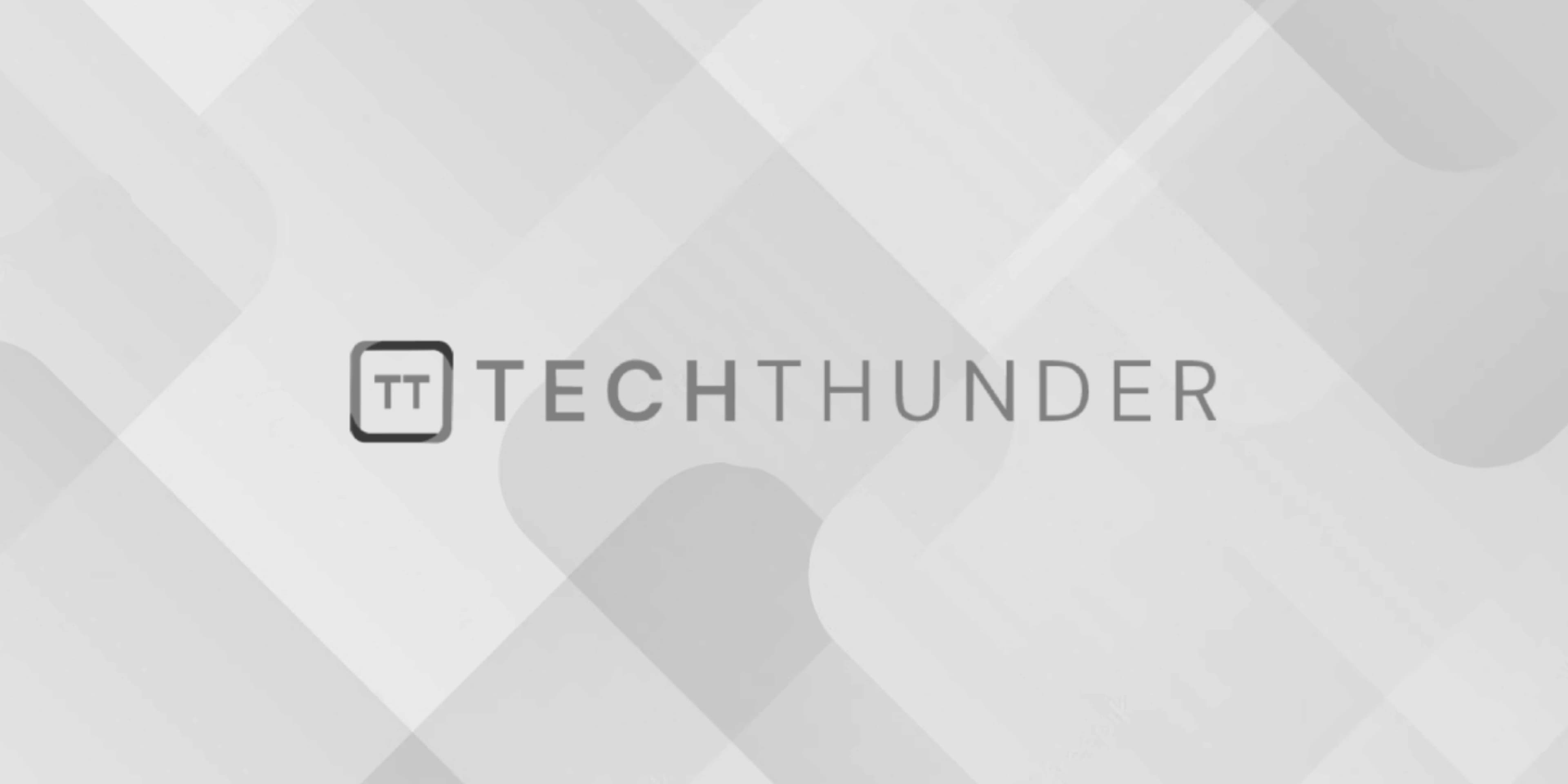
Use Elasticsearch from scratch in Laravel 5
To use Elasticsearch from scratch in Laravel 5, you’ll need to follow several steps to set up Elasticsearch and integrate it into your Laravel application. Here’s a step-by-step guide:
Step 1: Install Elasticsearch
Before you can use Elasticsearch, you need to install and set it up on your server. You can download Elasticsearch from the official website: https://www.elastic.co/downloads/elasticsearch
Once you’ve installed Elasticsearch, make sure it’s running on your server.
