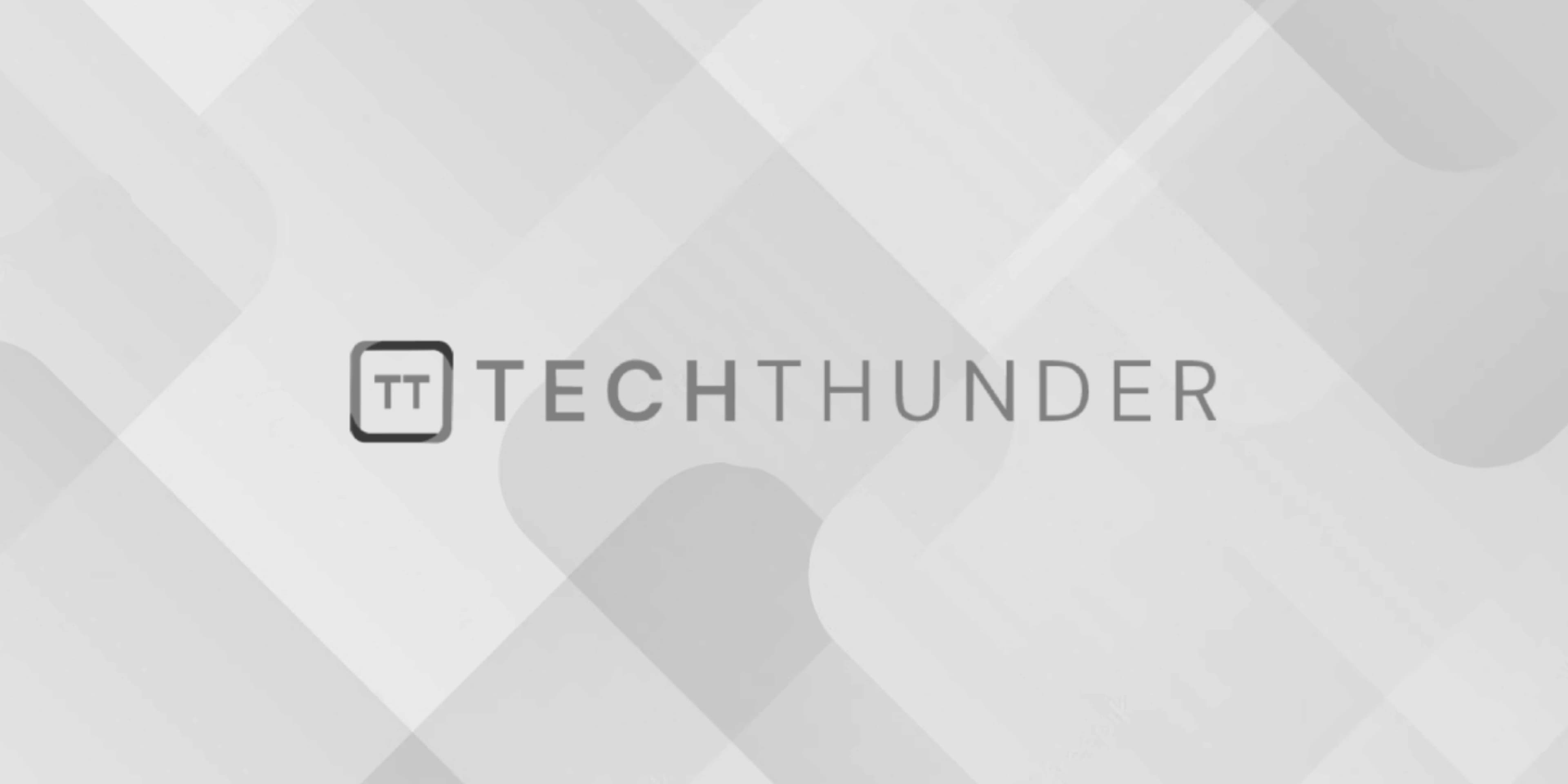
Laravel Google OAuth authentication using Socialite Package
Laravel’s Socialite package makes it easy to implement OAuth authentication with various social media platforms, including Google. Below is a step-by-step guide to integrating Google OAuth authentication using the Socialite package in a Laravel application:
- Install the Socialite Package: If you haven’t already, install the Socialite package using Composer:
composer require laravel/socialite
- Create Google OAuth App: Before you proceed, you’ll need to create a project in the Google Developers Console and set up OAuth credentials. Follow these steps:
- Go to the Google Developers Console.
- Create a new project and navigate to the “Credentials” section.
- Click on “Create Credentials” and choose “OAuth client ID”.
- Select “Web application” as the application type.
- Add the authorized redirect URI, which will be something like
http://your-app-url/login/google/callback
.
- Configure OAuth Credentials: In your
.env
file, add your Google OAuth credentials:
GOOGLE_CLIENT_ID=your-google-client-id
GOOGLE_CLIENT_SECRET=your-google-client-secret
GOOGLE_REDIRECT=http://your-app-url/login/google/callback
- Implement Google OAuth Login with Socialite: In your
config/services.php
file, add the Google configuration:
'google' => [
'client_id' => env('GOOGLE_CLIENT_ID'),
'client_secret' => env('GOOGLE_CLIENT_SECRET'),
'redirect' => env('GOOGLE_REDIRECT'),
],
- Routes and Controller: Create routes for the OAuth authentication and callback in
routes/web.php
:
Route::get('login/google', 'Auth\LoginController@redirectToGoogle');
Route::get('login/google/callback', 'Auth\LoginController@handleGoogleCallback');
Create a controller (if not already done) and add the necessary methods for Google OAuth authentication:
php artisan make:controller Auth\LoginController
// app/Http/Controllers/Auth/LoginController.php
use Laravel\Socialite\Facades\Socialite;
public function redirectToGoogle()
{
return Socialite::driver('google')->redirect();
}
public function handleGoogleCallback()
{
$user = Socialite::driver('google')->user();
// Perform user registration or login
// Example:
// $userModel = User::firstOrCreate([
// 'email' => $user->email
// ]);
// Auth::login($userModel);
return redirect()->route('home');
}
- Create Views and Links: Create views and links for logging in using Google. For example:
//resources/views/auth/login.blade.php
<a href="{{ url('login/google') }}">Login with Google</a>
- Testing: Start your Laravel development server:
php artisan serve
You should be able to visit the login page and see the “Login with Google” link. Clicking this link will redirect you to Google’s authentication page, and after granting access, you’ll be redirected back to your application.
Remember to adjust the routes, controllers, and views based on your application’s needs. This guide provides a basic setup for Google OAuth authentication using the Socialite package.