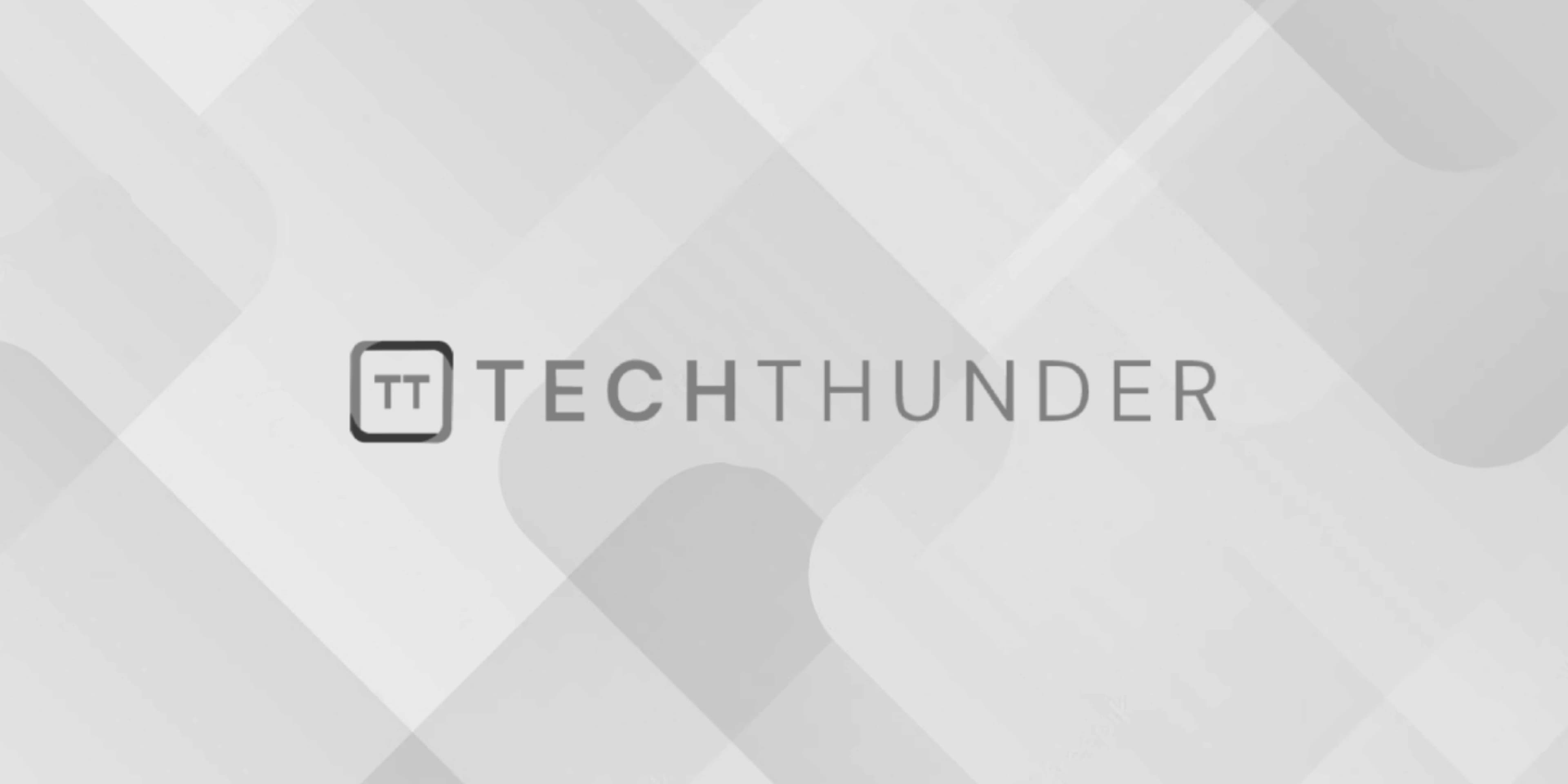
Creating First Laravel Project
Creating your first Laravel project is an exciting step towards building web applications using the Laravel PHP framework. Here are the steps to create your first Laravel project:
1. Prerequisites:
Before you start, make sure you have the following prerequisites installed on your system:
- PHP: Laravel requires PHP 7.4 or higher.
- Composer: A PHP dependency manager. You can download it from https://getcomposer.org/download/.
- Laravel Installer (optional): You can install the Laravel Installer globally using Composer:
composer global require laravel/installer
- A web server (e.g., Apache, Nginx) with PHP support.
2. Create a New Laravel Project:
You can create a new Laravel project using Composer or the Laravel Installer.
Using Composer (Recommended):
Open your terminal and run the following command to create a new Laravel project. Replace my-laravel-app
with your desired project name:
composer create-project --prefer-dist laravel/laravel my-laravel-app
This command will download the Laravel framework and its dependencies, setting up a new project in a directory named my-laravel-app
.
Using Laravel Installer (Optional):
If you’ve installed the Laravel Installer globally, you can create a new project using the following command:
laravel new my-laravel-app
3. Configure Your Environment:
Navigate to the project directory:
cd my-laravel-app
In the project’s root directory, you’ll find a file named .env.example
. Duplicate this file and rename it to .env
. Update the .env
file with your database credentials and other configuration settings.
Generate a unique application key by running:
php artisan key:generate
4. Serve Your Laravel Application:
You can use Laravel’s built-in development server to run your application. In the terminal, navigate to your project’s directory and run:
php artisan serve
This will start the development server, and you’ll see a message indicating the server is running at a specific URL (usually http://127.0.0.1:8000
). Open this URL in your web browser to access your Laravel application.
5. Explore Your First Laravel Project:
Congratulations! You’ve created your first Laravel project. You can now start building your web application using Laravel’s powerful features, such as routing, controllers, views, and database interactions.
Here are a few things to explore:
- Routes: Define your application’s routes in the
routes/web.php
file. - Controllers: Create controllers in the
app/Http/Controllers
directory to handle requests. - Views: Store your views in the
resources/views
directory and use Blade templating for dynamic content. - Database: Configure your database connection in the
.env
file and use Laravel’s Eloquent ORM for database interactions. - Middleware: Learn about middleware for handling tasks like authentication and authorization.
Refer to the official Laravel documentation (https://laravel.com/docs) for detailed information on how to build web applications with Laravel. Laravel’s documentation is comprehensive and a valuable resource as you start working on your project.