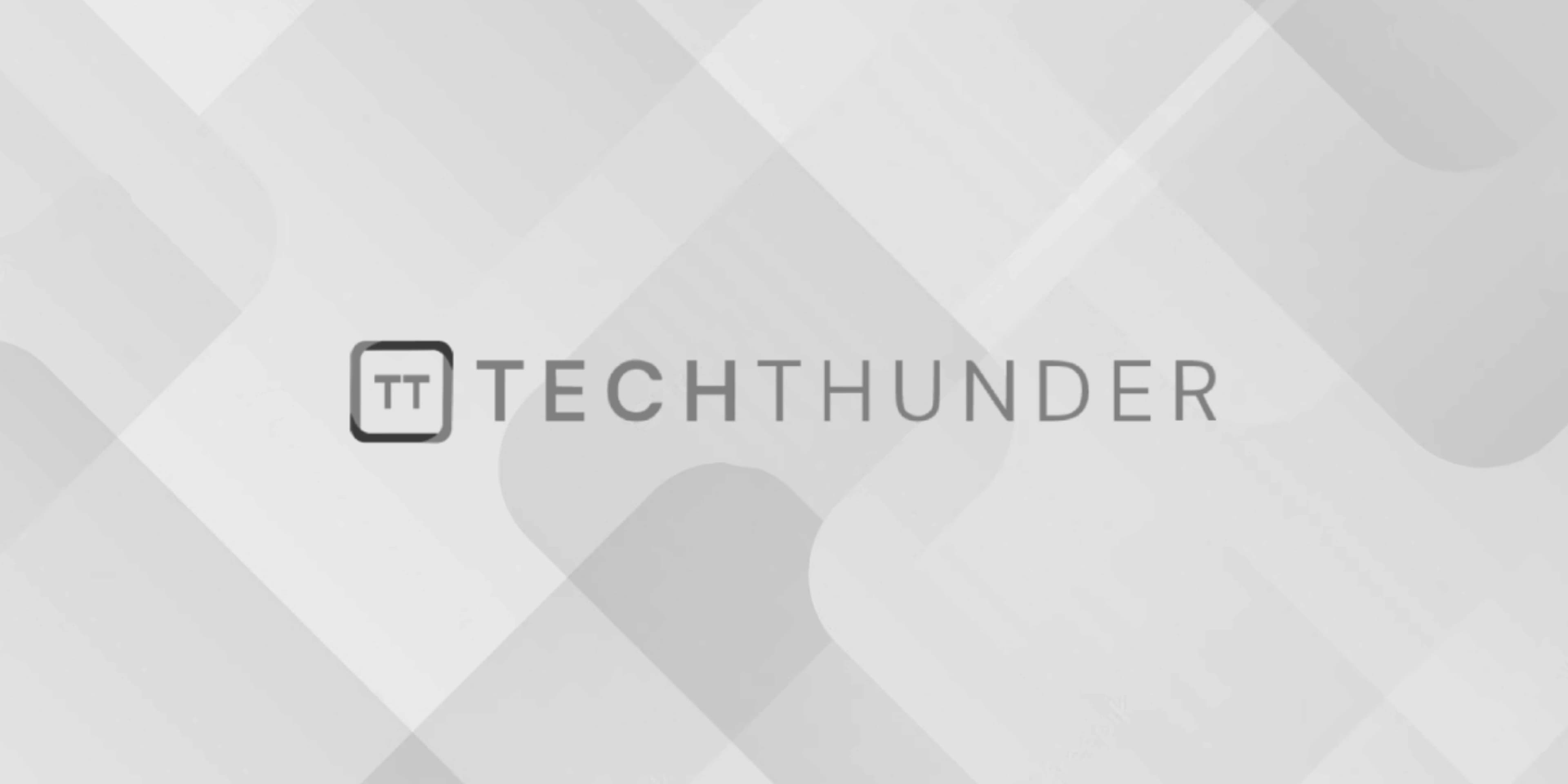
Routing Controllers
The laravel routing controllers allow you to define routes that are associated with controller actions, enabling you to handle HTTP requests in a more organized and structured manner. With routing controllers, you can separate your application’s logic into dedicated controller classes, making your code cleaner and more maintainable.
Here’s how to define routes that point to controller actions in Laravel:
1. Create a Controller:
Before you can route to controller actions, you need to create a controller class. You can use the make:controller
Artisan command to generate a new controller. For example, to create a controller named “HomeController,” you would run:
php artisan make:controller HomeController
This will generate a new controller file in the app/Http/Controllers
directory.
2. Define a Controller Action:
Inside your controller class, you can define methods (controller actions) that will handle specific HTTP requests. For example, you might define an index
method to handle requests to the home page:
use Illuminate\Http\Request;
class HomeController extends Controller
{
public function index()
{
return view('home');
}
}
In this example, the index
method returns a view named “home” when accessed.
3. Define a Route:
To route an HTTP request to a controller action, you can define a route in your routes/web.php
file. You typically use the Route::get
, Route::post
, or similar methods to define routes. For example:
Route::get('/', 'HomeController@index');
In this case, when a GET request is made to the root URL (“/”), Laravel will invoke the index
method of the HomeController
class.
4. Route Parameters:
You can pass route parameters to controller actions by including placeholders in your route definitions. For example:
Route::get('user/{id}', 'UserController@show');
In this route, {id}
is a parameter that will be passed to the show
method of the UserController
class. You can access this parameter in the method using the method’s parameter list.
5. Middleware:
You can apply middleware to routes to perform tasks like authentication and authorization. Middleware can be added directly to the route definition:
Route::get('profile', 'ProfileController@show')->middleware('auth');
In this example, the “auth” middleware is applied to the “show” method of the “ProfileController.”
6. Route Naming:
You can name routes to make it easier to generate URLs in your views and controllers. To name a route, use the name
method:
Route::get('user/{id}', 'UserController@show')->name('user.show');
Now you can generate URLs for this route using the route’s name:
$url = route('user.show', ['id' => 1]);
These are the basic steps for routing to controller actions in Laravel. By using controllers and defining routes this way, you can create organized and maintainable code for handling HTTP requests in your Laravel application.