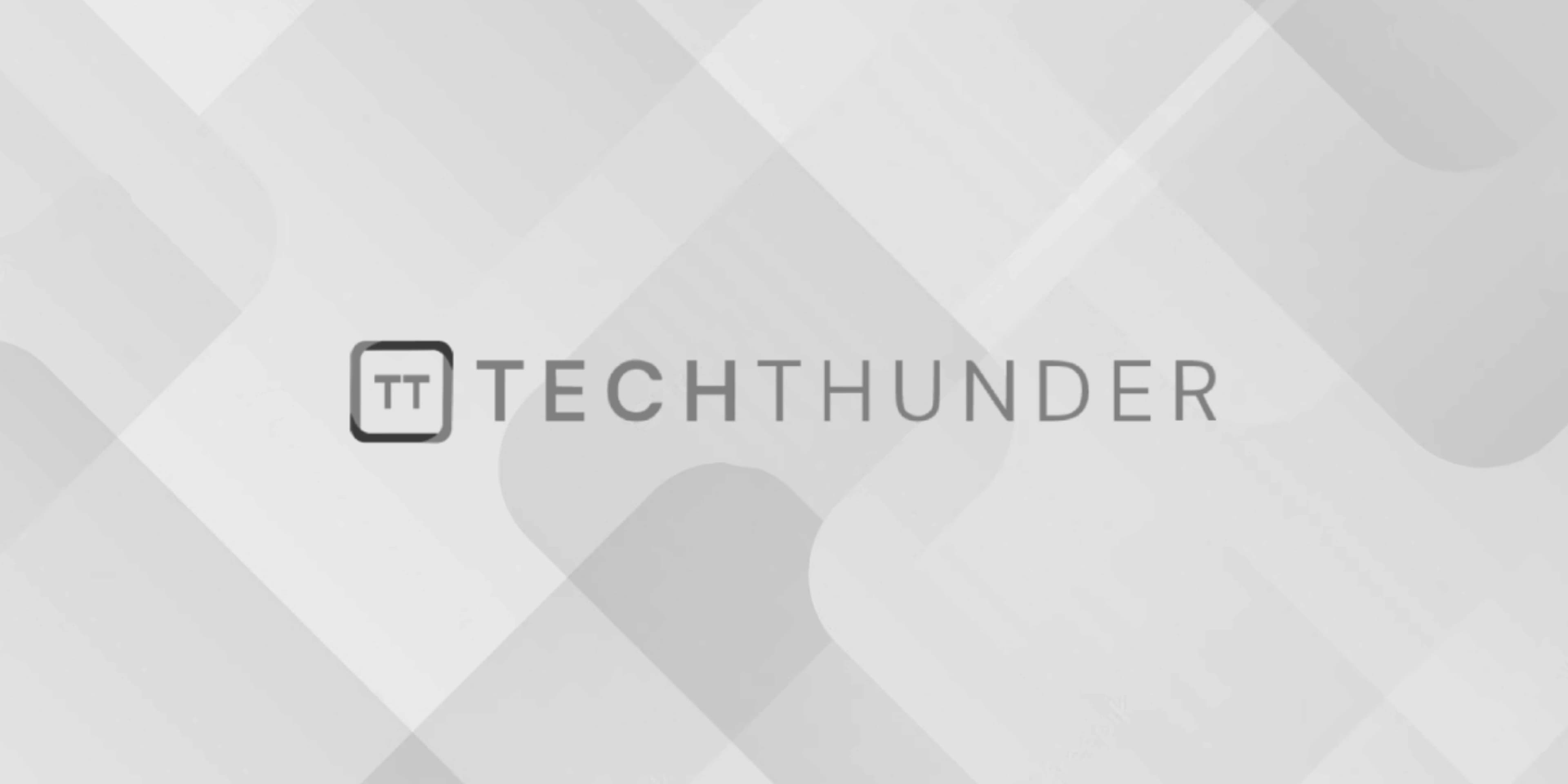
Send Email with Laravel 7/6 using Markdown Mailable Class
In Laravel 6 and 7, you can use Markdown Mailables to send beautifully designed emails using Markdown syntax. Markdown Mailables provide a clean and efficient way to compose and style your emails. Here’s how you can create and send an email using a Markdown Mailable class:
- Create a Markdown Mailable Class: Generate a Markdown Mailable class using the
php artisan
command-line tool:
php artisan make:mail WelcomeEmail
This will create a WelcomeEmail.php
file in the app/Mail
directory.
- Edit the Markdown Mailable Class: Open the
WelcomeEmail.php
file in theapp/Mail
directory and update thebuild
method to define your email’s subject and view:
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
use Illuminate\Contracts\Queue\ShouldQueue;
class WelcomeEmail extends Mailable
{
use Queueable, SerializesModels;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct()
{
//
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this->markdown('emails.welcome'); // resources/views/emails/welcome.blade.php
}
}
- Create the Markdown View: Create the corresponding Markdown view at
resources/views/emails/welcome.blade.php
. You can use Markdown syntax to structure and style your email content.
@component('mail::message')
# Welcome to our Application
Thanks for joining us! We're excited to have you on board.
@component('mail::button', ['url' => 'https://example.com'])
Visit Our Website
@endcomponent
Thanks,<br>
Your Application Team
@endcomponent
- Sending the Email: You can now send the email using your Markdown Mailable class. For example, from a controller method:
use Illuminate\Support\Facades\Mail;
use App\Mail\WelcomeEmail;
public function sendWelcomeEmail()
{
Mail::to('[email protected]')->send(new WelcomeEmail());
return 'Welcome email sent!';
}
- Queueing the Email (Optional): If you want to queue the email for sending asynchronously, you can use the
queue
method:
Mail::to('[email protected]')->queue(new WelcomeEmail());
Remember to customize the email’s content, subject, and other details according to your application’s needs. Markdown Mailables provide a great way to create visually appealing and responsive email templates.
Keep in mind that the steps provided here are based on Laravel 6 and 7 as of my last update in September 2021. If you’re using a newer version of Laravel, there might be some differences, so always refer to the official documentation for the most accurate and up-to-date information.