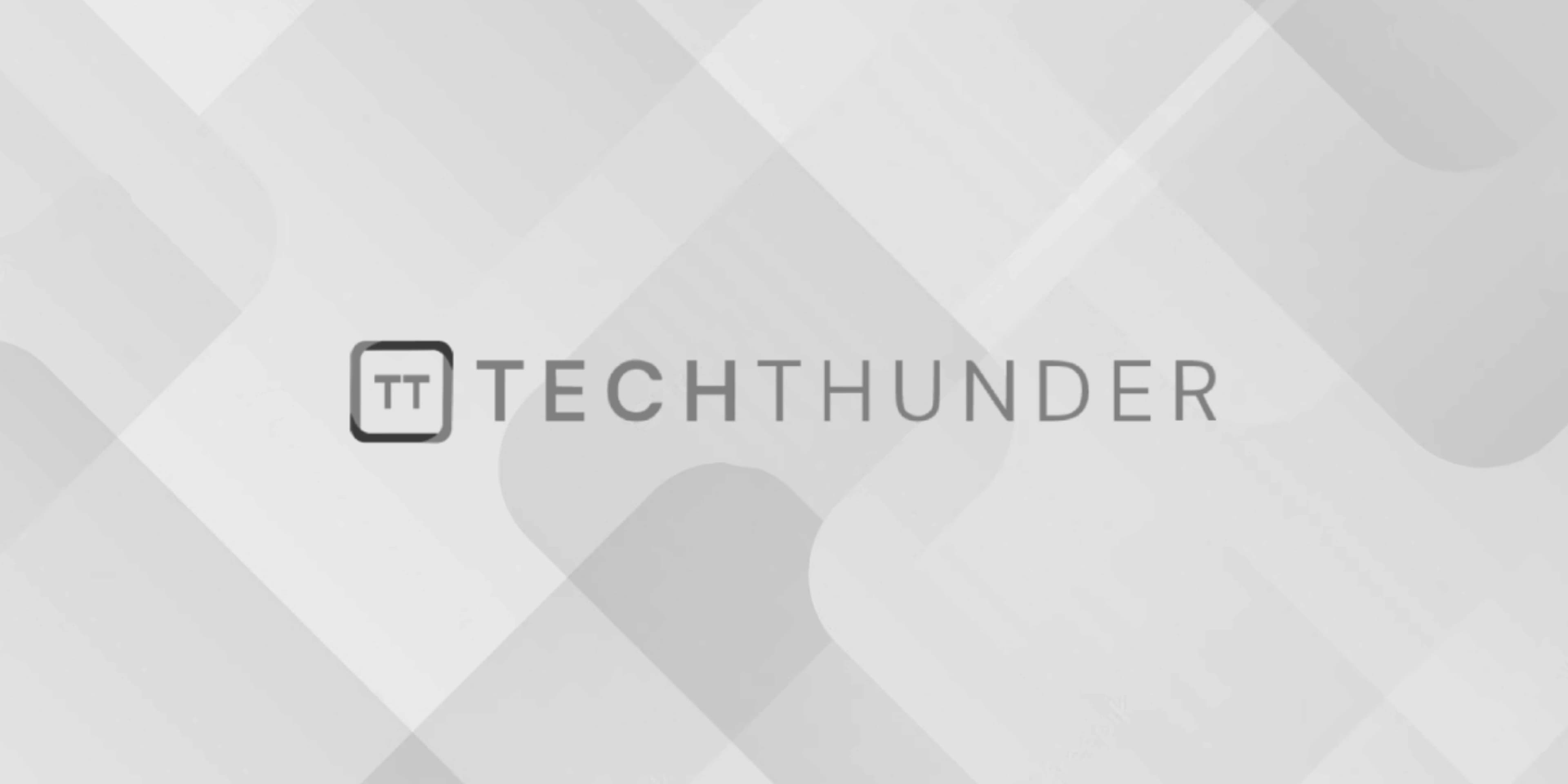
Laravel Route Groups
Laravel route groups allow you to group multiple routes together and apply common middleware, attributes, and other settings to all the routes within the group. This is useful for organizing routes and applying the same settings to a set of related routes. Route groups are defined using the Route::group
method in your routes/web.php
or routes/api.php
file.
Here’s how to use route groups in Laravel:
1. Basic Route Group:
You can define a basic route group with a closure function. For example, let’s create a group for authenticated users:
Route::middleware(['auth'])->group(function () {
// All routes inside this group require authentication
Route::get('/dashboard', 'DashboardController@index');
Route::get('/profile', 'ProfileController@index');
});
In this example, both /dashboard
and /profile
routes are inside the group and will require authentication.
2. Prefixing Route Group:
You can add a common prefix to all routes within the group using the prefix
method. For example:
Route::prefix('admin')->group(function () {
Route::get('/dashboard', 'AdminController@dashboard');
Route::get('/users', 'AdminController@users');
});
With this group, the routes will have the /admin
prefix, so /admin/dashboard
and /admin/users
will be the actual URLs.
3. Namespacing Controllers:
If you want to namespace controllers within a group, you can use the namespace
method:
Route::namespace('Admin')->group(function () {
Route::get('/dashboard', 'DashboardController@index');
Route::get('/users', 'UserController@index');
});
This is useful when you have controllers organized into different namespaces.
4. Middleware Groups:
You can apply a group of middleware to all routes within the group using the middleware
method. You can define middleware groups in your app/Http/Kernel.php
file and then apply them to the group:
Route::middleware(['web', 'auth', 'admin'])->group(function () {
// Routes requiring 'web', 'auth', and 'admin' middleware
});
5. Subdomains:
You can also group routes by subdomain using the domain
method:
Route::domain('api.example.com')->group(function () {
// Routes for the 'api.example.com' subdomain
});
6. Route Name Prefix:
You can add a common prefix to all route names within the group using the name
method:
Route::name('admin.')->group(function () {
Route::get('/dashboard', 'AdminController@dashboard')->name('dashboard');
Route::get('/users', 'AdminController@users')->name('users');
});
With this group, the route names will have the “admin.” prefix, like “admin.dashboard” and “admin.users.”
7. Route Attributes:
You can also apply common route attributes to the group using the attributes
method:
Route::attributes(['locale' => 'en'])->group(function () {
// Routes within this group will have the 'locale' attribute set to 'en'
});
Route groups are a powerful feature in Laravel for organizing and managing your routes. They help keep your code organized, reduce redundancy, and make it easier to apply middleware and attributes consistently to related routes.