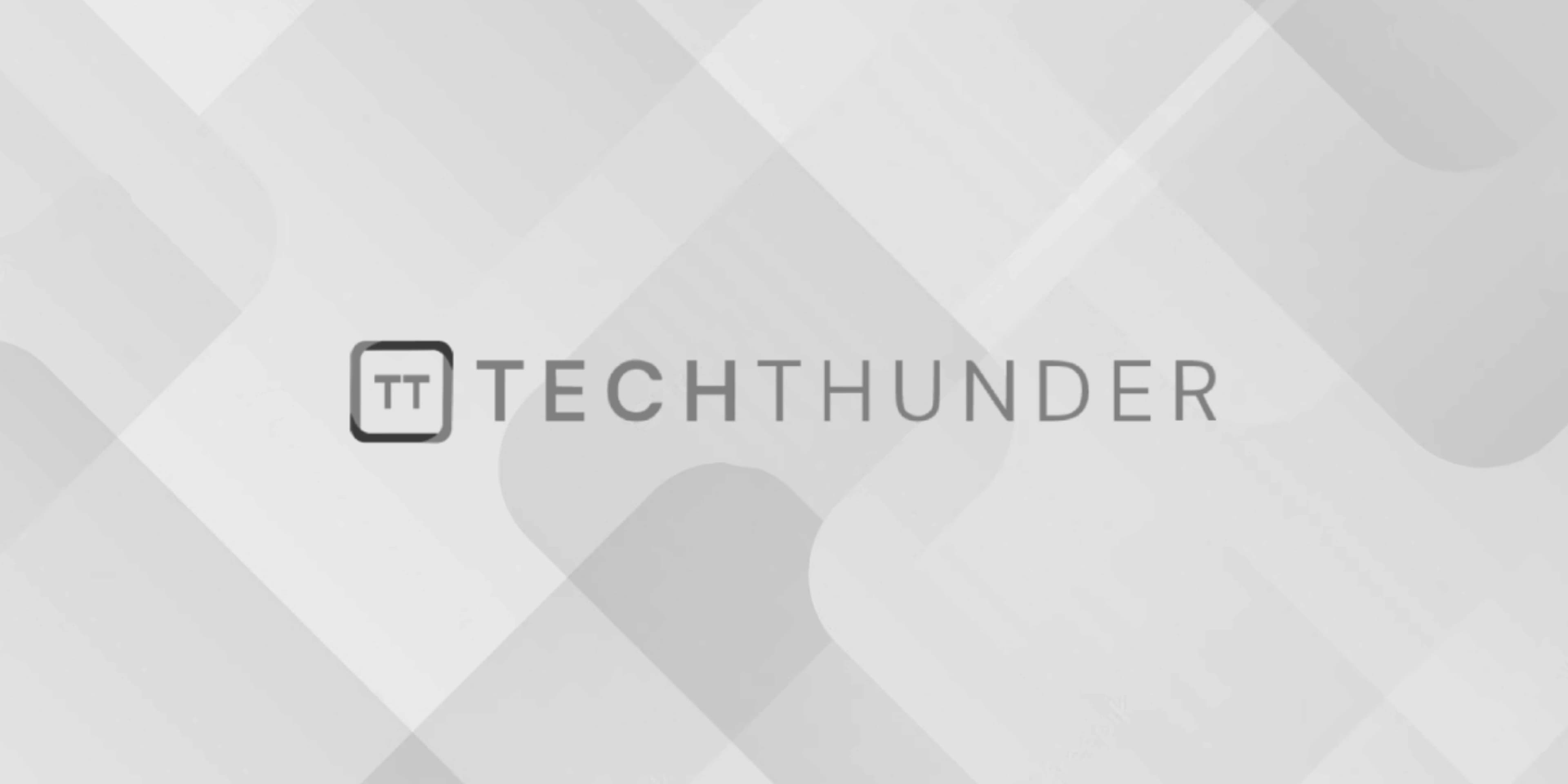
Laravel VueJS File Upload Using Vue-dropzone Example
The implement a Laravel Vue.js file upload using the vue2-dropzone
library, you can follow these steps. In this example, we will assume that you want to allow users to upload images.
