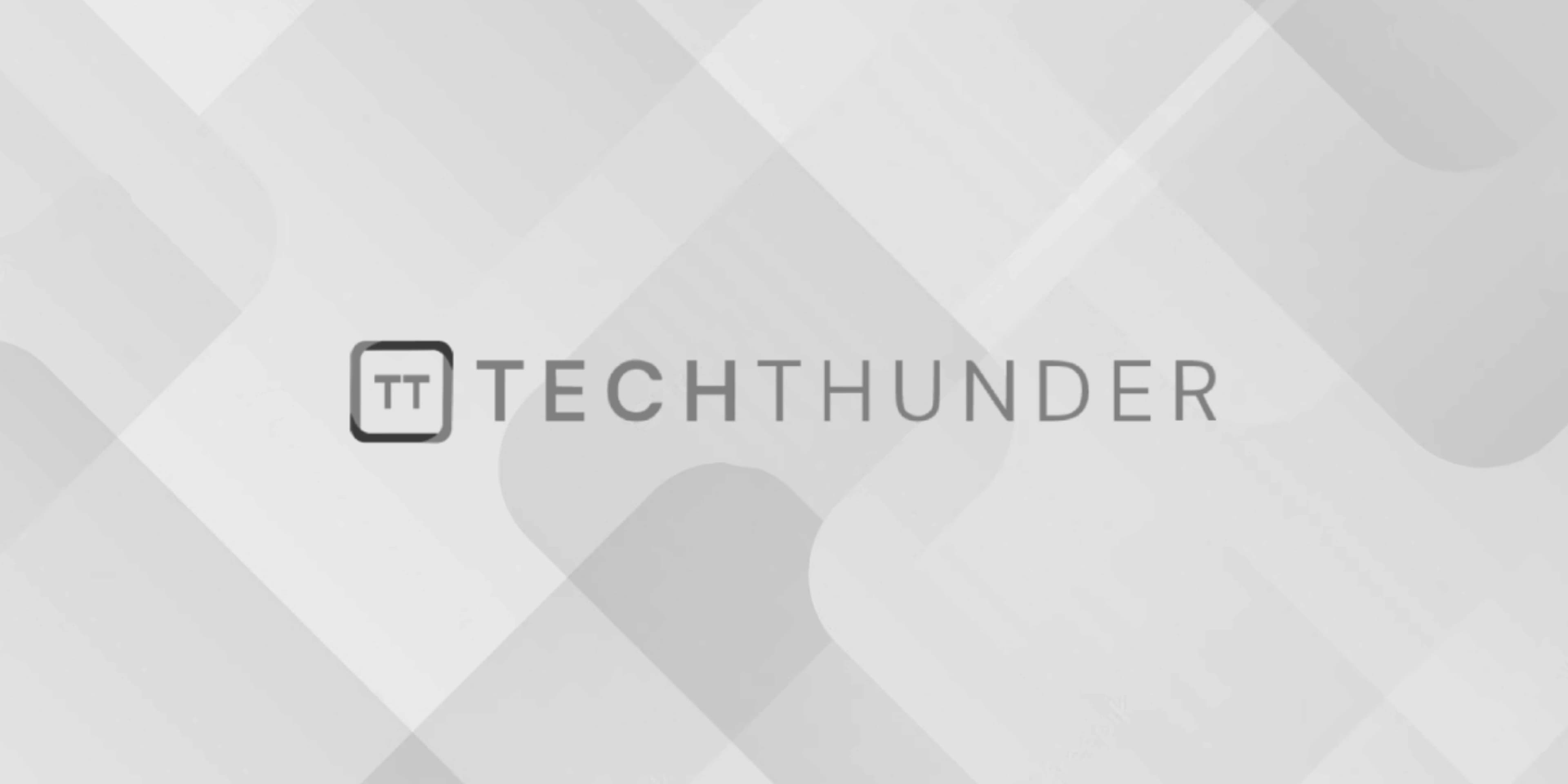
Laravel Database
Laravel provides a powerful and expressive database management system, making it easier for developers to interact with databases. It offers an Object-Relational Mapping (ORM) system called Eloquent, a query builder, and database migration tools. Here’s an overview of working with databases in Laravel:
1. Configuration:
Laravel’s database configuration is defined in the .env
file. You can specify database connection details such as the database type, host, username, password, and database name. By default, Laravel uses the MySQL database, but it supports other database systems like PostgreSQL, SQLite, and SQL Server.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=my_database
DB_USERNAME=my_username
DB_PASSWORD=my_password
2. Eloquent ORM:
Eloquent is Laravel’s implementation of the Active Record pattern, allowing you to interact with databases using PHP objects. To use Eloquent, you define a model class for each database table. Eloquent models provide an expressive way to query and manipulate data.
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $table = 'users';
}
With Eloquent, you can perform common database operations like creating, updating, retrieving, and deleting records easily:
// Create a new user record
$user = new User;
$user->name = 'John Doe';
$user->email = '[email protected]';
$user->save();
// Retrieve a user by ID
$user = User::find(1);
// Update a user's email
$user->email = '[email protected]';
$user->save();
// Delete a user
$user->delete();
3. Query Builder:
Laravel’s query builder provides a fluent, expressive way to build SQL queries without writing raw SQL statements. You can use the DB
facade to access the query builder:
use Illuminate\Support\Facades\DB;
$users = DB::table('users')
->where('status', 'active')
->orderBy('created_at', 'desc')
->get();
4. Migrations:
Database migrations in Laravel allow you to version and manage your database schema. You can create, update, or delete database tables and columns in a structured and incremental way. Migrations are created using Artisan commands and are located in the database/migrations
directory.
# Create a new migration
php artisan make:migration create_users_table
# Run all pending migrations
php artisan migrate
5. Seeding:
Seeding in Laravel allows you to populate your database with initial data. You can create seeder classes using Artisan commands and define the data you want to insert.
# Create a new seeder
php artisan make:seeder UsersTableSeeder
# Run seeders to populate the database
php artisan db:seed
6. Database Transactions:
Laravel provides a simple way to work with database transactions, ensuring data consistency. You can use the DB::transaction
method to wrap a series of database operations in a transaction:
DB::transaction(function () {
// Database operations here
}, 5); // 5 is the number of times to retry the transaction on failure
7. Raw SQL Queries:
While Laravel promotes the use of Eloquent and the query builder, you can still execute raw SQL queries using the DB
facade:
$users = DB::select('SELECT * FROM users WHERE id = ?', [1]);
These are some of the fundamental concepts and tools for working with databases in Laravel. Laravel’s database management features make it easy to interact with databases and build robust web applications with efficient database operations.