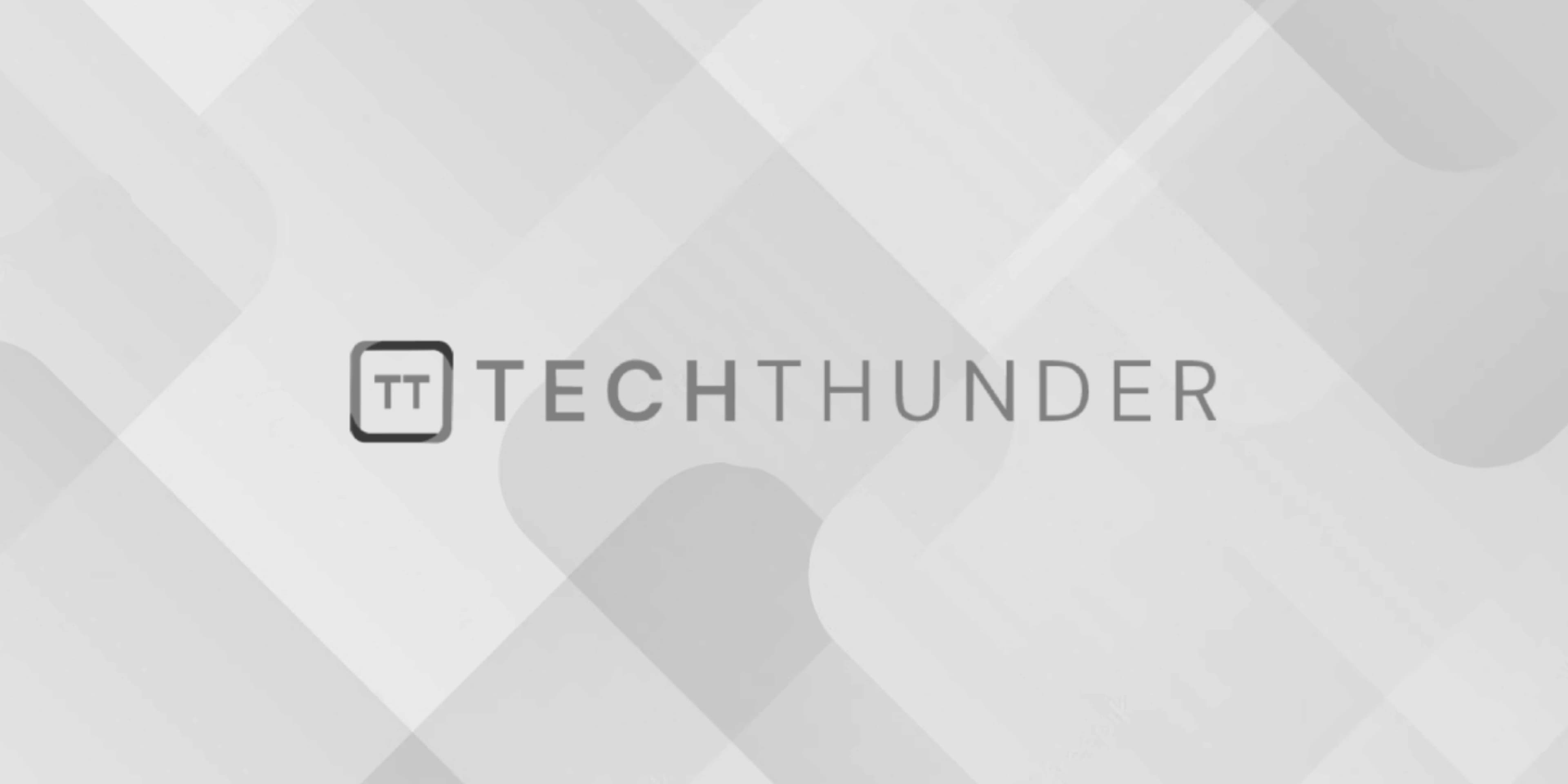
Migration Commands in Laravel
The laravel migration commands are used to create, manage, and execute database migrations. Migrations allow you to version and structure your database schema, making it easier to work collaboratively and keep your database schema in sync with your application’s code. Here are some commonly used migration commands in Laravel:
1. Create a New Migration:
To create a new migration, you can use the make:migration
Artisan command. This command generates a new migration file in the database/migrations
directory.
php artisan make:migration create_table_name
For example, to create a migration for a “posts” table:
php artisan make:migration create_posts_table
2. Running Migrations:
To execute pending migrations and apply the schema changes to the database, use the migrate
Artisan command:
php artisan migrate
This command will run all pending migrations in the order they were created.
3. Rolling Back Migrations:
If you need to undo the last batch of migrations, you can use the migrate:rollback
command:
php artisan migrate:rollback
To rollback a specific number of batches, use the --step
option:
php artisan migrate:rollback --step=2
4. Resetting and Refreshing the Database:
The migrate:reset
command will rollback all migrations:
php artisan migrate:reset
The migrate:refresh
command will rollback all migrations and then re-run them:
php artisan migrate:refresh
5. Creating a New Seeding Class:
To create a new seeder class for populating your database with initial data, use the make:seeder
command:
php artisan make:seeder UsersTableSeeder
6. Running Seeders:
To execute your seeders and populate the database with data, use the db:seed
Artisan command:
php artisan db:seed
You can also specify the seeder class to run:
php artisan db:seed --class=UsersTableSeeder
7. Rolling Back and Re-Running All Migrations and Seeders:
You can use the migrate:refresh
command along with the --seed
option to rollback all migrations and then re-run them, along with seeders:
php artisan migrate:refresh --seed
8. Viewing Migration Status:
To check the status of your migrations and see which migrations have been run and which are pending, use the migrate:status
command:
php artisan migrate:status
These are some of the commonly used migration commands in Laravel. Migrations provide a structured and version-controlled way to manage your database schema, making it easier to work collaboratively and keep your database schema in sync with your application’s code.