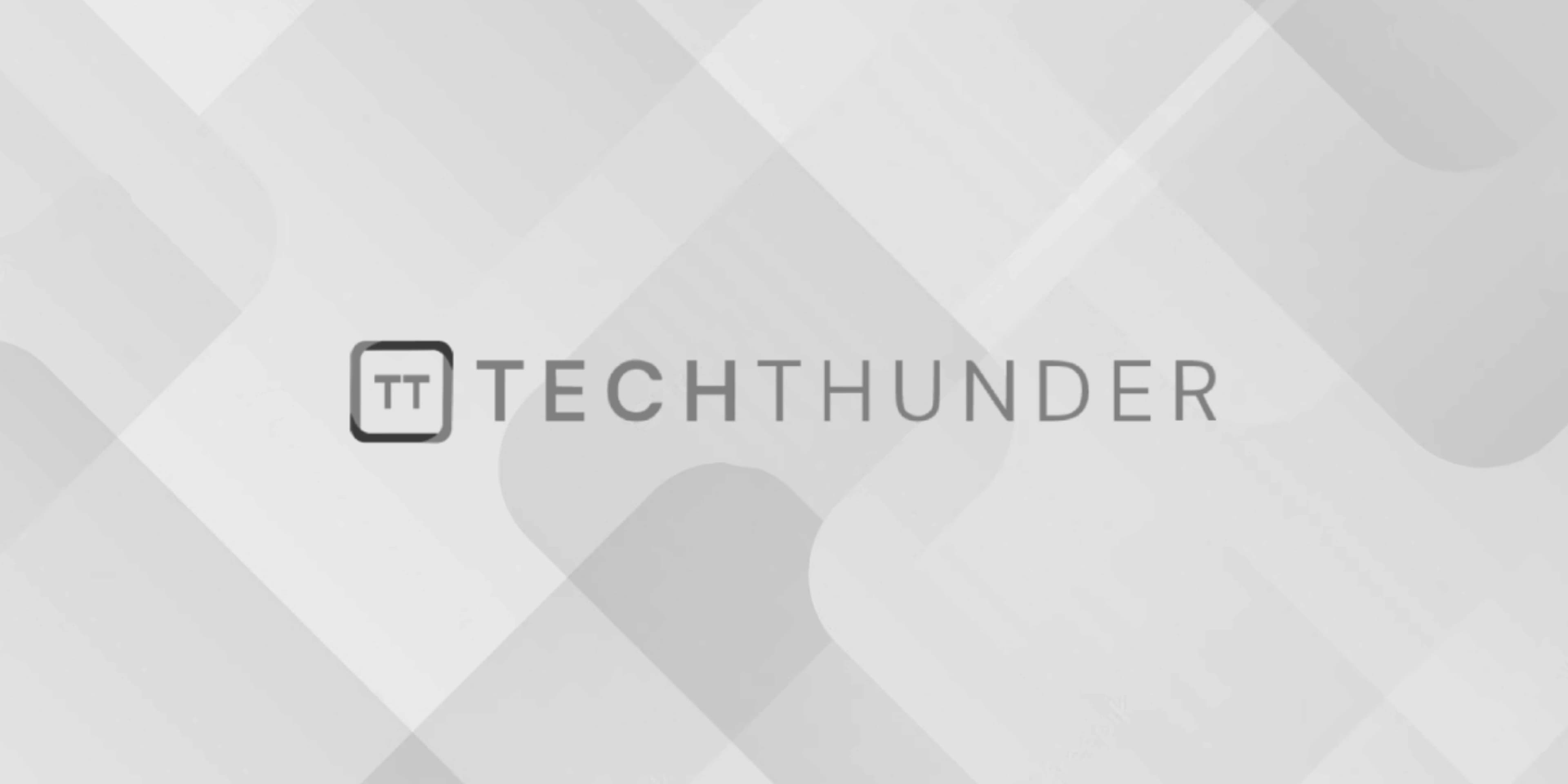
Laravel Resource Controllers
Laravel resource controllers provide a convenient way to handle common CRUD (Create, Read, Update, Delete) operations for a resource in your application. A resource, in Laravel terms, typically corresponds to a database table and its associated model. Resource controllers help you define standard routes and controller methods for performing these operations.
Here are the steps to create and use a resource controller in Laravel:
1. Generate a Resource Controller:
To create a resource controller, you can use the make:controller
Artisan command with the --resource
flag. For example, to create a resource controller for managing “posts,” you would run:
php artisan make:controller PostController --resource
This will generate a PostController
with predefined CRUD methods.
2. Define Routes:
Laravel provides a convenient way to define routes for resource controllers using the Route::resource
method. In your routes/web.php
file, you can define the routes for your “posts” resource like this:
Route::resource('posts', 'PostController');
This single line of code defines multiple routes for CRUD operations, such as creating, reading, updating, and deleting posts.
3. Controller Methods:
The generated PostController
will include methods for handling CRUD operations, as well as some other common actions. Here’s a list of the methods and their corresponding HTTP actions:
index
: Handles theGET /posts
route (Listing all posts).create
: Handles theGET /posts/create
route (Displaying the form for creating a new post).store
: Handles thePOST /posts
route (Storing a new post in the database).show
: Handles theGET /posts/{id}
route (Displaying a single post).edit
: Handles theGET /posts/{id}/edit
route (Displaying the form for editing a post).update
: Handles thePUT/PATCH /posts/{id}
route (Updating a post in the database).destroy
: Handles theDELETE /posts/{id}
route (Deleting a post from the database).
You can customize these methods in the PostController
according to your application’s needs.
4. Views:
By default, Laravel assumes that the views for your resource are located in a folder with the same name as the resource (e.g., resources/views/posts
). You can create Blade views for each of your controller actions within this folder.
5. Form Requests (Optional):
You can also use Laravel form request validation to validate incoming data before storing or updating a resource. These form request classes can be generated using the make:request
Artisan command and then applied to your controller methods.
6. Middleware (Optional):
You can add middleware to your controller methods to control access and perform actions like authentication and authorization.
7. Customizing Routes and Controller Methods (Optional):
If you need to customize your routes or controller methods, you can manually define routes in your routes/web.php
file and create corresponding methods in your controller.
Resource controllers in Laravel are a powerful way to quickly scaffold common CRUD operations for your application. They help maintain consistency in your routes and make it easier to manage resources. However, you can always customize and extend them to fit your specific application requirements.