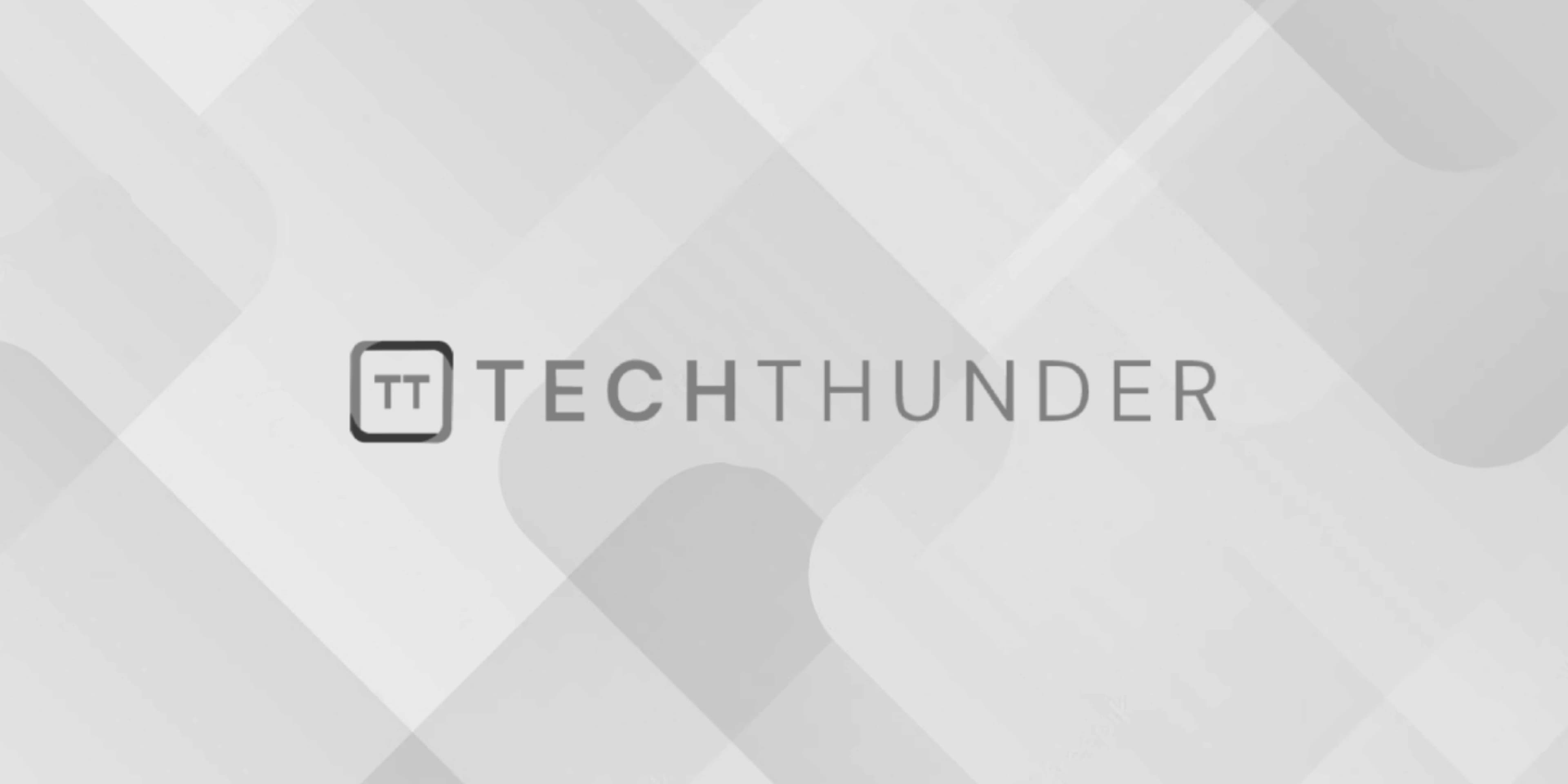
Laravel Middleware
Middleware in Laravel is a crucial part of the request handling process. It allows you to filter and modify incoming HTTP requests and outgoing responses before they reach your application’s routes or after they leave your application’s controllers. Middleware is used to perform tasks such as authentication, authorization, logging, request and response modification, and more. Laravel provides several built-in middleware, and you can also create custom middleware to suit your application’s specific needs.
Here’s how you can work with middleware in Laravel:
- Built-in Middleware: Laravel includes several built-in middleware for common tasks. Some commonly used built-in middleware include:
auth
: Checks if the user is authenticated. Redirects to the login page if not.guest
: Checks if the user is a guest (not authenticated).throttle
: Limits the rate at which certain routes can be accessed to prevent abuse.cors
: Handles Cross-Origin Resource Sharing (CORS) requests. You can apply these middleware to routes in yourroutes/web.php
orroutes/api.php
file:
Route::get('/dashboard', 'DashboardController@index')->middleware('auth');
- Creating Custom Middleware: You can create custom middleware using the
make:middleware
Artisan command:
php artisan make:middleware CustomMiddleware
This will create a new middleware class in the app/Http/Middleware
directory. You can then define custom logic in the handle
method of your middleware:
public function handle($request, Closure $next)
{
// Custom middleware logic here
return $next($request);
}
- Middleware Groups: You can group multiple middleware together and apply them to routes as a group. This is useful when you want to apply the same set of middleware to multiple routes:
Route::middleware(['auth', 'custom'])->group(function () {
// Routes that use both 'auth' and 'custom' middleware
});
- Middleware Parameters: You can pass parameters to your custom middleware by specifying them when applying the middleware to a route:
Route::get('/example', 'ExampleController@index')->middleware('custom:param1,param2');
Then, in your middleware’s handle
method, you can access these parameters:
public function handle($request, Closure $next, $param1, $param2)
{
// Use $param1 and $param2
return $next($request);
}
- Terminable Middleware: Terminable middleware allows you to perform actions after the response has been sent to the client. To create terminable middleware, you can implement the
TerminableMiddleware
interface and define theterminate
method:
public function terminate($request, $response)
{
// Perform actions after the response has been sent
}
Laravel will automatically call the terminate
method after the response has been sent.
- Global Middleware: Global middleware is executed on every HTTP request to your application. You can specify global middleware in the
$middleware
property of theapp/Http/Kernel.php
file:
protected $middleware = [
// ...
\App\Http\Middleware\CustomGlobalMiddleware::class,
];
Middleware in Laravel provides a powerful and flexible way to add functionality to your application’s request handling process. It helps you keep your code organized and allows you to apply various filters and modifications to incoming requests and outgoing responses.