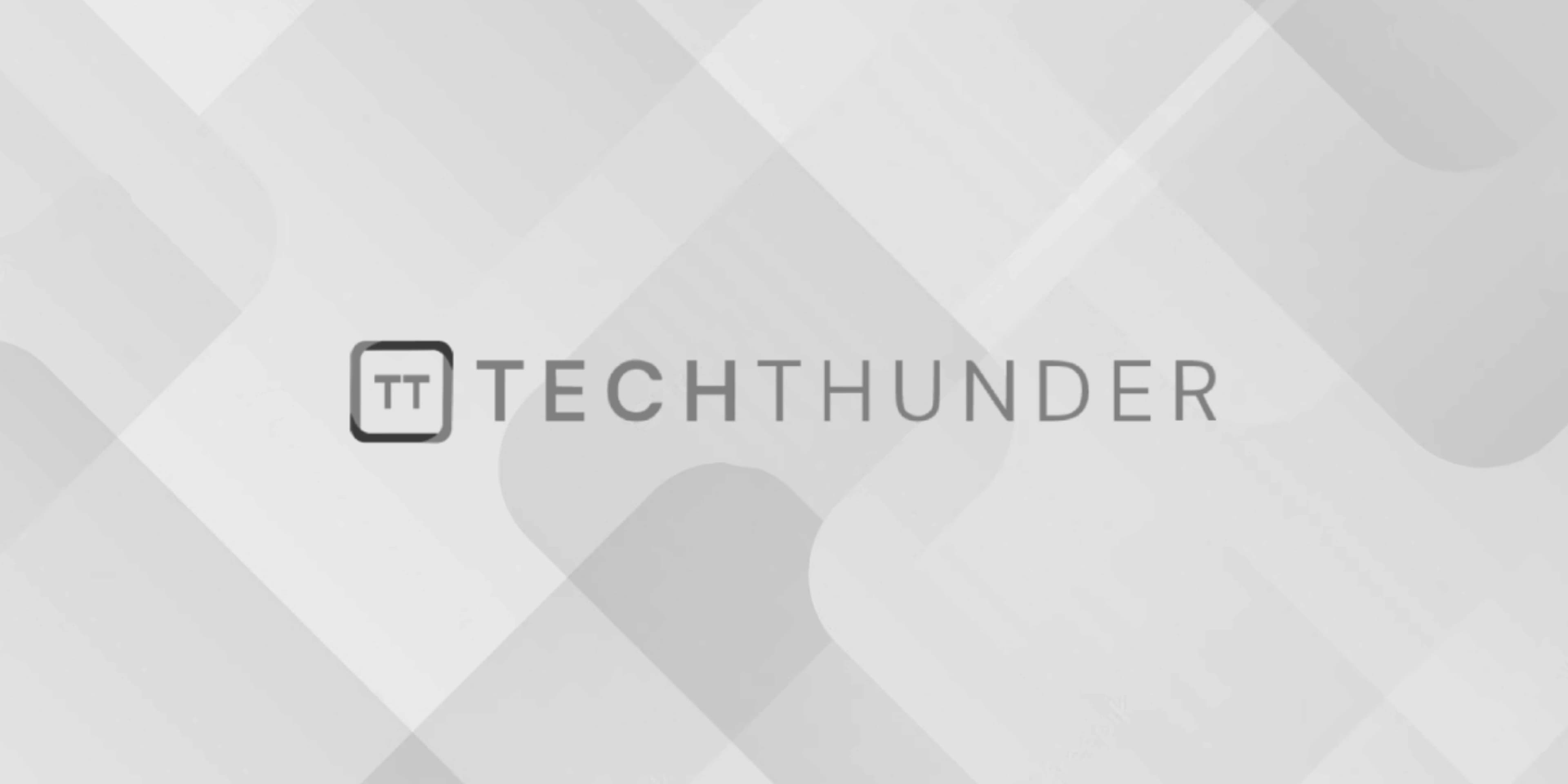
Laravel 5.7 Modular Structure Application
The Laravel 5.7 framework doesn’t come with built-in support for modular structures out of the box, but you can still create a modular structure for your application to organize your codebase in a more maintainable way. Here’s a general approach to implementing a modular structure in a Laravel 5.7 application:
- Create a “Modules” Directory: Start by creating a “Modules” directory at the root of your Laravel project. This directory will contain your individual modules, each with its own set of controllers, models, views, and routes.
/your-laravel-project
/app
/bootstrap
/config
/database
/Modules
/ModuleName
/Controllers
/Models
/Views
/Routes
/AnotherModuleName
/Controllers
/Models
/Views
/Routes
/public
/resources
/routes
...
- Create Modules with Controllers, Models, Views, and Routes: For each module you want to create, follow a similar structure to the default Laravel structure within the respective module’s directory. For example, create controllers in
Modules/ModuleName/Controllers
, models inModules/ModuleName/Models
, views inModules/ModuleName/Views
, and routes inModules/ModuleName/Routes
. - Route File for Each Module: Create a separate route file for each module in the
Modules/ModuleName/Routes
directory. You can define routes specific to that module in this file.
// Modules/ModuleName/Routes/web.php
Route::group(['namespace' => 'Modules\ModuleName\Controllers', 'prefix' => 'module-name'], function () {
// Define your routes here
});
- Load Module Routes: In your main
routes/web.php
file, include the route files for each module:
// routes/web.php
foreach (glob(base_path('Modules/*/Routes/web.php')) as $moduleRoutes) {
include $moduleRoutes;
}
// Define your other application routes here
- Use Namespaces and Autoloading: Make sure you’re using proper namespaces and autoloading for classes in your modules. Also, don’t forget to register your modules in the composer.json file:
"autoload": {
"psr-4": {
"App\\": "app/",
"Modules\\": "Modules/"
}
},
- Service Providers and Configuration: If your modules require additional service providers or configuration files, you can manually load them in your
config/app.php
andconfig/services.php
files.
Remember that this modular structure is not as integrated as what you might find in some other frameworks, but it can still help you organize your codebase better. If you’re starting a new project, you might want to consider using Laravel 8 (or newer) which introduced native support for package discovery and improved modular structure capabilities through “packages” and namespaces.
Keep in mind that Laravel and its ecosystem have evolved since Laravel 5.7, so it’s a good idea to check the latest documentation and best practices for your specific version of Laravel.