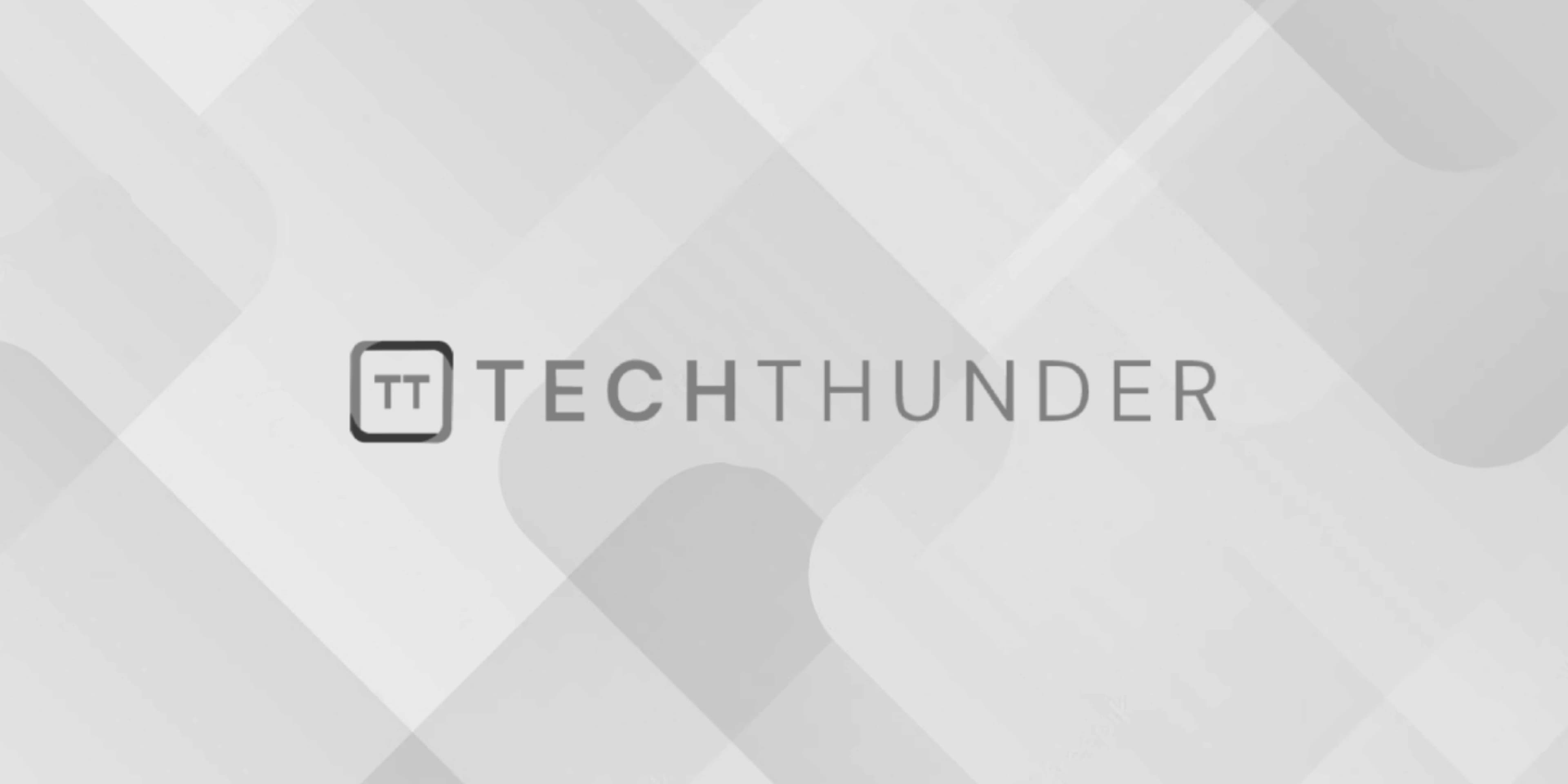
203 views
Laravel Vue JS Axios Post Request
In Laravel, Vue.js, and Axios, you can use Axios to make POST requests to your Laravel backend. Here’s a step-by-step guide on how to make a POST request using Axios in a Vue.js component:
- Install Axios: If you haven’t already, you need to install Axios in your Vue.js project:
Bash
npm install axios
- Create a Vue Component: Create a Vue component where you want to handle the POST request. For example, you can create a new component named
PostForm.vue
. - Import Axios: Import Axios at the beginning of your Vue component script section:
Vue
<script>
import axios from 'axios';
export default {
// Component code...
}
</script>
- Define Data Properties: In your component, define data properties to hold the form data and any response data from the backend:
Vue
<script>
export default {
data() {
return {
formData: {
// Your form fields here
},
response: null,
error: null
};
},
// Other component methods...
}
</script>
- Create a Method for Sending POST Request: Create a method that uses Axios to send a POST request to your Laravel backend:
Vue
<script>
export default {
data() {
// ...
},
methods: {
submitForm() {
axios.post('/api/your-endpoint', this.formData)
.then(response => {
this.response = response.data;
this.error = null;
})
.catch(error => {
this.error = error.response.data.error;
this.response = null;
});
}
}
}
</script>
Replace /api/your-endpoint
with the actual URL of your Laravel backend endpoint.
- Create the Form in the Template: In your component’s template, create a form and bind the form fields to the
formData
properties. Also, add a submit button that calls thesubmitForm
method:
Vue
<template>
<div>
<form @submit.prevent="submitForm">
<!-- Form fields -->
<input v-model="formData.field1" type="text" placeholder="Field 1">
<input v-model="formData.field2" type="text" placeholder="Field 2">
<button type="submit">Submit</button>
</form>
<p v-if="response">{{ response.message }}</p>
<p v-if="error">{{ error }}</p>
</div>
</template>
- Use the Vue Component: Finally, use your Vue component in your application where needed.
Remember to adjust the endpoint URL and data properties according to your application’s structure and needs. Also, make sure your Laravel routes and controllers are set up to handle the incoming POST request.
This example outlines the basic steps for making a POST request using Axios in a Vue.js component within a Laravel application. Adjustments and additional logic may be necessary depending on your specific use case and requirements.