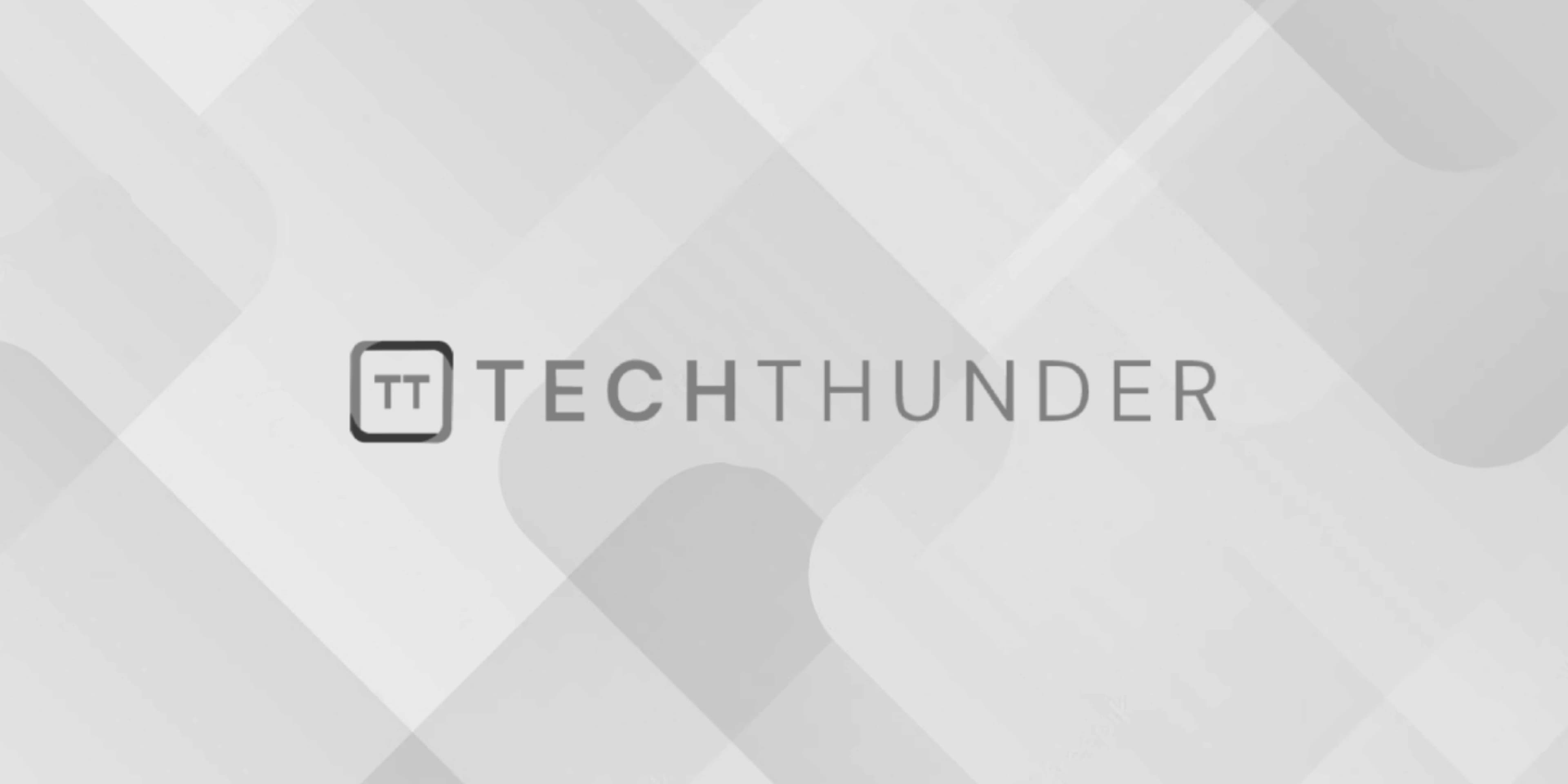
Laravel Tinker
Laravel Tinker is an interactive REPL (Read-Eval-Print Loop) console that allows you to interact with your Laravel application from the command line. It provides an interactive environment where you can run PHP code and Laravel-specific commands to inspect and manipulate your application’s data and components. Here’s how to use Laravel Tinker:
1. Accessing Tinker:
You can access Laravel Tinker by running the following command in your terminal:
php artisan tinker
This command opens the Tinker shell, and you can start interacting with your Laravel application from there.
2. Running PHP Code:
Inside the Tinker shell, you can run regular PHP code and Laravel-specific code. For example, you can perform calculations or create variables:
$sum = 5 + 3;
3. Accessing Models and Eloquent:
One of the most common uses of Tinker is to interact with your Eloquent models. You can retrieve, create, update, and delete records from your database using Eloquent syntax:
// Retrieve all records from a model
$users = App\User::all();
// Create a new record
$user = new App\User;
$user->name = 'John Doe';
$user->email = '[email protected]';
$user->password = bcrypt('password');
$user->save();
// Update a record
$user = App\User::find(1);
$user->name = 'Updated Name';
$user->save();
// Delete a record
$user = App\User::find(1);
$user->delete();
4. Querying the Database:
You can also run raw database queries using Tinker:
DB::table('users')->where('name', 'John')->first();
5. Accessing Configuration:
You can access your Laravel application’s configuration values using Tinker:
config('app.name');
6. Accessing Services and Dependencies:
You can access and interact with Laravel’s services and dependencies:
// Access the cache service
Cache::put('key', 'value', 60);
// Access the mail service
Mail::to('[email protected]')->send(new App\Mail\WelcomeMail());
// Access the event dispatcher
event(new App\Events\UserRegistered($user));
7. Exiting Tinker:
To exit the Tinker shell, simply type exit
or press Ctrl+D in your terminal.
8. Custom Tinker Commands:
You can create custom Tinker commands to perform specific tasks in your application. To do this, define your custom commands in the AppServiceProvider.php
file.
9. Caution with Tinker:
Be cautious when using Tinker in a production environment. It provides powerful access to your application’s internals, and running incorrect commands can lead to data loss or unintended consequences.
Laravel Tinker is a valuable tool for debugging, testing, and exploring your application. It’s especially useful for quickly testing code snippets and checking the state of your application during development. However, always be mindful of the environment in which you’re using Tinker to avoid any unexpected issues.