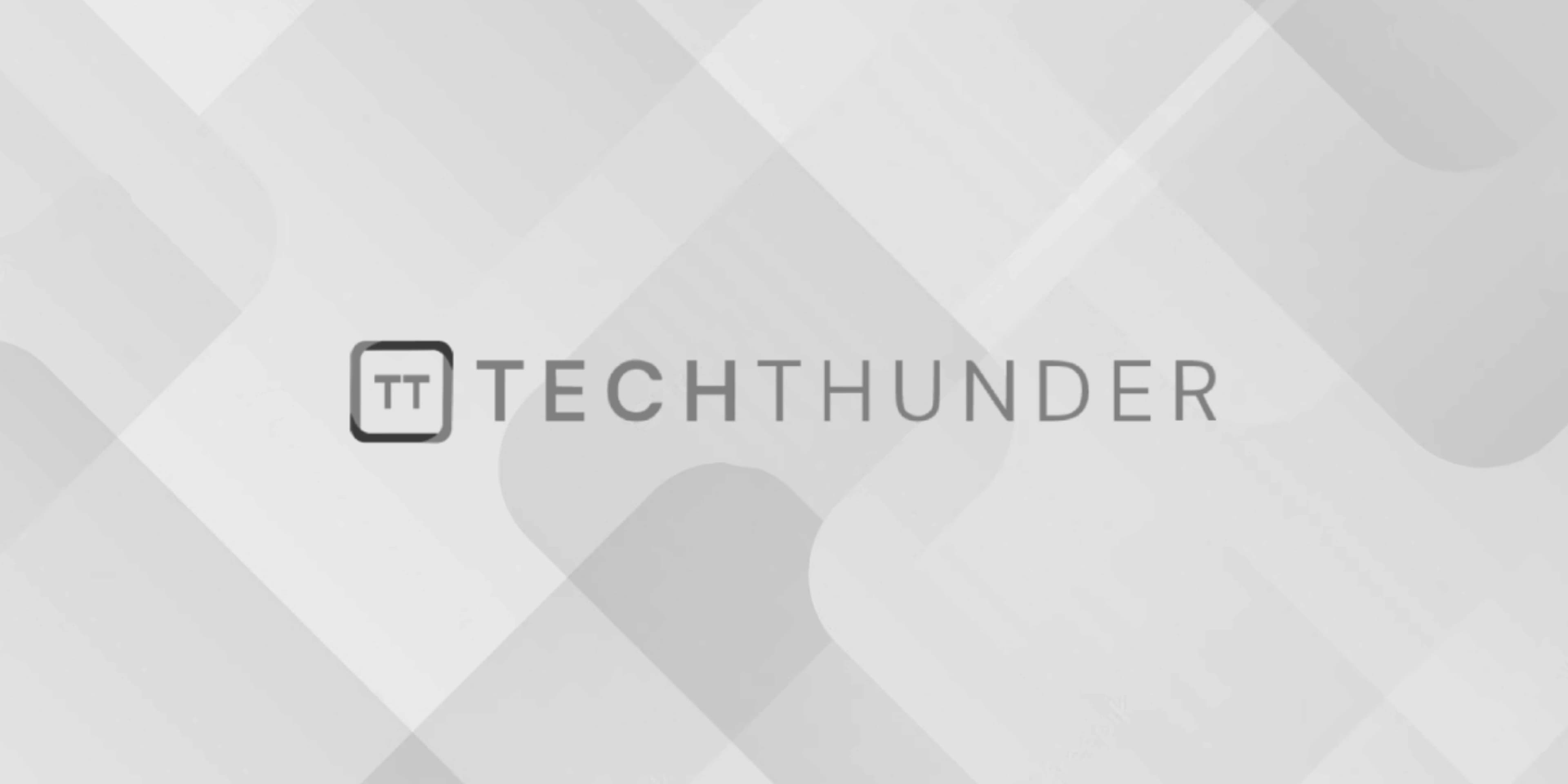
Laravel Migration
The Laravel, database migrations are a way to version and manage your database schema. Migrations allow you to define changes to your database schema in a structured and incremental way, making it easy to collaborate with other developers and keep your database schema in sync with your application code.
Here’s how to work with Laravel migrations:
1. Creating a Migration:
You can create a new migration using the make:migration
Artisan command. For example, to create a migration for creating a “posts” table:
php artisan make:migration create_posts_table
This will generate a new migration file in the database/migrations
directory.
2. Defining the Schema:
In the generated migration file, you can define the structure of the database table using the Schema
facade. For example, to create a “posts” table with columns for a title and content:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('posts');
}
}
In this example, we’ve defined the table’s columns, including an “id” column (for the primary key), “title,” “content,” and timestamps for created and updated dates.
3. Running Migrations:
To execute the migrations and create the database table, use the migrate
Artisan command:
php artisan migrate
This will run all pending migrations. Laravel keeps track of which migrations have already been executed in the database, so it will only run new migrations.
4. Rolling Back Migrations:
If you need to undo a migration, you can use the migrate:rollback
Artisan command. This will execute the down
method of the last batch of migrations:
php artisan migrate:rollback
5. Viewing Migration Status:
You can view the status of your migrations using the migrate:status
Artisan command. This will show you which migrations have been run and which are pending:
php artisan migrate:status
6. Modifying Migrations:
If you need to modify an existing table, you can create a new migration using the make:migration
command and then use the Schema
facade to modify the table. For example, to add a “published_at” column to the “posts” table:
php artisan make:migration add_published_at_to_posts
In the generated migration file, you can add the column definition to the up
method and a rollback definition to the down
method.
7. Refreshing the Database:
To completely rebuild the database, you can use the migrate:refresh
Artisan command, which will roll back all migrations and then re-run them:
php artisan migrate:refresh
8. Seeding the Database:
Laravel also provides the ability to seed your database with initial data using seeders. You can create a seeder class using the make:seeder
Artisan command and define the data you want to insert into your tables.
9. Running Specific Migrations:
You can run a specific migration or a specific batch of migrations using the --path
and --step
options with the migrate
command. For example:
php artisan migrate --path=/database/migrations/my_migration.php
php artisan migrate --step=5
These are some of the fundamental concepts and commands related to Laravel migrations. Migrations are a powerful tool for managing your database schema and keeping it in sync with your application’s code as it evolves over time.