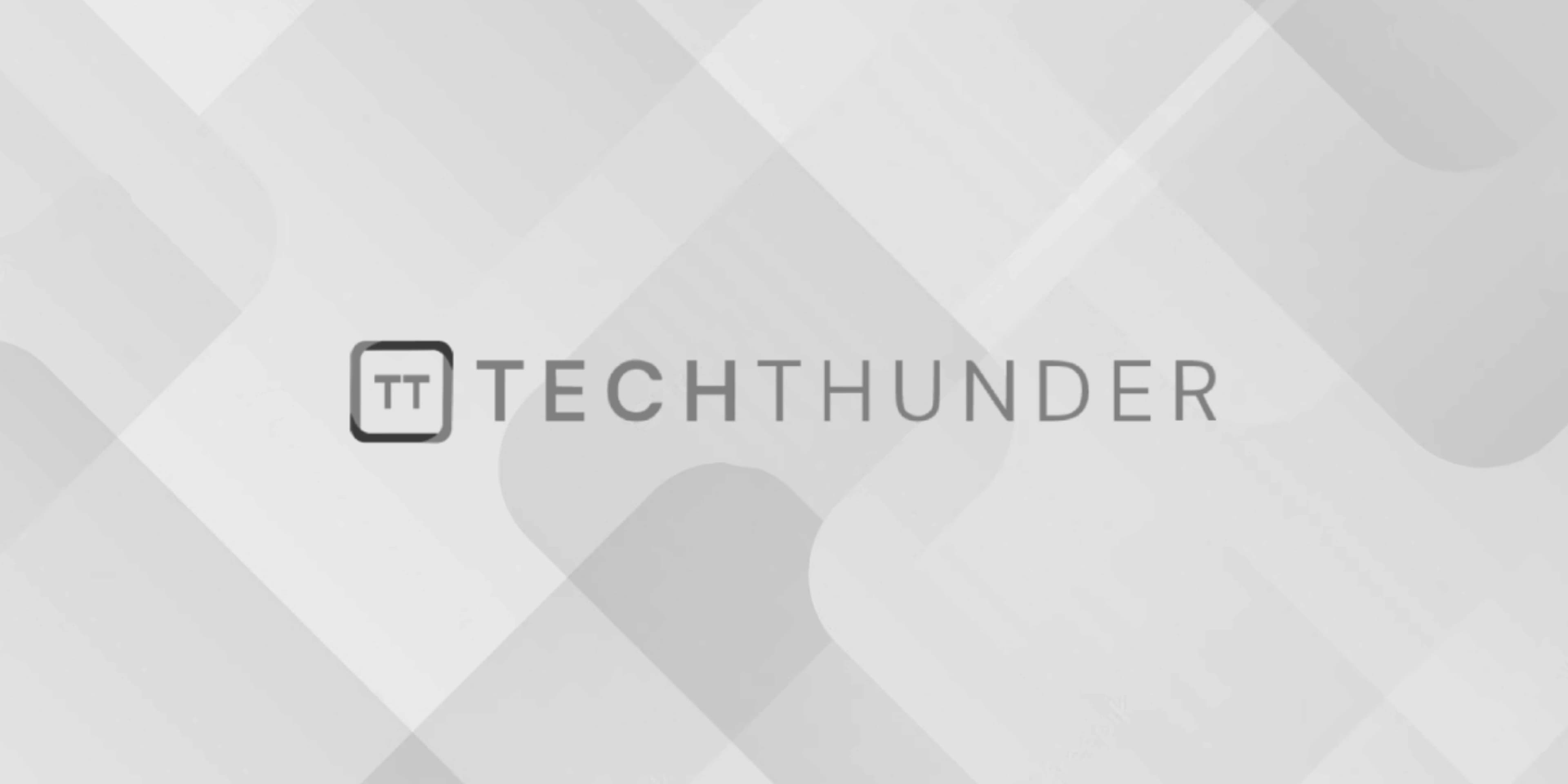
Elasticsearch with pagination in Laravel 5
The implement Elasticsearch with pagination in a Laravel 5 application, you can follow these steps:
Step 1: Install Elasticsearch
Ensure that you have Elasticsearch installed and running on your server. You can download and install Elasticsearch from the official website: https://www.elastic.co/downloads/elasticsearch
Step 2: Install Elasticsearch Client for Laravel
Laravel provides a package called “Scout” to integrate with Elasticsearch. You can install it using Composer:
composer require laravel/scout
Step 3: Configure Elasticsearch in Laravel
Open your Laravel application’s .env
file and add the Elasticsearch connection details:
SCOUT_DRIVER=elasticsearch
ELASTICSEARCH_HOST=http://localhost:9200
You can adjust the host and port according to your Elasticsearch setup.
Step 4: Create an Elasticsearch Index for Your Model
You need to create an Elasticsearch index for the model you want to search. Use the scout:import
Artisan command to do this:
php artisan scout:import "App\Models\YourModel"
Step 5: Implement Pagination
You can use Laravel’s built-in pagination features in combination with Elasticsearch. Here’s an example of how to do this in a controller:
use App\Models\YourModel;
public function search(Request $request)
{
$query = $request->input('q');
$perPage = 10; // Adjust the number of items per page as needed
$results = YourModel::search($query)->paginate($perPage);
return view('search_results', compact('results'));
}
In this example, replace YourModel
with the model you want to search and search_results
with the name of your Blade view for displaying the search results.
Step 6: Display Pagination in Your Blade View
In your Blade view (search_results.blade.php
in this case), you can display the pagination links like this:
<div class="pagination">
{{ $results->links() }}
</div>
This code will render pagination links at the bottom of your search results.
That’s it! You’ve implemented Elasticsearch with pagination in your Laravel 5 application. You can adjust the search logic and pagination settings according to your project’s requirements.