Step 2: Install Elasticsearch PHP Client
To interact with Elasticsearch from Laravel, you’ll need the Elasticsearch PHP client. Install it using Composer:
composer require elasticsearch/elasticsearch
Step 3: Create a Laravel Project
If you haven’t already, create a new Laravel project:
composer create-project laravel/laravel laravel-elasticsearch
Step 4: Configure Elasticsearch Connection
In your Laravel project, open the config/database.php
file. You can create a new Elasticsearch connection by adding the following configuration in the connections
array:
'elasticsearch' => [
'driver' => 'elasticsearch',
'hosts' => [
[
'host' => env('ELASTICSEARCH_HOST', 'localhost'),
'port' => env('ELASTICSEARCH_PORT', 9200),
'scheme' => env('ELASTICSEARCH_SCHEME', 'http'),
],
],
'log_enabled' => true, // Set this to true for logging Elasticsearch queries
],
Step 5: Set Environment Variables
In your .env
file, add the following environment variables for Elasticsearch:
ELASTICSEARCH_HOST=localhost
ELASTICSEARCH_PORT=9200
ELASTICSEARCH_SCHEME=http
You can adjust these values to match your Elasticsearch configuration.
Step 6: Create an Elasticsearch Service Provider
Generate a custom service provider for Elasticsearch:
php artisan make:provider ElasticsearchServiceProvider
In the generated ElasticsearchServiceProvider.php
file, register the Elasticsearch client in the register
method:
use Elasticsearch\Client;
use Elasticsearch\ClientBuilder;
public function register()
{
$this->app->bind(Client::class, function () {
return ClientBuilder::create()
->setHosts(config('database.connections.elasticsearch.hosts'))
->setLogger(app('log'))
->build();
});
}
Step 7: Create an Elasticsearch Index
Create an Elasticsearch index for your data. For example, if you want to search for products, you can create a migration and a model for products:
php artisan make:migration create_products_index
php artisan make:model Product
In the migration file, define the structure of your Elasticsearch index:
use Elasticsearch\Client;
public function up()
{
$client = app(Client::class);
$params = [
'index' => 'products',
'body' => [
'mappings' => [
'properties' => [
'id' => ['type' => 'integer'],
'name' => ['type' => 'text'],
'description' => ['type' => 'text'],
// Add more fields as needed
],
],
],
];
$client->indices()->create($params);
}
public function down()
{
$client = app(Client::class);
$client->indices()->delete(['index' => 'products']);
}
Run the migration to create the Elasticsearch index:
php artisan migrate
Step 8: Use Elasticsearch in Your Application
You can now use Elasticsearch to index and search data in your Laravel application. For example, to index a product:
use Elasticsearch\Client;
public function indexProduct(Product $product)
{
$client = app(Client::class);
$params = [
'index' => 'products',
'id' => $product->id,
'body' => $product->toArray(),
];
$client->index($params);
}
To search for products:
use Elasticsearch\Client;
public function searchProducts($query)
{
$client = app(Client::class);
$params = [
'index' => 'products',
'body' => [
'query' => [
'match' => ['name' => $query],
],
],
];
$response = $client->search($params);
// Process the search results
return $response;
}
These are basic examples, and you can customize your Elasticsearch queries and indexing logic as needed for your application.
Remember to handle exceptions and errors properly and consider adding validation and user authentication to your application to ensure data security and integrity.
That’s it! You’ve set up Elasticsearch in a Laravel 5 application from scratch and can now use it to index and search data.