Step 1: Install Laravel
If you haven’t already, create a Laravel project by running:
composer create-project --prefer-dist laravel/laravel laravel-vue-dropzone-example
Step 2: Install Dependencies
Next, install the necessary dependencies, including Vue.js and vue2-dropzone
:
composer require laravel/ui
php artisan ui vue
npm install
npm install vue2-dropzone --save
Step 3: Set Up Routes
In your routes/web.php
file, define a route that will return the view for your file upload component:
Route::get('/file-upload', 'FileUploadController@index');
Step 4: Create a Controller
Generate a controller for handling the file upload and view:
php artisan make:controller FileUploadController
In your FileUploadController.php
, add the following code to handle the view:
public function index()
{
return view('file-upload');
}
Step 5: Create a Blade View
Create a Blade view for the file upload form. Create a file named file-upload.blade.php
in the resources/views
directory:
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row">
<div class="col-md-8 col-md-offset-2">
<div class="panel panel-default">
<div class="panel-heading">File Upload</div>
<div class="panel-body">
<file-upload></file-upload>
</div>
</div>
</div>
</div>
</div>
@endsection
Step 6: Create a Vue Component
Create a Vue component for the file upload functionality. You can generate the component using Artisan:
php artisan make:component FileUpload
Edit the resources/js/components/FileUpload.vue
file with the following content:
<template>
<div>
<form @submit.prevent="uploadFile">
<vue-dropzone
ref="myDropzone"
id="file"
:options="dropzoneOptions"
@vdropzone-success="fileUploaded"
@vdropzone-error="fileUploadError"
></vue-dropzone>
<button type="submit">Upload File</button>
</form>
</div>
</template>
<script>
import 'dropzone/dist/dropzone.css';
import vue2Dropzone from 'vue2-dropzone';
export default {
components: {
vueDropzone: vue2Dropzone,
},
data() {
return {
dropzoneOptions: {
url: '/upload',
maxFilesize: 2, // Set your maximum file size here
acceptedFiles: 'image/*', // Specify accepted file types
dictDefaultMessage: 'Drop files here or click to upload',
headers: {
'X-CSRF-TOKEN': document.querySelector('meta[name="csrf-token"]').content,
},
},
};
},
methods: {
uploadFile() {
this.$refs.myDropzone.processQueue();
},
fileUploaded(file, response) {
// Handle successful file upload
console.log('File uploaded:', file, 'Response:', response);
},
fileUploadError(file, errorMessage) {
// Handle file upload error
console.error('File upload error:', file, 'Error message:', errorMessage);
},
},
};
</script>
Step 7: Set Up Routes for File Upload
In your routes/web.php
file, define a route for handling the file upload:
Route::post('/upload', 'FileUploadController@upload');
Step 8: Create a Controller Method for File Upload
In your FileUploadController.php
, add the following code to handle the file upload:
use Illuminate\Http\Request;
public function upload(Request $request)
{
$uploadedFile = $request->file('file');
// Perform any necessary validation here
$filename = $uploadedFile->store('uploads', 'public');
return response()->json(['message' => 'File uploaded successfully', 'filename' => $filename]);
}
Make sure you have a public
disk configured in your config/filesystems.php
file.
Step 9: Set Up CSRF Token in Your Layout
Make sure to include the CSRF token in the <head>
section of your layout file (resources/views/layouts/app.blade.php
):
<meta name="csrf-token" content="{{ csrf_token() }}">
Step 10: Include the Vue Component
In your layout file (resources/views/layouts/app.blade.php
), include the Vue component:
<script src="{{ mix('js/app.js') }}" defer></script>
Step 11: Compile Assets
Compile your assets to make the Vue component available:
npm run dev
Step 12: Start the Development Server
Start the Laravel development server:
php artisan serve
Now, you should be able to visit /file-upload
in your browser and see the file upload form with the vue2-dropzone
component. Users can drag and drop files or click to select files for upload.
This example demonstrates a basic setup for Laravel file uploads with Vue.js using vue2-dropzone
. You can further customize the behavior and styling as needed for your project.